What are hooks rules in React
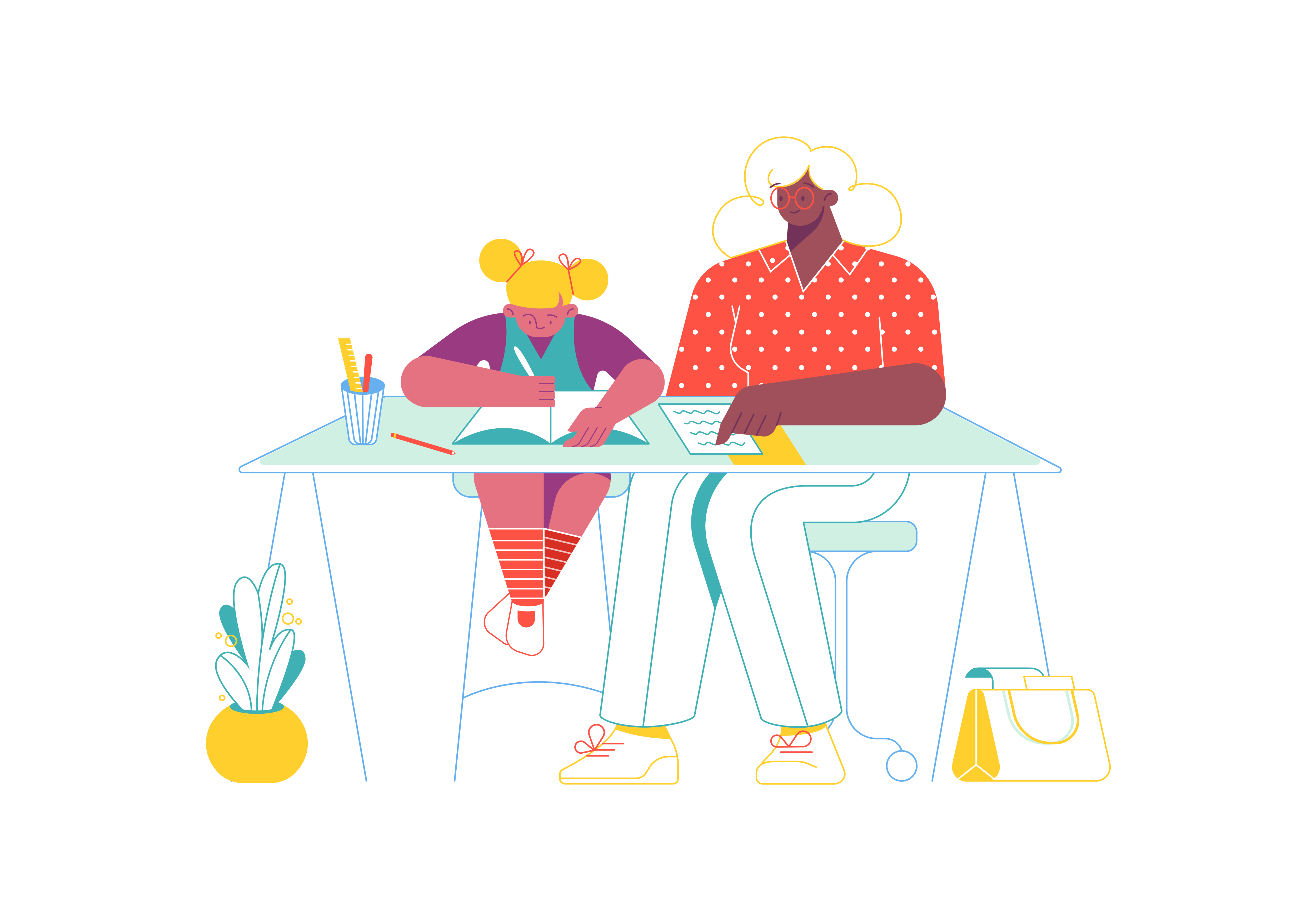
React Hooks, introduced in React 16.8, revolutionized state management and side effect handling in functional components. However, as powerful as they are, React Hooks come with specific rules and best practices to ensure consistent and reliable behavior in React applications. In this comprehensive guide, we’ll explore the rules governing the use of React Hooks, understand their significance, and delve into best practices for effective and error-free development.
-
The Basics of React Hooks
-
Introduction to Hooks: React Hooks provide a way to use state and lifecycle features in functional components, previously exclusive to class components. They include useState, useEffect, useContext, and more, allowing developers to encapsulate and reuse component logic.
