What are the key features of React
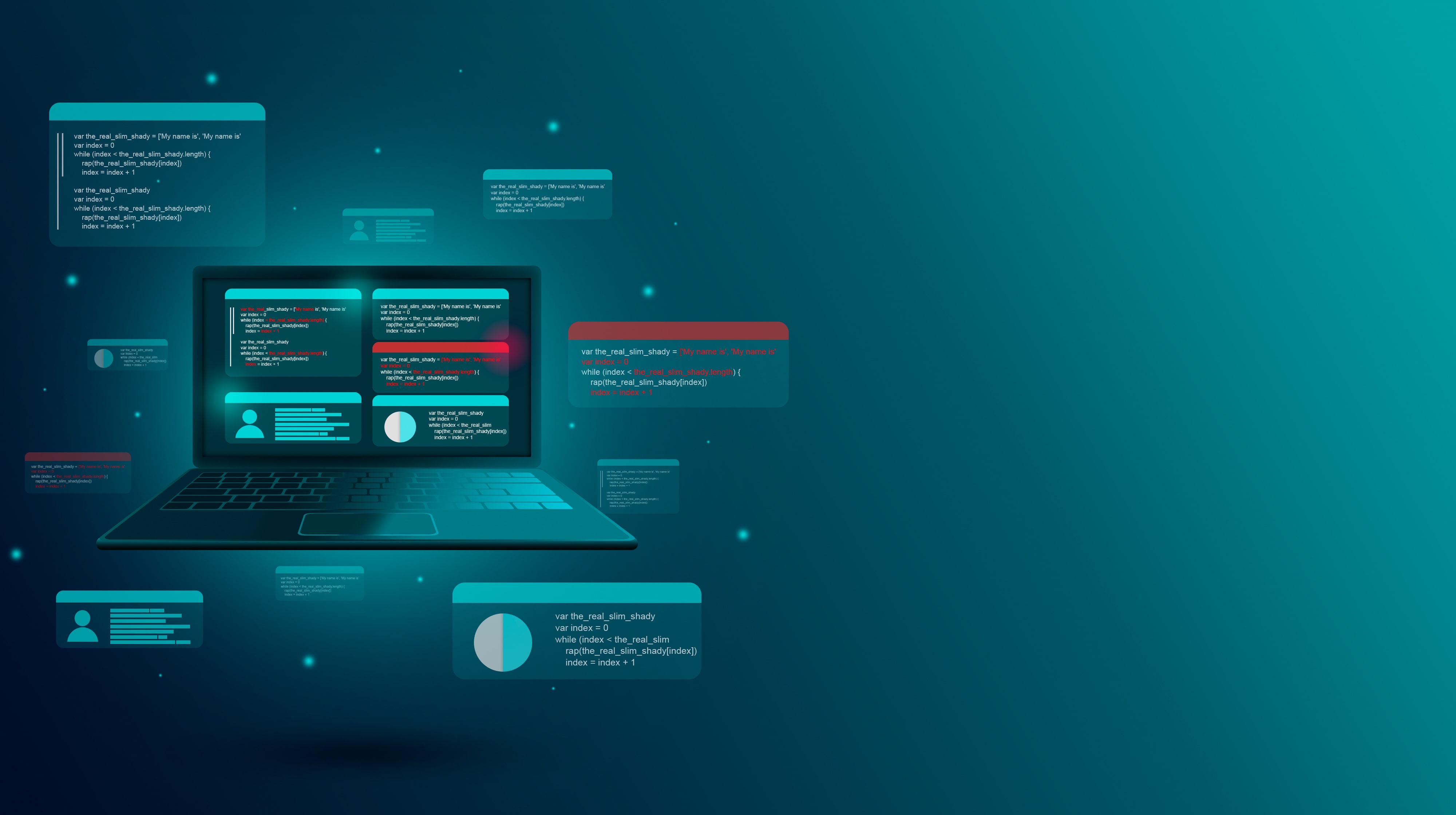
React, a JavaScript library developed by Facebook, has become a powerhouse in the world of front-end development. Renowned for its flexibility, efficiency, and component-based architecture, React has significantly influenced the way developers approach building user interfaces. In this article, we will delve into the key features that make React a standout choice for web development.
