How to implement a collapsible sidebar menu with Bootstrap
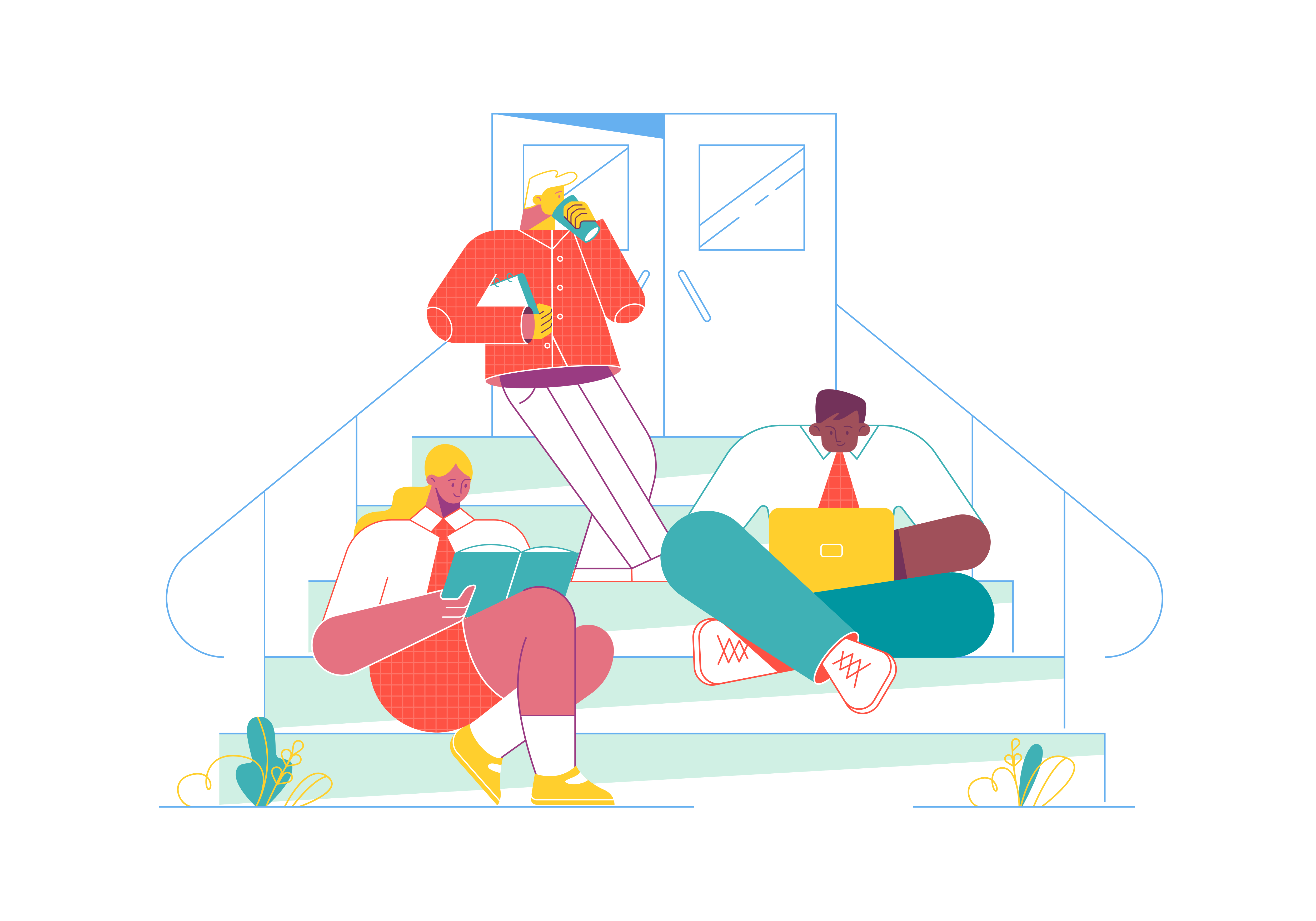
In the realm of web development, creating a user-friendly and responsive navigation system is paramount. One popular solution for achieving this is Bootstrap, a front-end framework that simplifies the process of building stylish and functional interfaces. In this article, we will delve into the steps of implementing a collapsible sidebar menu with Bootstrap, exploring the intricacies of structure, styling, and interactivity.
Understanding the Basics
Before we dive into the implementation, let’s briefly understand what a collapsible sidebar menu is and why it’s a valuable addition to your web design toolkit.
A collapsible sidebar menu is a navigation element that starts in a fully expanded state and can be toggled to collapse or expand, providing a space-saving approach to navigation on websites and web applications. This design is particularly useful for projects with a significant amount of content, as it allows users to focus on the main content area while providing easy access to navigation when needed.
