How to use Bootstrap's utility classes for flexbox alignment
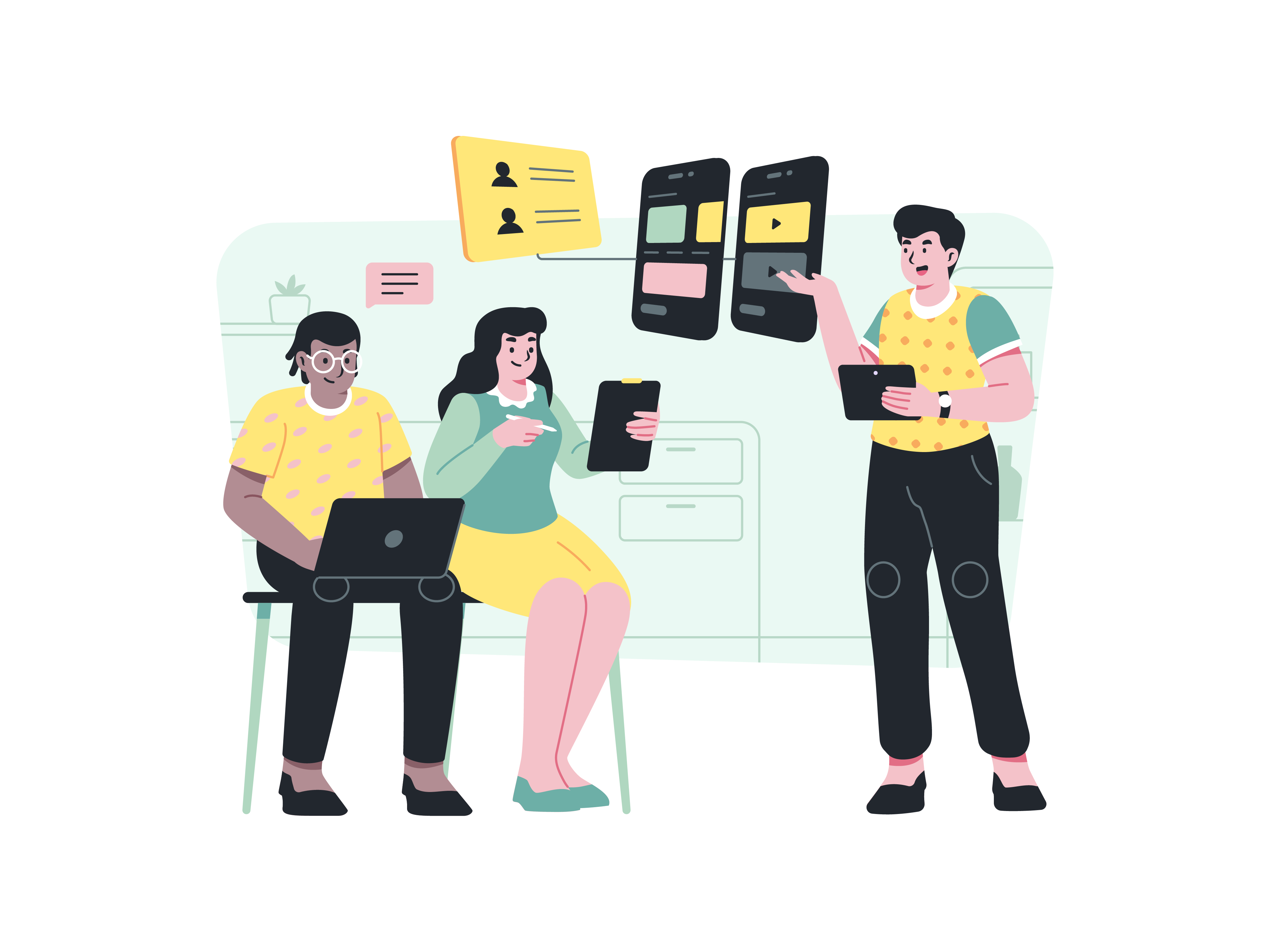
Flexbox, a powerful layout model in CSS, has revolutionized the way we design and structure web layouts. Bootstrap, a leading front-end framework, simplifies the use of Flexbox with a range of utility classes. In this comprehensive guide, we’ll explore how to leverage Bootstrap’s utility classes for Flexbox alignment. Whether you’re a seasoned developer or a newcomer to web design, mastering these classes will empower you to create flexible and responsive layouts effortlessly.
