What is Redux, and how does it integrate with React
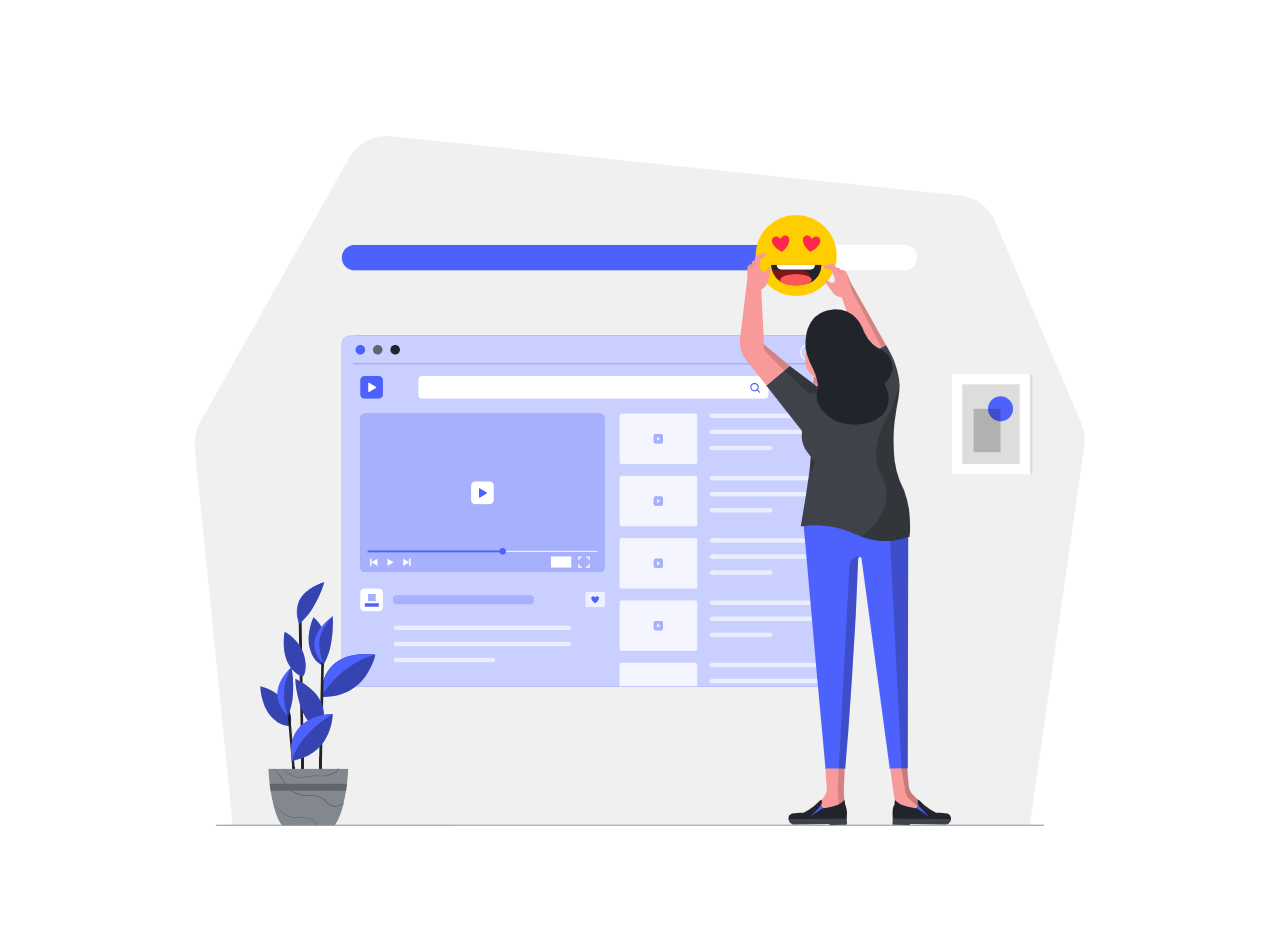
Redux, a predictable state container for JavaScript applications, has become a staple in the React ecosystem for managing state in a scalable and maintainable way. In this comprehensive guide, we’ll delve into what Redux is, its core principles, and explore how it seamlessly integrates with React to provide a robust state management solution for building complex and dynamic user interfaces.
What is Redux
The Core Principles
Redux is built on three fundamental principles: a single source of truth, state is read-only, and changes are made through pure functions. These principles help maintain a predictable state and make it easier to understand and debug the application.
- Single Source of Truth In a Redux application, the entire state of the application is stored in a single JavaScript object, commonly referred to as the “store.” This ensures a single source of truth, making it straightforward to track and manage the application’s state.