1. Component-Based Architecture
At the core of React’s design is its component-based architecture. Components are independent, reusable building blocks that encapsulate a part of the user interface. This modular approach makes it easier to manage and maintain code, encourages reusability, and facilitates a clear and organized structure for complex applications.
// Example of a React component
class MyComponent extends React.Component {
render() {
return <p>Hello, React!</p>;
}
}
2. Virtual DOM
React introduces the concept of the Virtual DOM, a lightweight in-memory representation of the actual DOM. When the state of a React component changes, a new Virtual DOM is created and compared with the previous one. React then efficiently updates the actual DOM with only the necessary changes. This process significantly improves performance by minimizing direct manipulation of the DOM and reducing unnecessary re-rendering.
3. JSX (JavaScript XML)
JSX is a syntax extension for JavaScript that allows developers to write XML-like code within JavaScript. It provides a more readable and expressive way to define the structure of components. JSX gets transpiled to regular JavaScript, making it compatible with browsers. This approach simplifies the creation of UI components and enhances the overall development experience.
// Example of JSX in React
const element = <h1>Hello, JSX!</h1>;
4. One-Way Data Binding
React enforces a unidirectional data flow, meaning that data flows in a single direction—from parent components to child components. This approach makes it easier to trace and manage the flow of data within an application. Parent components pass data down to child components through props, and any required changes are communicated back through callback functions.
5. React Native
React extends its capabilities beyond the web with React Native, a framework for building mobile applications using JavaScript and React. Developers can leverage their React skills to create cross-platform mobile apps, sharing a significant portion of the codebase between web and mobile applications. This streamlined approach enhances efficiency and accelerates development for multiple platforms.
// Example of React Native component
import React from 'react';
import { Text, View } from 'react-native';
class MyMobileComponent extends React.Component {
render() {
return (
<View>
<Text>Hello, React Native!</Text>
</View>
);
}
}
6. Declarative Syntax
React uses a declarative syntax, allowing developers to describe how the UI should look based on the current state. Instead of imperatively specifying each step to achieve a desired state, developers can focus on declaring the desired outcome. This makes the code more expressive, readable, and easier to understand.
7. Efficient State Management
React provides a robust state management system that allows components to manage their own state. When a component’s state changes, React efficiently updates the affected parts of the UI. The use of the setState
function ensures that state changes trigger the necessary updates while maintaining predictability and control.
// Example of state management in React
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
increment = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.increment}>Increment</button>
</div>
);
}
}
8. Lifecycle Methods
React components have a lifecycle that consists of various phases, such as mounting, updating, and unmounting. React provides lifecycle methods that developers can utilize to execute code at specific points in a component’s lifecycle. This capability is instrumental in managing resources, performing side effects, and optimizing performance.
// Example of a lifecycle method in React
class MyComponent extends React.Component {
componentDidMount() {
// Code to execute after the component is rendered
}
render() {
return <p>Hello, React!</p>;
}
}
9. Reusable Components
React’s component-based architecture promotes reusability. Developers can create modular components and reuse them across different parts of the application. This not only enhances code organization but also simplifies the process of scaling and maintaining applications.
10. Community and Ecosystem
React boasts a large and active community of developers. This community contributes to a vast ecosystem of libraries, tools, and resources that complement and enhance React development. The availability of a rich ecosystem accelerates development, as developers can leverage pre-built solutions and share knowledge within the community.
Conclusion
React’s key features, including its component-based architecture, Virtual DOM, JSX syntax, one-way data binding, React Native for mobile development, declarative syntax, efficient state management, lifecycle methods, reusable components, and a thriving community, collectively contribute to its popularity and effectiveness in building modern web applications. By understanding and harnessing these features, developers can create scalable, efficient, and maintainable user interfaces that meet the demands of today’s dynamic web development landscape. Whether you’re a seasoned developer or just starting with React, these features form the foundation for a powerful and versatile development experience.
How to use Bootstrap’s utility classes for flexbox alignment
How does React differ from other JavaScript frameworks
What is JSX, and how does it differ from regular JavaScript syntax
How do I create a multi-column layout with Tailwind CSS
How do I handle dark mode in Tailwind CSS
How can I add a new font to my Tailwind CSS project
How to create a responsive timeline with vertical lines in Bootstrap