How to create a responsive contact form with validation in Bootstrap
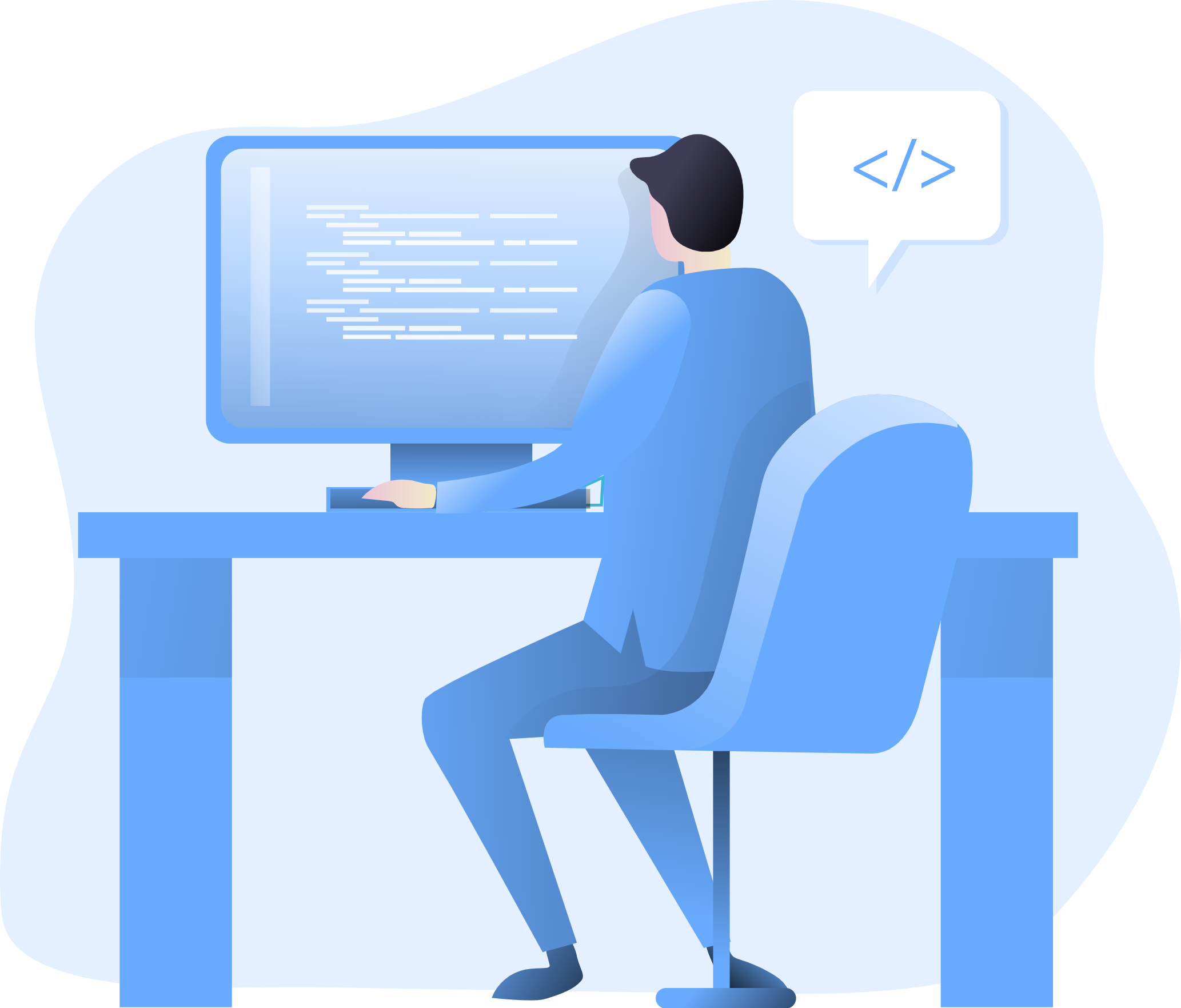
In the realm of web development, creating a responsive and user-friendly contact form is a crucial aspect of engaging with your audience effectively. Bootstrap, a powerful front-end framework, provides a seamless solution for crafting aesthetically pleasing and functional forms. In this article, we’ll explore the step-by-step process of creating a responsive contact form with validation using Bootstrap, ensuring a smooth and error-free user experience.
