How do you handle authentication in a React application
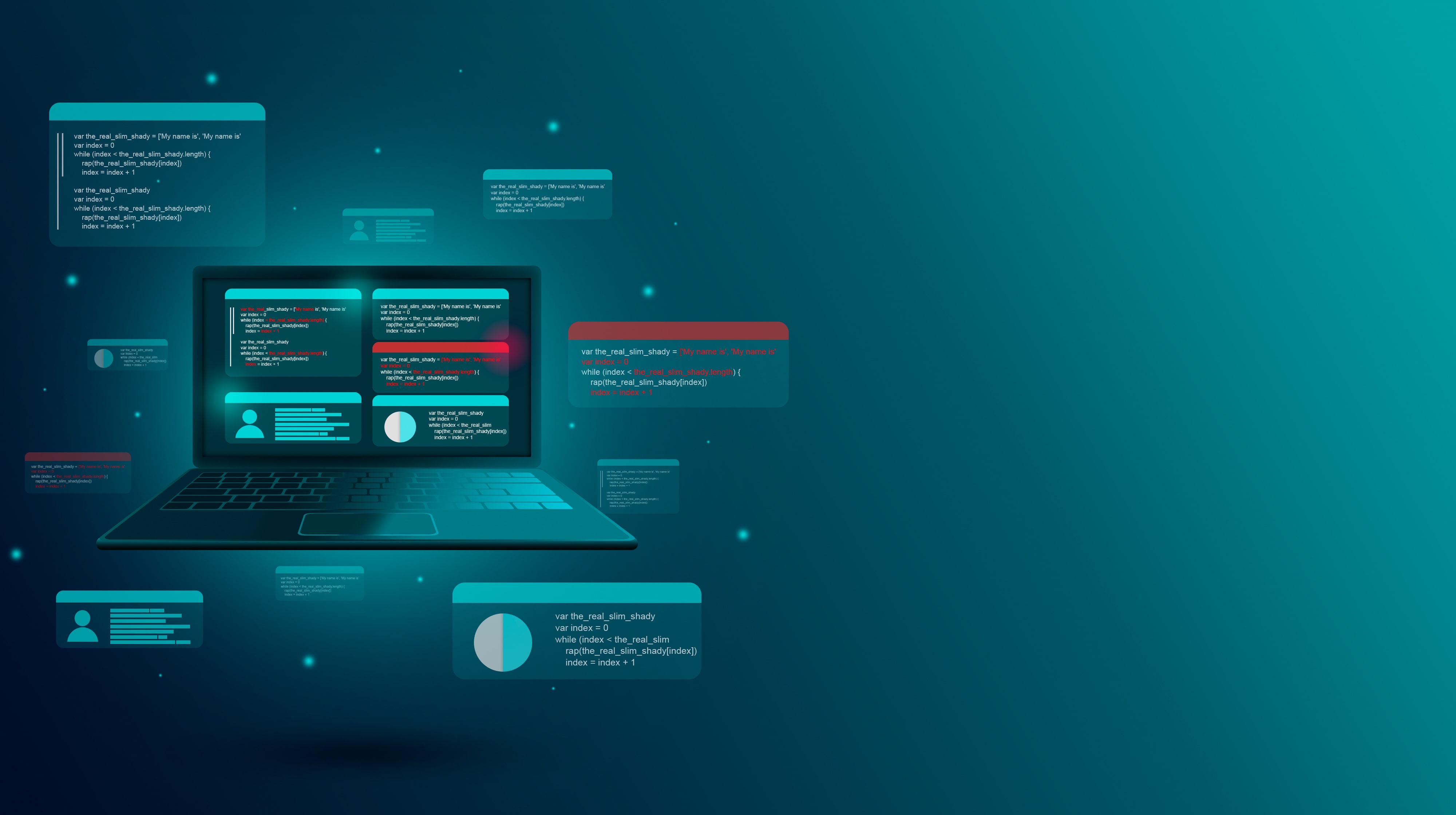
Authentication is a fundamental aspect of building secure and user-friendly web applications, and React provides developers with various strategies for implementing robust authentication systems. In this comprehensive guide, we’ll explore different approaches to handling authentication in React applications, covering essential concepts, common challenges, and best practices for ensuring a secure user authentication experience.
-
Understanding Authentication in Web Applications
Authentication is the process of verifying the identity of users, ensuring they have the appropriate credentials to access protected resources. In a React application, this typically involves managing user sessions, tokens, or other authentication mechanisms to grant or deny access based on user identity.
-
JWT (JSON Web Tokens) Authentication
JSON Web Tokens have become a popular choice for authentication in React applications. JWTs are compact, URL-safe means of representing claims between two parties. In a typical JWT authentication flow, the server generates a token upon successful user login, and this token is then included in subsequent requests to authenticate the user.