- State is Read-Only State in Redux is immutable, meaning it cannot be directly modified. Instead, any changes to the state must be expressed as plain objects known as “actions.” These actions describe what happened in the application and are the only way to modify the state.
- Changes are Made Through Pure Functions To specify how the state is transformed by actions, Redux relies on pure functions called “reducers.” Reducers take the current state and an action as input and return a new state. Because reducers are pure functions, they produce the same output for the same input, making them predictable and easy to test.
Key Concepts
-
Actions Actions are plain JavaScript objects that describe changes in the application. They must have a
type
property indicating the type of action and can include additional data as needed.const incrementCounter = { type: 'INCREMENT_COUNTER', payload: 1, };
-
Reducers Reducers are functions responsible for specifying how the state changes in response to actions. They take the current state and an action as parameters and return a new state.
const counterReducer = (state = 0, action) => { switch (action.type) { case 'INCREMENT_COUNTER': return state + action.payload; default: return state; } };
-
Store The store is an object that holds the application’s state. It is created by passing the root reducer to the
createStore
function from the Redux library.import { createStore } from 'redux'; const store = createStore(counterReducer);
-
Dispatch Actions are dispatched to the store using the
dispatch
method. This is how components signal that something has happened in the application.store.dispatch(incrementCounter);
-
Subscribe Components can subscribe to the store to be notified of changes to the state. This allows them to update their UI in response to state changes.
const unsubscribe = store.subscribe(() => { console.log('Current state:', store.getState()); });
Integrating Redux with React
1. Setting Up Redux
To integrate Redux with a React application, the first step is to install the required packages:
npm install redux react-redux
The redux
package provides the core functionality of Redux, while react-redux
provides bindings for React.
2. Creating the Store
The next step is to create the Redux store. This involves defining reducers and combining them into a root reducer using the combineReducers
utility.
// reducers.js
const counterReducer = (state = 0, action) => {
// Reducer logic
};
const rootReducer = combineReducers({
counter: counterReducer,
// Add other reducers as needed
});
export default rootReducer;
// index.js
import { createStore } from 'redux';
import { Provider } from 'react-redux';
import rootReducer from './reducers';
const store = createStore(rootReducer);
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root')
);
3. Connecting Components
The connect
function from react-redux
is used to connect React components to the Redux store. This allows components to access the state and dispatch actions.
// CounterComponent.js
import React from 'react';
import { connect } from 'react-redux';
const CounterComponent = ({ counter, incrementCounter }) => {
return (
<div>
<p>Counter: {counter}</p>
<button onClick={incrementCounter}>Increment</button>
</div>
);
};
const mapStateToProps = (state) => ({
counter: state.counter,
});
const mapDispatchToProps = (dispatch) => ({
incrementCounter: () => dispatch({ type: 'INCREMENT_COUNTER' }),
});
export default connect(mapStateToProps, mapDispatchToProps)(CounterComponent);
4. Accessing State in Components
The connect
function injects the specified parts of the Redux state as props into the connected component. In the example above, counter
is injected into the CounterComponent
as a prop.
5. Dispatching Actions
Similarly, actions can be dispatched by calling the functions provided in mapDispatchToProps
. In this case, incrementCounter
is a prop that, when called, dispatches the INCREMENT_COUNTER
action.
Best Practices and Considerations
- Use Redux Wisely Redux is a powerful tool, but it may not be necessary for every application. Consider the complexity and state management needs of your application before introducing Redux.
- Organize Actions and Reducers As the application grows, organize actions and reducers into separate files based on functionality. This improves maintainability and makes it easier to reason about the state changes.
- Middleware for Side Effects
For handling asynchronous operations or side effects, Redux middleware like
redux-thunk
orredux-saga
can be employed. These provide a clean way to manage asynchronous logic within the Redux architecture. - Immutability for Predictability: Embrace immutability when updating state in reducers. This ensures that the state changes are predictable and makes debugging easier.
- DevTools for Debugging Utilize browser extensions like Redux DevTools to inspect and debug the state changes in your application. This powerful tool provides insights into the state at each step and aids in identifying issues.
Conclusion
Redux, with its principles of a single source of truth, immutable state, and predictable state changes, has proven to be a valuable tool for state management in React applications. By seamlessly integrating with React components, Redux provides a scalable and maintainable solution for handling complex state logic.
Understanding the core concepts of Redux, such as actions, reducers, and the store, is essential for effective integration with React. By following best practices, developers can harness the full power of Redux to build robust and scalable applications that effectively manage state and provide a seamless user experience.
How do you manage state in a Redux store
How does React handle memoization
How do you handle forms in React with controlled and uncontrolled components
What is the purpose of the useMemo hook in React
How can you optimize performance in React applications