How does React handle the key prop when components are re-ordered in a list
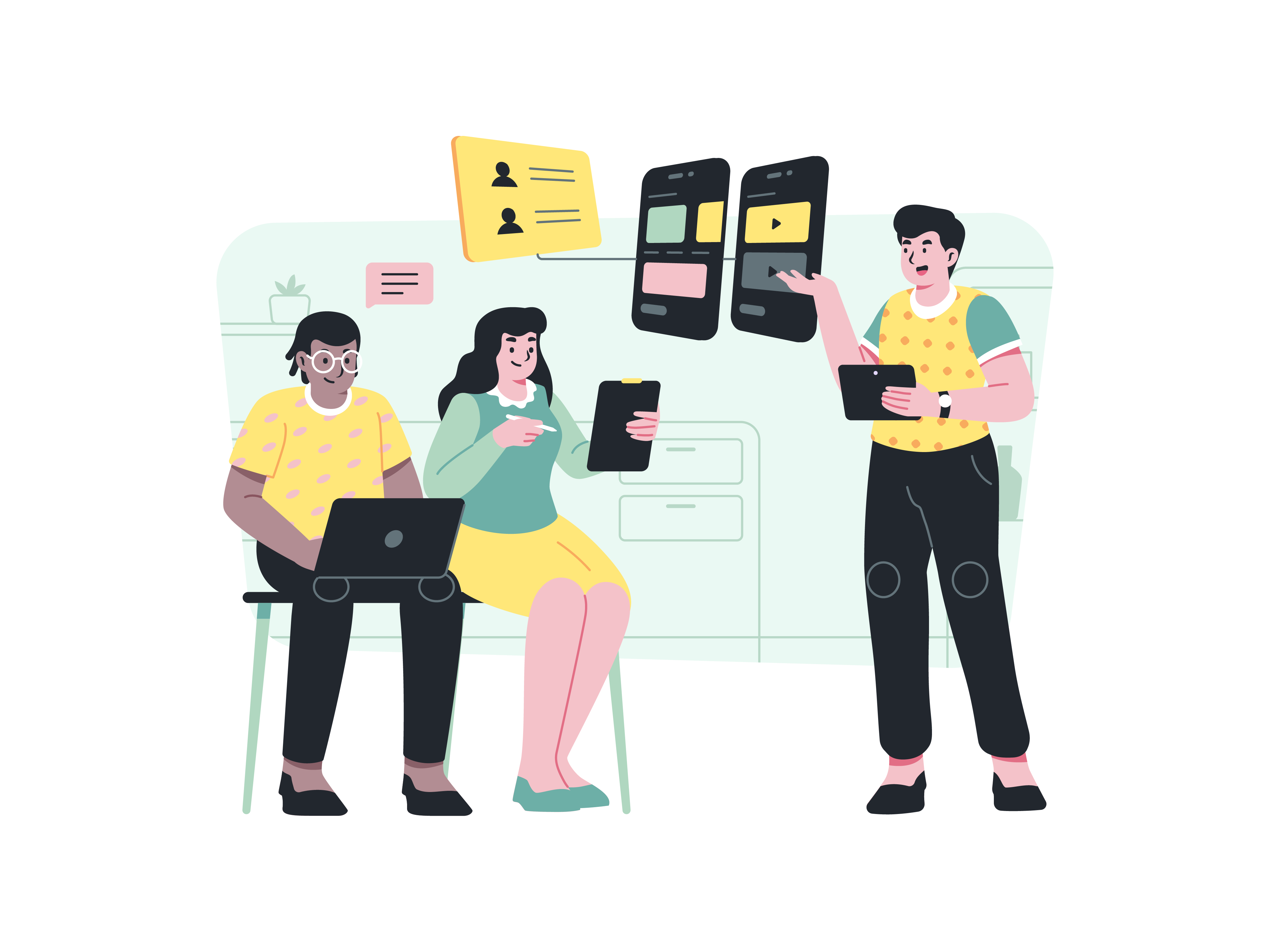
In React, the key
prop is a special attribute that helps the library identify individual components within a list. It is essential for React’s reconciliation algorithm, which determines the most efficient way to update the DOM when the state or props of a component change.