-
Commonly Used Hooks:
useState
: Manages state in functional components.useEffect
: Handles side effects in functional components.useContext
: Accesses context values within functional components.useReducer
: A more advanced state management hook.useCallback
anduseMemo
: Optimize performance by memoizing functions and values.
-
-
Rules for Using React Hooks
-
Only Call Hooks at the Top Level: Hooks should be called at the top level of functional components and not inside loops, conditions, or nested functions. This ensures that Hooks are called consistently, allowing React to maintain the order and association between Hooks and components.
// Good function MyComponent() { const [count, setCount] = useState(0); useEffect(() => { // Effect logic }, [count]); // Component logic }
-
Call Hooks Only from React Functions: Hooks should only be called from within React functional components or custom hooks. Avoid calling them from regular JavaScript functions, as this could lead to unexpected behavior.
-
Do Not Call Hooks Conditionally: Hooks should not be called conditionally based on some logical expressions. Ensure that Hooks are called in the same order on every render to maintain consistent state and effects.
// Avoid function MyComponent() { if (condition) { const [value, setValue] = useState(initialValue); } }
-
Rules for Custom Hooks: Custom hooks should start with the word “use” to indicate that they follow the rules of Hooks. This naming convention is crucial for distinguishing between regular functions and custom hooks.
// Good function useCustomHook() { // Custom hook logic }
-
-
Best Practices for Using React Hooks
-
Separate Concerns with Multiple Hooks: Break down the logic of a component into multiple smaller hooks to separate concerns and enhance reusability. This also makes it easier to test and reason about individual pieces of functionality.
function MyComponent() { const userData = useUserData(); const theme = useTheme(); const analytics = useAnalytics(); // Component logic using userData, theme, and analytics }
-
Use the Dependency Array in useEffect Wisely: Be mindful of the dependency array in useEffect. Include only the variables that are directly used inside the effect. Avoid unnecessary re-renders and side effects by carefully specifying dependencies.
useEffect(() => { // Effect logic using someVariable }, [someVariable]);
-
Leverage useReducer for Complex State Logic: For components with complex state logic that involves multiple sub-states or transitions, consider using
useReducer
instead of multipleuseState
calls.useReducer
can enhance code readability and maintainability.const initialState = { count: 0 }; function reducer(state, action) { switch (action.type) { case 'increment': return { count: state.count + 1 }; // Other cases default: throw new Error(); } } function MyComponent() { const [state, dispatch] = useReducer(reducer, initialState); // Component logic using state and dispatch }
-
Optimize Performance with useCallback and useMemo: Utilize
useCallback
to memoize functions anduseMemo
to memoize values, preventing unnecessary re-creations of these entities on each render. This is particularly useful in performance-critical scenarios.const memoizedCallback = useCallback(() => { // Memoized callback logic }, [dependency]); const memoizedValue = useMemo(() => computeExpensiveValue(a, b), [a, b]);
-
-
Common Pitfalls and Solutions
-
State Updates May Be Asynchronous: Understand that state updates with
useState
may be asynchronous, especially when updating based on the previous state. Use the functional form ofsetState
to ensure correct updates.// Incorrect setCount(count + 1); // Correct setCount((prevCount) => prevCount + 1);
-
Avoiding Stale Closures in useEffect: Be cautious when using variables from the component scope inside
useEffect
, as closures may lead to stale values. Include those variables in the dependency array if needed.useEffect(() => { // Effect logic using someVariable }, [someVariable]);
-
Understanding Dependency Array in useEffect: Carefully manage the dependency array in
useEffect
to avoid missing updates or causing unnecessary re-renders. Consider using eslint-plugin-react-hooks to enforce rules related to dependency arrays.
-
-
Conclusion
React Hooks have transformed the way developers manage state and side effects in functional components. Adhering to the rules and best practices ensures a clean and reliable codebase, making it easier to scale and maintain React applications. By mastering the intricacies of React Hooks, developers can harness their full potential for creating efficient, modular, and maintainable code in the ever-evolving landscape of web development.
How do I handle dark mode in Tailwind CSS
How can I add a new font to my Tailwind CSS project
What is the purpose of the “purge” option in the Tailwind CSS configuration
What are the utility classes for responsive design in Tailwind CSS
How do I create a fixed navbar using Tailwind CSS