// Example of handling JWT authentication in React const handleLogin = async (username, password) => { const response = await fetch('/api/login', { method: 'POST', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify({ username, password }), }); if (response.ok) { const { token } = await response.json(); localStorage.setItem('token', token); } };
-
Session-based Authentication
Session-based authentication involves storing session data on the server, typically in a server-side store or a database. Upon successful login, the server generates a session identifier that is sent to the client. Subsequent requests include this session identifier, allowing the server to associate the request with a specific authenticated user.
// Example of handling session-based authentication in React const handleLogin = async (username, password) => { const response = await fetch('/api/login', { method: 'POST', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify({ username, password }), }); if (response.ok) { const { sessionId } = await response.json(); document.cookie = `sessionId=${sessionId}`; } };
-
OAuth and Social Media Authentication
OAuth is an authentication protocol that enables users to grant a third-party application limited access to their resources without sharing their credentials. Many React applications leverage OAuth for social media authentication, allowing users to log in using their existing accounts from platforms like Google, Facebook, or GitHub.
// Example of handling OAuth authentication in React const handleOAuthLogin = () => { window.location.href = '/api/oauth/google'; // Redirect to OAuth provider };
-
React Context and Global State Management
Managing authentication state across various components in a React application can be challenging. React Context, coupled with a global state management solution like Redux or React’s built-in useReducer and useContext, provides an elegant way to share authentication state throughout the application.
// Example of using React Context for authentication const AuthContext = createContext(); const AuthProvider = ({ children }) => { const [user, setUser] = useState(null); const login = (userData) => { setUser(userData); }; const logout = () => { setUser(null); }; return ( <AuthContext.Provider value={{ user, login, logout }}> {children} </AuthContext.Provider> ); }; const useAuth = () => useContext(AuthContext);
-
Secure Routes and Route Guards
Protecting certain routes from unauthorized access is a crucial aspect of authentication. React Router, a widely used routing library for React, allows developers to implement route guards by checking the authentication state before rendering specific components.
// Example of implementing route guards in React Router const PrivateRoute = ({ component: Component, ...rest }) => { const { user } = useAuth(); return ( <Route {...rest} render={(props) => user ? <Component {...props} /> : <Redirect to="/login" /> } /> ); };
-
Token Expiry and Refreshing
Managing token expiry is critical for maintaining the security of an authentication system. Refresh tokens, along with access tokens, can be employed to extend the user’s session without requiring a full login. React applications need to handle token expiry gracefully, redirecting users to re-authenticate when necessary.
-
Cross-Site Request Forgery (CSRF) Protection
Protecting against CSRF attacks is vital in web applications. Implementing measures such as anti-CSRF tokens and validating the origin of requests helps prevent attackers from performing unauthorized actions on behalf of authenticated users.
-
Securely Storing Credentials
Storing sensitive information, such as tokens or session identifiers, requires careful consideration. While browser storage options like localStorage and sessionStorage are convenient, using HttpOnly cookies for secure storage on the client side is a recommended practice.
-
Logging and Monitoring
Incorporating proper logging and monitoring mechanisms helps identify potential security issues or abnormal activities related to authentication. Monitoring failed login attempts, unusual access patterns, and unexpected token validations can enhance the overall security of the authentication system.
-
Best Practices and Considerations
- HTTPS Usage: Always use HTTPS to encrypt data transmitted between the client and server, preventing unauthorized access to sensitive information.
- Security Headers: Implement security headers, such as Content Security Policy (CSP) and Strict Transport Security (HSTS), to enhance the overall security posture of the application.
- Regular Security Audits: Conduct regular security audits and stay informed about emerging threats and best practices in web application security.
- Two-Factor Authentication (2FA): Consider implementing Two-Factor Authentication to add an extra layer of security for user accounts.
- Error Handling: Provide meaningful error messages to users during authentication to prevent information leakage that could be exploited by attackers.
-
Conclusion
Implementing authentication in a React application involves a combination of industry-standard practices, careful consideration of security implications, and leveraging the appropriate tools and libraries. By understanding the various authentication strategies, addressing common challenges, and incorporating best practices, developers can ensure the creation of secure, user-friendly, and resilient React applications in the ever-evolving landscape of web development.
How to implement a fixed sidebar with a scrollable content area
How to use Bootstrap’s responsive flexbox utilities
How to create a responsive timeline with vertical lines in Bootstrap
How to use Bootstrap’s card group for organizing content
How do I create a multi-column layout with Tailwind CSS
How do I handle dark mode in Tailwind CSS
How can I add a new font to my Tailwind CSS project