Setting Up Your Project
To get started, make sure you have Bootstrap integrated into your project. You can either download the Bootstrap files and include them in your project or use a CDN. Here’s a basic HTML template with Bootstrap included:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link href="path/to/bootstrap.min.css" rel="stylesheet">
<title>Collapsible Sidebar Menu with Bootstrap</title>
</head>
<body>
<!-- Your content goes here -->
<script src="path/to/bootstrap.bundle.min.js"></script>
</body>
</html>
Replace “path/to/bootstrap.min.css” and “path/to/bootstrap.bundle.min.js” with the correct file paths or URLs.
Creating the HTML Structure
The foundation of our collapsible sidebar menu lies in the HTML structure. Bootstrap utilizes a combination of container-fluid
, row
, and col
classes to create a flexible and responsive layout. Here’s a basic example:
<div class="container-fluid">
<div class="row">
<nav class="col-md-2 d-none d-md-block bg-light sidebar">
<!-- Sidebar content goes here -->
</nav>
<main role="main" class="col-md-9 ml-sm-auto col-lg-10 px-4">
<!-- Main content goes here -->
</main>
</div>
</div>
In this structure, the nav
element represents our sidebar, and the main
element represents the main content area. The d-none d-md-block
classes ensure that the sidebar is hidden on smaller screens.
Adding Content to the Sidebar
Now, let’s populate the sidebar with some content. Bootstrap offers a variety of components that can be used in the sidebar, such as navigation links and headings. Here’s an example:
<nav class="col-md-2 d-none d-md-block bg-light sidebar">
<div class="sidebar-sticky">
<ul class="nav flex-column">
<li class="nav-item">
<a class="nav-link active" href="#">
<span data-feather="home"></span>
Home
</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">
<span data-feather="file"></span>
Page 1
</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">
<span data-feather="shopping-cart"></span>
Page 2
</a>
</li>
</ul>
</div>
</nav>
Here, we’ve used Bootstrap’s nav
and nav-link
classes to create a list of navigation links. The flex-column
class ensures that the links are displayed in a vertical layout.
Adding a Toggle Button
To make our sidebar collapsible, we need to add a toggle button. Bootstrap provides a navbar-toggler
class that we can use for this purpose. Here’s how you can integrate it into your sidebar:
<nav class="col-md-2 d-none d-md-block bg-light sidebar">
<div class="sidebar-sticky">
<!-- Toggle button -->
<button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#sidebarToggle" aria-controls="sidebarToggle" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<!-- Sidebar content -->
<div class="collapse" id="sidebarToggle">
<ul class="nav flex-column">
<!-- Navigation links go here -->
</ul>
</div>
</div>
</nav>
In this example, the navbar-toggler
button is linked to a div
with the id sidebarToggle
. This div
contains the collapsible content, which is hidden by default (collapse
class). The button’s attributes (data-toggle
, data-target
, etc.) are crucial for the functionality of the toggle.
Styling the Sidebar
While Bootstrap provides a clean and functional design out of the box, you might want to customize the appearance to match your project’s aesthetics. You can add your styles or use Bootstrap utility classes to adjust colors, fonts, and spacing. Here’s a basic example:
<nav class="col-md-2 d-none d-md-block bg-dark sidebar">
<div class="sidebar-sticky">
<button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#sidebarToggle" aria-controls="sidebarToggle" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse" id="sidebarToggle">
<ul class="nav flex-column">
<li class="nav-item">
<a class="nav-link active" href="#">
<span data-feather="home"></span>
Home
</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">
<span data-feather="file"></span>
Page 1
</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">
<span data-feather="shopping-cart"></span>
Page 2
</a>
</li>
</ul>
</div>
</div>
</nav>
In this adjusted example, the sidebar has a dark background (bg-dark
) for a more modern look.
Adding JavaScript for Interactivity
The final step is to add the necessary JavaScript to enable the sidebar’s collapsing functionality. Ensure that Bootstrap’s JavaScript file is included in your project, as mentioned in the initial setup. The following script initializes the Bootstrap components, including the sidebar toggle:
<script src="path/to/bootstrap.bundle.min.js"></script>
Replace “path/to/bootstrap.bundle.min.js” with the correct file path or URL.
Conclusion
Implementing a collapsible sidebar menu with Bootstrap can significantly enhance the navigation experience on your website or web application. By following these steps, you can create a responsive and visually appealing sidebar that adapts seamlessly to various screen sizes.
How to use Bootstrap’s responsive flexbox utilities
How to create a responsive timeline with vertical lines in Bootstrap
How to use Bootstrap’s card group for organizing content
How to use Bootstrap’s table-responsive class