Setting Up Your Project
Before delving into the implementation, make sure you have Bootstrap integrated into your project. You can either download the Bootstrap files and include them locally or use a Content Delivery Network (CDN). Here’s a basic HTML template with Bootstrap included:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link href="path/to/bootstrap.min.css" rel="stylesheet">
<title>Responsive Contact Form with Bootstrap</title>
</head>
<body>
<!-- Your content goes here -->
<script src="path/to/bootstrap.bundle.min.js"></script>
</body>
</html>
Replace “path/to/bootstrap.min.css” and “path/to/bootstrap.bundle.min.js” with the correct file paths or URLs.
Creating the Contact Form
Bootstrap provides a variety of form components and styles that make it easy to build responsive and visually appealing forms. Let’s create a basic contact form structure:
<div class="container">
<form id="contactForm" class="needs-validation" novalidate>
<div class="mb-3">
<label for="name" class="form-label">Name</label>
<input type="text" class="form-control" id="name" name="name" required>
<div class="invalid-feedback">
Please enter your name.
</div>
</div>
<div class="mb-3">
<label for="email" class="form-label">Email</label>
<input type="email" class="form-control" id="email" name="email" required>
<div class="invalid-feedback">
Please enter a valid email address.
</div>
</div>
<div class="mb-3">
<label for="message" class="form-label">Message</label>
<textarea class="form-control" id="message" name="message" rows="5" required></textarea>
<div class="invalid-feedback">
Please enter a message.
</div>
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
</div>
In this example, we’ve used Bootstrap’s form components such as form-control
for inputs and btn
for the submit button. Each input field has the required
attribute for basic form validation.
Adding Validation Feedback
Bootstrap provides styles and classes for displaying validation feedback to users. We’ve already included the invalid-feedback
class for each input field. This class will be used to display error messages when the user submits the form with incomplete or incorrect information.
Implementing JavaScript for Advanced Validation
While basic HTML validation is useful, incorporating JavaScript can enhance the validation process. Bootstrap’s form validation classes and events make it easy to implement custom validation logic. Add the following script after including the Bootstrap JavaScript file:
<script>
// JavaScript for form validation
(function () {
'use strict';
// Fetch all the forms we want to apply custom Bootstrap validation styles to
var forms = document.querySelectorAll('.needs-validation');
// Loop over them and prevent submission
Array.from(forms).forEach(function (form) {
form.addEventListener('submit', function (event) {
if (!form.checkValidity()) {
event.preventDefault();
event.stopPropagation();
}
form.classList.add('was-validated');
}, false);
});
})();
</script>
This script adds a custom event listener to each form with the class needs-validation
. It prevents the form from being submitted if it fails basic HTML validation and adds the class was-validated
to apply Bootstrap’s custom validation styles.
Styling for Responsiveness
Bootstrap ensures that your contact form looks great on devices of all sizes by using its grid system and responsive utility classes. However, you can further customize the styling based on your design preferences. Here’s an example:
<style>
/* Additional custom styling */
body {
background-color: #f8f9fa;
}
.container {
background-color: #ffffff;
margin-top: 50px;
padding: 30px;
border-radius: 8px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
</style>
This CSS snippet adds a subtle box shadow and background color to the form container for a clean and modern look.
Conclusion
Creating a responsive contact form with validation using Bootstrap is a straightforward process that significantly enhances user interaction on your website. By leveraging Bootstrap’s form components and validation styles, you ensure a seamless and visually pleasing experience for users on various devices. Customize the form based on your specific needs, and consider adding additional styling to match your overall design aesthetic. With the power of Bootstrap, crafting an effective and visually appealing contact form has never been more accessible.
How to create a responsive timeline with vertical lines in Bootstrap
How to use Bootstrap’s card group for organizing content
How to implement a collapsible sidebar menu with Bootstrap
How to use Bootstrap’s text truncation classes for long content
How to implement a parallax scrolling effect with Bootstrap