When rendering a list of components, React uses the key
prop to uniquely identify each item. This identification is critical for React to understand which components need to be added, removed, or re-ordered when the list undergoes changes.
React’s Reconciliation Algorithm
React’s reconciliation algorithm, often referred to as the Virtual DOM diffing algorithm, is at the heart of efficient updates. When a component’s state or props change, React generates a new virtual DOM representation. It then compares this new representation with the previous one to identify the minimal set of changes required to update the actual DOM.
The key
prop plays a crucial role in this process. By associating a unique key with each component, React can efficiently determine which components have been added, removed, or re-ordered in the list. This ensures that updates are applied optimally, minimizing unnecessary DOM manipulations.
Importance of Keys in Component Re-ordering
Consider a scenario where you have a list of components rendered in a certain order. If the components are re-ordered without using keys, React might struggle to differentiate between them. Without keys, React relies on the order of elements to identify components, which can lead to suboptimal performance and unexpected behavior.
// Without keys
const ComponentList = () => {
return (
<ul>
<li>Component A</li>
<li>Component B</li>
<li>Component C</li>
</ul>
);
};
Now, let’s explore how adding keys to the components helps React maintain a clear understanding of the component identity:
// With keys
const ComponentList = () => {
return (
<ul>
<li key="a">Component A</li>
<li key="b">Component B</li>
<li key="c">Component C</li>
</ul>
);
};
With keys in place, React can accurately track the identity of each component. When re-ordering occurs, React recognizes the changes and efficiently updates the DOM accordingly.
Handling Re-ordering Scenarios
React’s reconciliation algorithm excels in handling various scenarios involving component re-ordering. Let’s explore these scenarios and understand how React ensures optimal performance:
1. Adding Components
When new components are added to the list, React identifies them by their unique keys. It efficiently adds these components to the DOM without re-rendering the entire list, resulting in a seamless user experience.
2. Removing Components
If components are removed from the list, React uses the key
prop to pinpoint the components to be removed. This targeted removal minimizes the impact on performance compared to re-rendering the entire list.
3. Re-ordering Components
When components are re-ordered, React leverages the keys to understand the changes in the list order. It then applies the necessary updates without unnecessary re-renders, ensuring an efficient and visually consistent result.
Common Pitfalls and Best Practices
While the key
prop is a powerful tool, it’s crucial to use it correctly to avoid potential pitfalls. Here are some best practices and considerations:
a. Ensure Uniqueness
Each key should be unique within its scope. Using non-unique keys can lead to unpredictable behavior and negatively impact performance.
b. Stable Identifiers
Avoid using volatile values like indexes as keys, especially when dealing with dynamic lists. If the list can change, indexes may not provide stable identifiers, leading to unintended consequences.
// Avoid using indexes as keys
{items.map((item, index) => (
<Component key={index} />
))}
c. Dynamic Key Generation
If the list items don’t have a natural unique identifier, consider generating dynamic keys based on item properties. This ensures stability and uniqueness.
// Use dynamic keys based on item properties
{items.map((item) => (
<Component key={item.id} />
))}
Conclusion
In conclusion, the key
prop is a vital element in React’s toolkit for efficiently handling component re-ordering in lists. By providing a stable and unique identifier for each component, developers enable React’s reconciliation algorithm to make informed decisions about updates, additions, and removals.
Understanding the inner workings of React’s reconciliation process and adopting best practices for key usage empowers developers to create performant and responsive applications. With a clear grasp of how React handles the key
prop, developers can leverage this mechanism to build robust and efficient user interfaces in their React applications.
How to create a responsive contact form with Bootstrap
How to use Bootstrap’s utilities for hiding and showing elements
How to implement a sticky footer with a content area that scrolls
How to use Bootstrap’s form control sizing classes
What are the utility classes for responsive design in Tailwind CSS