How does React handle animations
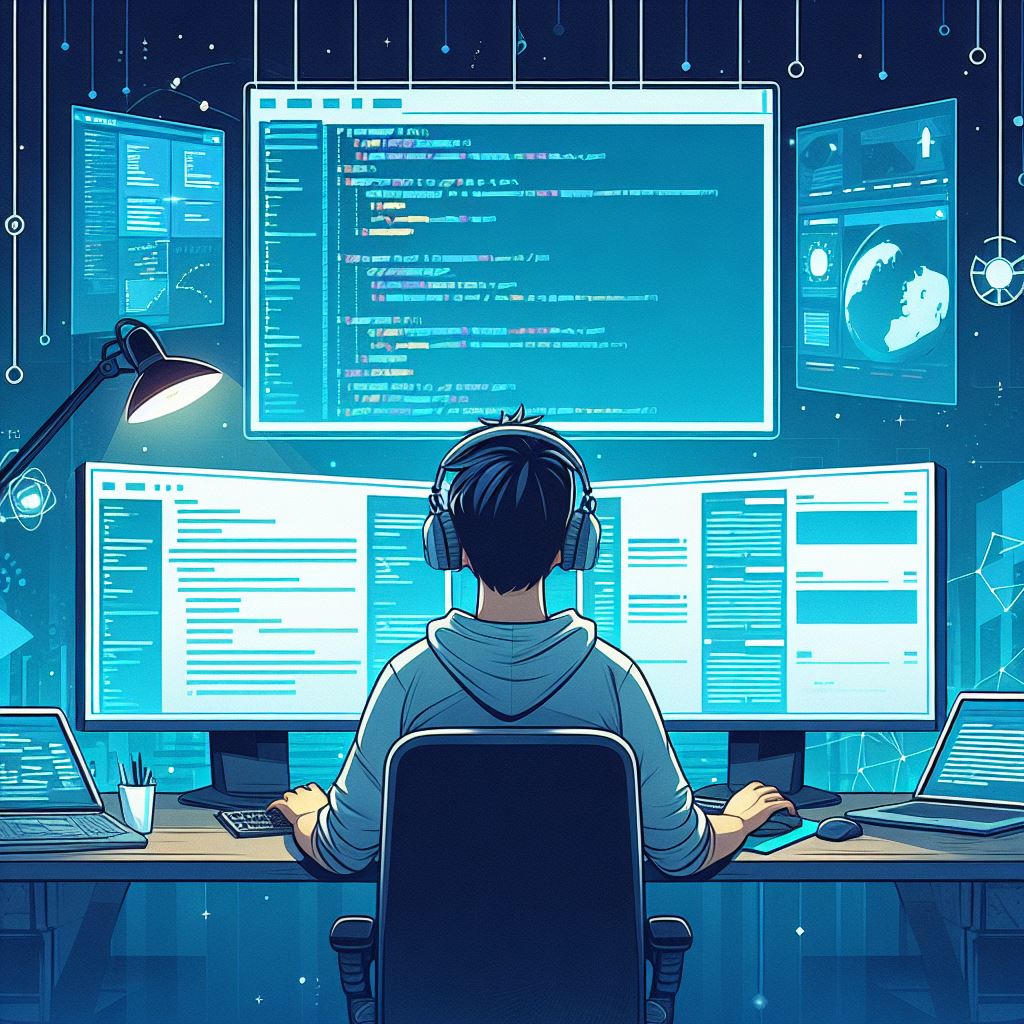
React, a powerhouse in the world of front-end development, has proven its mettle in creating dynamic and interactive user interfaces. When it comes to animations, React offers a robust system that seamlessly integrates with its component-based architecture. In this article, we’ll unravel the intricacies of how React handles animations, exploring its core principles, popular libraries, and best practices for creating smooth and engaging user experiences.
