How does React handle code splitting with React.lazy, Suspense, and webpack 5
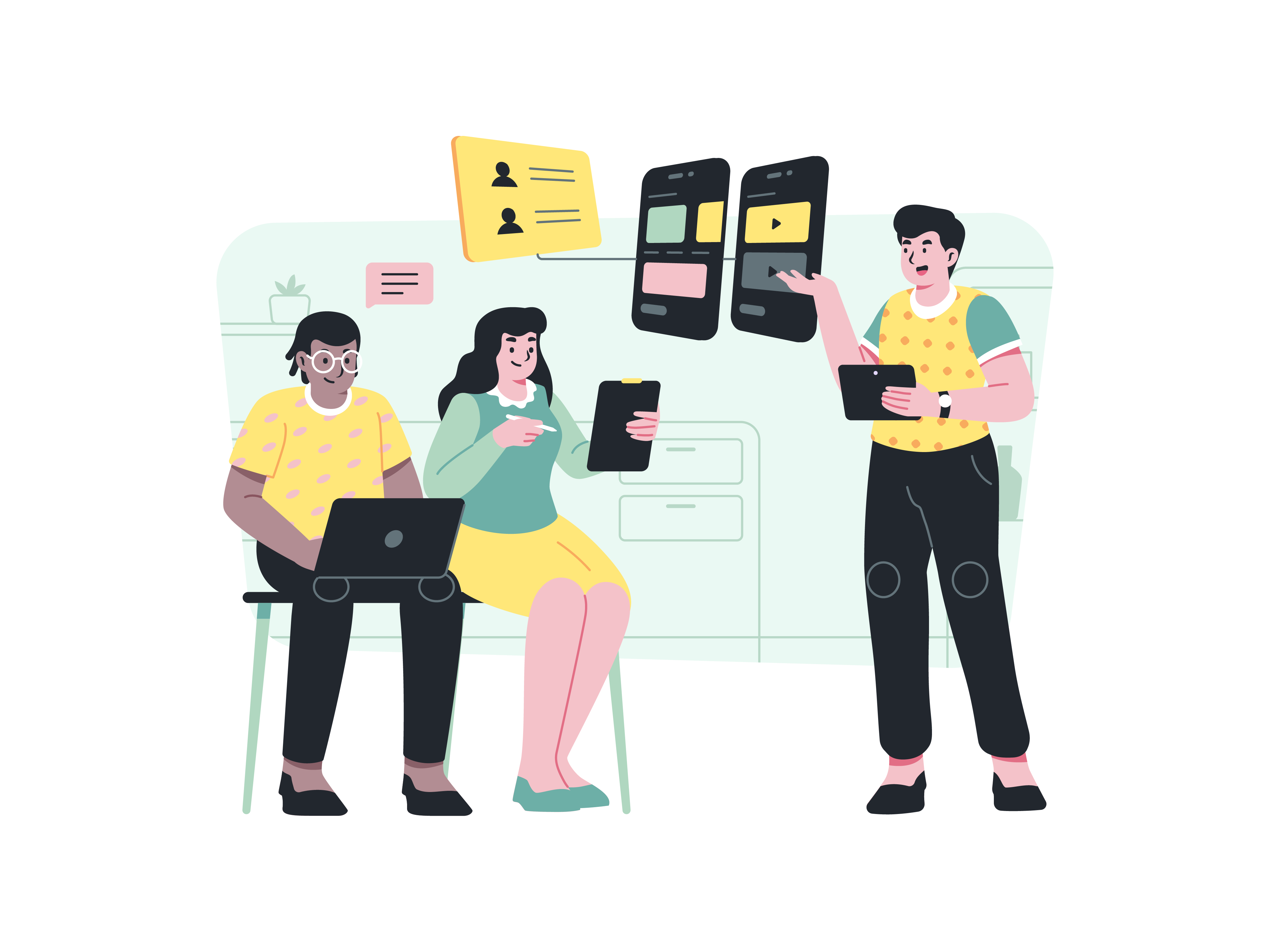
Code splitting is a powerful optimization technique that allows React developers to break down their application’s bundle into smaller, more manageable chunks. With the introduction of React.lazy, Suspense, and the advancements in Webpack 5, code splitting in React has become more accessible and efficient. In this comprehensive guide, we will delve into how React handles code splitting using these features, exploring their roles, usage, and the synergy between them for creating highly performant web applications.
-
Understanding the Need for Code Splitting
- Overview of Bundling in React: In traditional React applications, the entire JavaScript bundle is loaded upfront, even if the user only needs a specific section of the application. This can result in slower initial load times and affect overall performance.
- Benefits of Code Splitting: Code splitting addresses this issue by dynamically loading only the necessary JavaScript code for a specific route or component. This leads to faster initial load times, improved user experience, and optimized resource utilization.
-
React.lazy and Dynamic Imports
-
Introduction to React.lazy: React.lazy is a feature introduced in React 16.6 that enables developers to load components lazily, or on-demand, through dynamic imports. This feature simplifies the implementation of code splitting, allowing for more granular control over when and how components are loaded.