Understanding Flexbox and Its Power
Before we delve into Bootstrap’s utility classes, let’s briefly understand Flexbox and why it’s a game-changer in web design.
Flexbox, or the Flexible Box Layout, is a one-dimensional layout model that allows you to design complex web layouts with a more efficient and predictable structure. It brings flexibility to container elements and their children, enabling easy alignment, distribution of space, and ordering of items, regardless of their size or dynamic content.
Now, let’s explore how Bootstrap’s utility classes seamlessly integrate with Flexbox, offering a streamlined approach to achieving common alignment tasks.
Setting Up Your Project
Before using Bootstrap’s utility classes, ensure you have Bootstrap integrated into your project. You can either download the Bootstrap files and include them locally or use a Content Delivery Network (CDN). Here’s a basic HTML template with Bootstrap included:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link href="path/to/bootstrap.min.css" rel="stylesheet">
<title>Flexbox Alignment with Bootstrap</title>
</head>
<body>
<!-- Your content goes here -->
<script src="path/to/bootstrap.bundle.min.js"></script>
</body>
</html>
Replace “path/to/bootstrap.min.css” and “path/to/bootstrap.bundle.min.js” with the correct file paths or URLs.
Aligning Items with Flexbox
Bootstrap’s utility classes for Flexbox alignment are intuitive and easy to use. Let’s explore some common alignment scenarios and how to achieve them.
1. Centering Items Horizontally
To horizontally center items within a container, use the justify-content-center
class on the container:
```html
<div class="d-flex justify-content-center">
<!-- Your centered content goes here -->
</div>
This class applies Flexbox’s justify-content
property, aligning items along the horizontal axis and centering them within the container.
2. Centering Items Vertically
To vertically center items within a container, use the align-items-center
class on the container:
```html
<div class="d-flex align-items-center">
<!-- Your centered content goes here -->
</div>
This class applies Flexbox’s align-items
property, aligning items along the vertical axis and centering them within the container.
3. Centering Items Both Horizontally and Vertically
For both horizontal and vertical centering, combine the justify-content-center
and align-items-center
classes:
```html
<div class="d-flex justify-content-center align-items-center">
<!-- Your centered content goes here -->
</div>
This combination ensures both axes are centered, creating a perfectly centered layout.
4. Aligning Items to the Start or End
To align items to the start or end of a container, use the justify-content-start
or justify-content-end
classes:
```html
<div class="d-flex justify-content-start">
<!-- Your content aligned to the start -->
</div>
<div class="d-flex justify-content-end">
<!-- Your content aligned to the end -->
</div>
These classes control the horizontal alignment along the main axis of the container.
5. Wrapping Items
When dealing with multiple items, you may want them to wrap onto a new line if they exceed the container width. Use the flex-wrap
class:
```html
<div class="d-flex flex-wrap">
<!-- Your content that wraps onto a new line if necessary -->
</div>
This class ensures that items wrap onto a new line as needed, preventing overflow.
Responsive Flexbox Alignment
Bootstrap’s utility classes seamlessly adapt to different screen sizes, making your layouts responsive. To further enhance responsiveness, include the Bootstrap viewport meta tag in the <head>
section of your HTML:
```html
<meta name="viewport" content="width=device-width, initial-scale=1.0">
This meta tag ensures proper scaling on various devices.
Customizing Flexbox Alignment
Bootstrap provides additional utility classes that allow you to fine-tune your Flexbox alignment. For example, you can use the justify-content-between
class to evenly distribute items along the main axis with maximum space between them.
```html
<div class="d-flex justify-content-between">
<!-- Your content with space distributed between items -->
</div>
Explore Bootstrap’s documentation to discover a plethora of utility classes for Flexbox alignment and customize your layouts to perfection.
Conclusion
Bootstrap’s utility classes for Flexbox alignment empower developers and designers to create flexible and responsive layouts effortlessly. By understanding and leveraging these classes, you can achieve precise alignment, distribution of space, and ordering of items within your containers. Whether you’re centering content, aligning to the start or end, or enabling item wrapping, Bootstrap’s intuitive utility classes make it a breeze to implement complex layouts with minimal effort. Elevate your web design skills by incorporating Flexbox alignment into your Bootstrap projects and unlock the full potential of modern, responsive layouts.
How to use Bootstrap’s text truncation classes for long content
How to create a responsive contact form with validation in Bootstrap
How to use Bootstrap’s container and container-fluid classes
How to implement a sticky header with Bootstrap
How to implement a card flip animation with Bootstrap
How to use Bootstrap’s table-responsive class