The Basics: React and the Virtual DOM
-
At the heart of React’s animation capabilities lies the Virtual DOM. React’s Virtual DOM is a lightweight representation of the actual DOM, enabling efficient updates and rendering. When state or props change, React calculates the difference between the current and new Virtual DOM, updating only the necessary parts of the actual DOM. This mechanism forms the foundation for handling animations in a performant manner.
-
CSS Transitions and Animations
React seamlessly integrates with CSS transitions and animations. By leveraging the
className
prop, developers can dynamically apply different CSS classes based on component state changes. This triggers CSS transitions or animations, providing a straightforward way to animate elements in response to user interactions or changing data.// Example: Using CSS transitions import React, { useState } from 'react'; import './Example.css'; const Example = () => { const [isOpen, setIsOpen] = useState(false); return ( <div className={`animated-container ${isOpen ? 'open' : 'closed'}`}> {/* Content goes here */} </div> ); };
-
React Transition Group
React Transition Group is a widely used library for managing component state transitions in React. It provides a set of components that assist in handling the lifecycle of animations, including entering, exiting, and transitioning states. The
CSSTransition
component, for instance, simplifies the process of applying CSS transitions based on component state changes.// Example: Using React Transition Group import React, { useState } from 'react'; import { CSSTransition } from 'react-transition-group'; import './Example.css'; const Example = () => { const [isOpen, setIsOpen] = useState(false); return ( <CSSTransition in={isOpen} timeout={300} classNames="fade" unmountOnExit > <div> {/* Content goes here */} </div> </CSSTransition> ); };
-
React Spring
React Spring is a powerful animation library that goes beyond traditional CSS-based animations. It utilizes a physics-based approach, providing a more natural and dynamic feel to animations. React Spring seamlessly integrates with React components, allowing for complex animations with a concise and declarative syntax.
// Example: Using React Spring import { useSpring, animated } from 'react-spring'; const Example = () => { const props = useSpring({ opacity: 1, from: { opacity: 0 } }); return <animated.div style={props}>Hello, React Spring!</animated.div>; };
-
Hooks for Animation
With the introduction of React Hooks, developers can manage component state and lifecycle in functional components. The
useEffect
hook is particularly useful for handling animations. By combining state anduseEffect
, developers can orchestrate animations in response to changes in component state.// Example: Using Hooks for Animation import React, { useState, useEffect } from 'react'; const Example = () => { const [isOpen, setIsOpen] = useState(false); useEffect(() => { // Perform animation logic based on isOpen }, [isOpen]); return ( <div> {/* Content goes here */} </div> ); };
-
Optimizing Performance
While React simplifies the process of handling animations, optimizing performance remains crucial. Minimizing unnecessary renders, leveraging hardware acceleration, and using libraries like React.memo for memoization are essential practices. Additionally, the
useLayoutEffect
hook can be employed to perform animations before the browser repaints, ensuring smoother transitions. -
Best Practices for Animation in React
- Declarative Animations Adopt a declarative approach to animations, describing what the animation should look like based on the component’s state.
- Hardware Acceleration
Leverage hardware acceleration for smoother animations by using CSS properties like
transform
andopacity
. - Avoid Direct DOM Manipulation Refrain from direct DOM manipulation, as it may conflict with React’s Virtual DOM reconciliation and lead to unpredictable behavior.
- Consider Performance Impact Be mindful of the performance impact of animations, especially on less powerful devices. Consider using libraries like Lottie for complex animations.
- Test Across Browsers Test animations across different browsers to ensure compatibility and a consistent user experience.
Conclusion
In the dynamic landscape of web development, React’s approach to handling animations stands out for its simplicity and flexibility. Whether through CSS transitions, React Transition Group, React Spring, or custom hooks, React provides developers with a plethora of tools to create engaging and performant animations. By understanding the underlying principles and adopting best practices, developers can unlock the full potential of React’s animation capabilities, delivering seamless and delightful user experiences.
What is the significance of the should Component Update method
How to integrate Bootstrap with JavaScript libraries like jQuery
How to implement a modal/popup with Bootstrap
How to center elements in Bootstrap
How to customize the Bootstrap theme