-
Syntax of React.lazy: The syntax of React.lazy involves using dynamic import to lazily load a component. The lazy-loaded component is then wrapped with React.lazy, creating a new component that can be rendered in the application.
const MyLazyComponent = React.lazy(() => import('./MyComponent'));
-
Usage in React Components: React.lazy is typically used in scenarios where a component is not needed immediately but should be loaded when a certain condition is met, such as when navigating to a specific route.
const MyLazyComponent = React.lazy(() => import('./MyComponent')); const MyParentComponent = () => ( <React.Suspense fallback={<LoadingSpinner />}> <MyLazyComponent /> </React.Suspense> );
-
-
React Suspense for Component-Level Loading
-
Introduction to React Suspense: React Suspense is a complementary feature to React.lazy that allows components to suspend rendering while waiting for something, such as a lazy-loaded component, to resolve.
-
Error Boundaries and Fallback UI: Suspense is used in conjunction with error boundaries to gracefully handle loading states and potential errors. The
fallback
prop provides a UI to display while the suspended component is being loaded.const MyLazyComponent = React.lazy(() => import('./MyComponent')); const MyParentComponent = () => ( <React.Suspense fallback={<LoadingSpinner />}> <MyLazyComponent /> </React.Suspense> );
-
Error Boundaries for Error Handling: React error boundaries, implemented using
try...catch
statements or thecomponentDidCatch
lifecycle method, are crucial for catching errors during the rendering of suspended components.
-
-
Webpack 5 and Module Federation
- Overview of Webpack 5: Webpack 5, the latest version of the popular JavaScript module bundler, introduces improvements in performance, tree shaking, and support for the new Module Federation feature.
- Tree Shaking for Dead Code Elimination: Webpack 5 enhances tree shaking, a process that eliminates dead code from the bundle, ensuring that only the necessary code is included. This is particularly beneficial for optimizing the size of code-split chunks.
- Module Federation for Micro Frontends: Module Federation is a groundbreaking feature in Webpack 5 that allows the creation of micro frontends – independent, loosely coupled applications that can be composed together. This aligns well with the principles of code splitting, enabling the creation of scalable and modular React applications.
-
Optimizing Code Splitting in React Applications
-
Granular Code Splitting: By strategically applying React.lazy to specific components or routes, developers can achieve granular code splitting, ensuring that only the essential parts of the application are loaded on demand.
-
Route-Based Code Splitting: Code splitting can be applied on a per-route basis, allowing different sections of a React application to be loaded dynamically. This is particularly useful for applications with complex navigation structures.
const HomePage = React.lazy(() => import('./HomePage')); const AboutPage = React.lazy(() => import('./AboutPage')); const App = () => ( <Router> <Suspense fallback={<LoadingSpinner />}> <Route path="/home" component={HomePage} /> <Route path="/about" component={AboutPage} /> </Suspense> </Router> );
-
Conditional Code Splitting: React.lazy can be used conditionally, allowing developers to decide at runtime whether a component should be loaded lazily based on certain conditions or user interactions.
const MyComponent = condition ? React.lazy(() => import('./MyComponent')) : null; const MyParentComponent = () => ( <Suspense fallback={<LoadingSpinner />}> {MyComponent && <MyComponent />} </Suspense> );
-
-
Best Practices for Code Splitting in React
-
Profile and Analyze Bundle Size: Regularly profile and analyze your application’s bundle size using tools like Webpack Bundle Analyzer to identify opportunities for further optimization.
-
Use Webpack’s
magicComments
for Naming Chunks: Webpack allows developers to name chunks using magic comments, providing more readable and predictable chunk names. This is especially useful when debugging or analyzing network requests.const MyLazyComponent = React.lazy(() => import(/* webpackChunkName: "my-chunk" */ './MyComponent'));
-
Consider Server-Side Rendering (SSR) Compatibility: If your React application uses server-side rendering, ensure that code splitting and lazy loading are compatible with the SSR setup to avoid issues with initial renders.
-
Regularly Update Dependencies: Keep your dependencies, including React, React.lazy, and Webpack, up to date to benefit from performance improvements and new features introduced in each release.
-
Test for Accessibility: When implementing code splitting, test for accessibility to ensure that users with assistive technologies have a smooth and accessible experience, even during dynamic loading.
-
-
Conclusion
Code splitting with React.lazy, Suspense, and Webpack 5 is a powerful combination that empowers developers to create highly performant and scalable applications. By understanding the synergy between React’s lazy loading capabilities and Webpack’s optimization features, developers can optimize bundle sizes, reduce initial load times, and enhance the user experience. Incorporating best practices ensures a clean and maintainable codebase, setting the stage for efficient development in the evolving landscape of React and modern web applications.
How do you handle code splitting with React Router
What is the significance of the useEffect hook in functional components
How do you manage state in a Redux store
How does React handle memoization
How do you handle forms in React with controlled and uncontrolled components
How to integrate Bootstrap with JavaScript libraries like jQuery
How to implement a modal/popup with Bootstrap