How to use Bootstrap's text truncation classes for long content
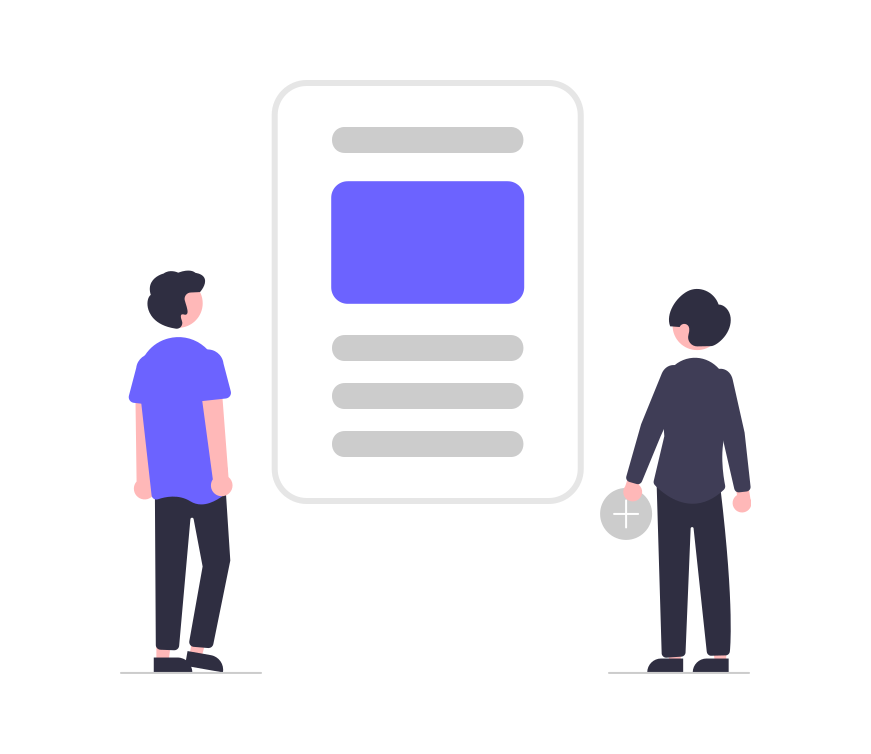
In the ever-expanding world of web development, managing content length and ensuring a polished user interface is crucial. Bootstrap, a popular front-end framework, offers a solution to tackle lengthy text content elegantly – the text truncation classes. In this article, we will explore the intricacies of Bootstrap’s text truncation classes, providing you with the knowledge to maintain a clean and organized layout while handling copious amounts of textual information.
Understanding Text Truncation
Text truncation involves shortening lengthy text content to fit within a specified space while maintaining readability. This technique is particularly beneficial in scenarios where space is limited, such as in cards, tables, or other components.
Bootstrap simplifies the process of text truncation by providing utility classes that seamlessly handle overflow and present a concise representation of content without compromising user experience.
Setting Up Your Project
Before diving into the implementation, ensure that you have Bootstrap integrated into your project. You can either download the Bootstrap files and include them locally or utilize a Content Delivery Network (CDN). Here’s a basic HTML template with Bootstrap included:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link href="path/to/bootstrap.min.css" rel="stylesheet">
<title>Bootstrap Text Truncation Guide</title>
</head>
<body>
<!-- Your content goes here -->
<script src="path/to/bootstrap.bundle.min.js"></script>
</body>
</html>
Replace “path/to/bootstrap.min.css” and “path/to/bootstrap.bundle.min.js” with the appropriate file paths or URLs.
Using Text Truncation Classes
Bootstrap provides a set of classes to handle text truncation elegantly. Let’s explore these classes and how to implement them.
1. text-truncate
The text-truncate
class truncates text with an ellipsis (…) to indicate that there is more content than what is currently visible. Apply this class to any element containing text that you want to truncate:
<p class="text-truncate">
Long text that needs to be truncated goes here.
</p>
2. overflow-hidden
The overflow-hidden
class hides any content that overflows the container. Combining this class with text-truncate
ensures a neat and tidy appearance:
<div class="overflow-hidden">
<p class="text-truncate">
Long text that needs to be truncated goes here.
</p>
</div>
3. d-inline-block
The d-inline-block
class makes an element an inline block, allowing it to be used in contexts where inline elements are expected. This is particularly useful for truncating text within inline elements:
<span class="d-inline-block text-truncate">
Long text that needs to be truncated goes here.
</span>
4. mw-100
The mw-100
class sets the maximum width of an element to 100%, ensuring that it doesn’t overflow its container. Combine it with text-truncate
for effective text truncation:
<div class="mw-100">
<p class="text-truncate">
Long text that needs to be truncated goes here.
</p>
</div>
5. text-break
The text-break
class allows text to break onto a new line, preventing it from overflowing its container. Use it in conjunction with text-truncate
for a comprehensive approach:
<p class="text-break text-truncate">
Long text that needs to be truncated goes here.
</p>
Customizing Text Truncation
Bootstrap’s text truncation classes are versatile and can be customized further to suit your design preferences. You can combine these classes, experiment with different container elements, and adjust styles using additional CSS if needed.
<div class="custom-container mw-100">
<p class="custom-text text-truncate">
Long text that needs to be truncated goes here.
</p>
</div>
In this example, a custom container with the mw-100
class is created to set the maximum width. The custom-text
class is used to apply additional styling if required.
Responsive Design
Bootstrap’s text truncation classes seamlessly adapt to different screen sizes, making your content look polished on both large screens and mobile devices. Ensure that you include the Bootstrap viewport meta tag in the <head>
section of your HTML:
<meta name="viewport" content="width=device-width, initial-scale=1.0">
This meta tag ensures proper scaling on various devices.
Conclusion
Mastering text truncation with Bootstrap’s utility classes empowers you to present lengthy content in a visually appealing and organized manner. Whether you’re working with paragraphs, headings, or inline elements, Bootstrap’s classes provide a straightforward solution to handle text overflow gracefully. Experiment with different combinations, customize styles, and leverage the responsiveness of Bootstrap to create a seamless user experience for your website or web application. With the power of text truncation at your fingertips, you can strike the perfect balance between information and aesthetics.
How to create a responsive form layout with Bootstrap
How to implement a parallax scrolling effect with Bootstrap
How to use Bootstrap’s list group as a menu
How to create a responsive pricing table with Bootstrap
How to use Bootstrap’s responsive flexbox utilities
How to create a responsive timeline with vertical lines in Bootstrap