How does React handle component-based architecture
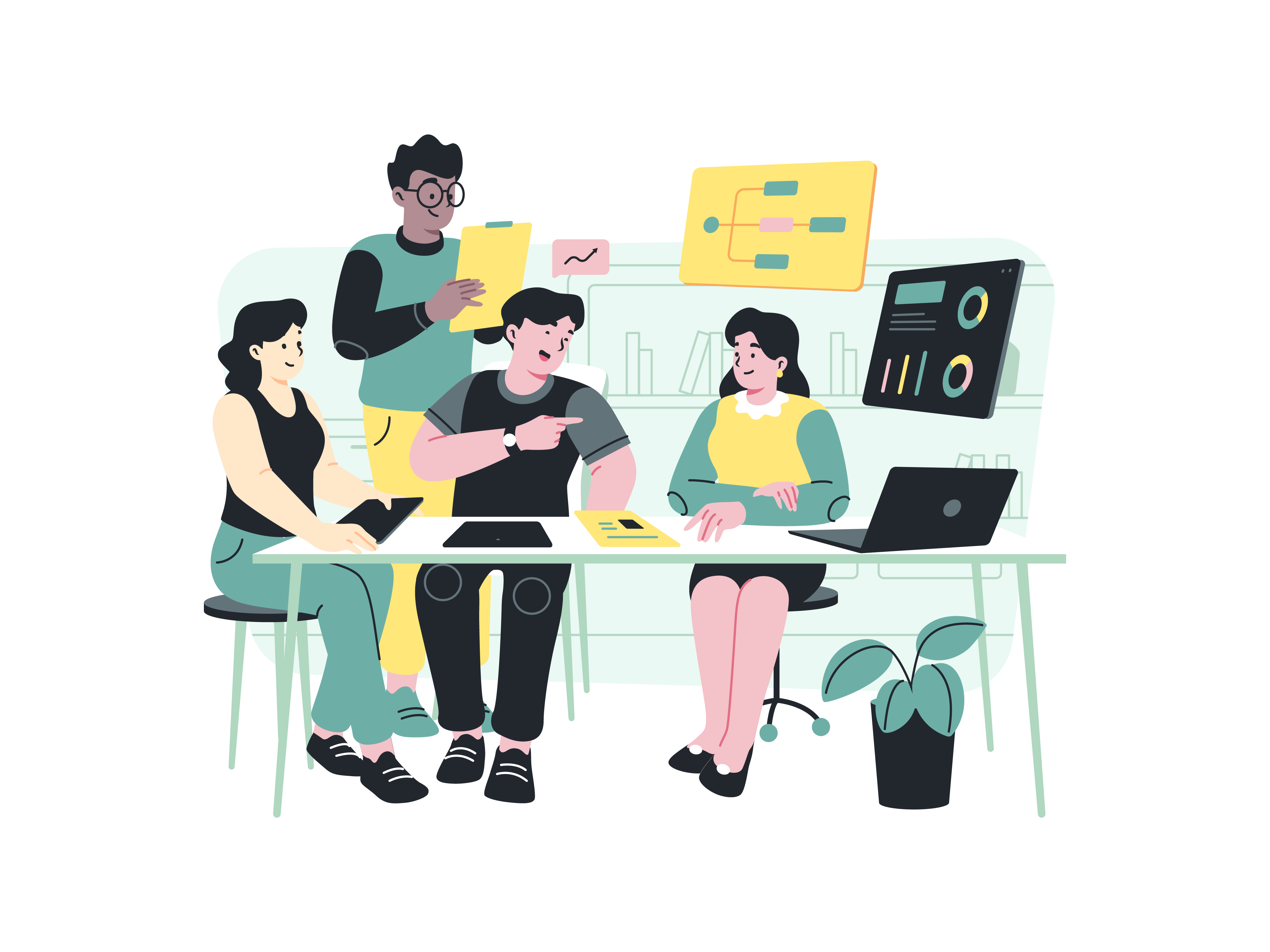
React is a JavaScript library for building user interfaces, and it is designed around the concept of a component-based architecture. This architecture allows you to break down your user interface into reusable and self-contained building blocks called components. Each component represents a part of the user interface and can have its own state, properties, and lifecycle methods.
Here are key concepts related to React’s component-based architecture;
Components
- Functional Components: These are simple, stateless components that are defined as JavaScript functions. They take in props (properties) as input and return React elements to describe what should be rendered.
const FunctionalComponent = (props) => {
return <div>{props.message}</div>;
};
- Class Components: These are more powerful components that can have state and lifecycle methods. They are defined as ES6 classes.
class ClassComponent extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
render() {
return <div>{this.state.count}</div>;
}
}
-
Props (Properties)
- Components can receive data from their parent components through props. Props are immutable, and they are used to pass information down the component tree.
const ParentComponent = () => { return <ChildComponent message="Hello from parent!" />; }; const ChildComponent = (props) => { return <div>{props.message}</div>; };
State
- State represents the internal data of a component. It can change over time in response to user actions or other events. Class components have a
state
object that is managed by React.
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
increment = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
<div>
Count: {this.state.count}
<button onClick={this.increment}>Increment</button>
</div>
);
}
}
Lifecycle Methods
- Class components have lifecycle methods that allow you to perform actions at different points in the component’s life cycle, such as when it is mounted, updated, or unmounted.
class LifecycleExample extends React.Component {
componentDidMount() {
// Called after the component is rendered to the DOM
console.log('Component did mount');
}
componentWillUnmount() {
// Called before the component is removed from the DOM
console.log('Component will unmount');
}
render() {
return <div>Hello, Lifecycle!</div>;
}
}
Virtual DOM
- React uses a virtual DOM to improve performance. Instead of directly manipulating the actual DOM, React creates a virtual representation of it in memory. When state or props change, React calculates the difference (diffing) between the previous and new virtual DOM and only updates the necessary parts of the actual DOM.
These principles make React highly efficient, scalable, and easy to maintain. Component-based architecture encourages code reusability, modularity, and a clear separation of concerns in your application.
How to use Bootstrap’s utility classes for flexbox alignment
How does React differ from other JavaScript frameworks
What is JSX, and how does it differ from regular JavaScript syntax
Uncover React’s Virtual DOM: Exploring Its Crucial Role and Advantages
What are the key features of React