What are the best practices for managing state in a React application
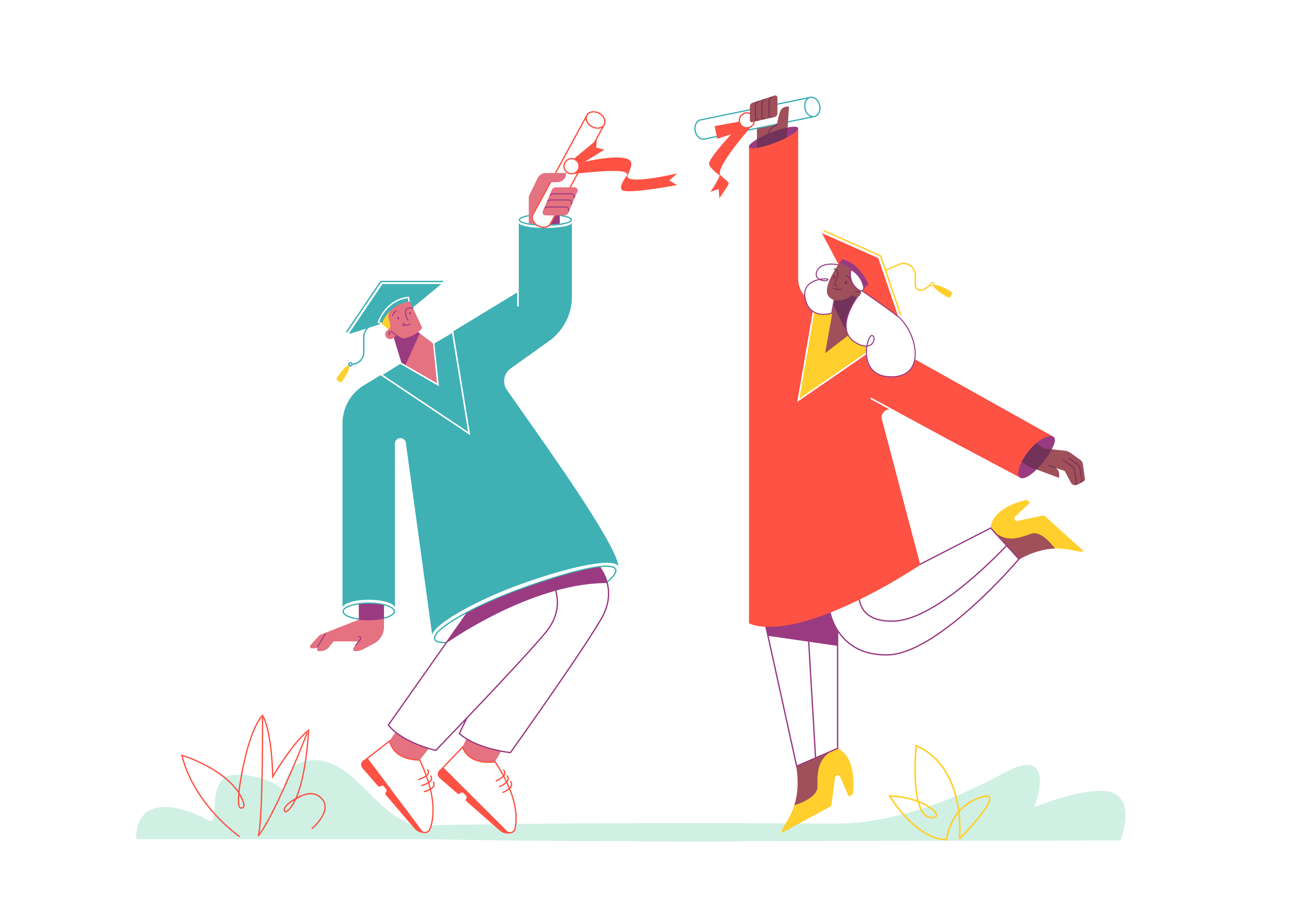
State management is a cornerstone of React development, influencing the responsiveness and efficiency of applications. As React applications grow in complexity, adopting best practices for managing state becomes imperative. In this comprehensive guide, we will delve into the key principles and practices that empower developers to effectively handle state in React applications, ensuring scalability, maintainability, and optimal performance.
1. Understand React State
Before diving into best practices, it’s crucial to understand the fundamentals of React state. State in React is a JavaScript object that represents the parts of a component that can change over time. It influences the rendering of the component and enables dynamic user interfaces.
2. Use Functional Components with Hooks
With the introduction of React Hooks, functional components can now manage state and side effects, eliminating the need for class components in many cases. useState
allows functional components to declare state variables, promoting a more concise and readable code structure.
import React, { useState } from 'react';
const MyComponent = () => {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
3. Separate Concerns with Multiple State Hooks
For components with multiple state variables, consider using separate useState
hooks for each concern. This improves code organization and makes it easier to reason about the component’s state.
const MyComponent = () => {
const [username, setUsername] = useState('');
const [password, setPassword] = useState('');
const [isLoggedIn, setLoggedIn] = useState(false);
// Component logic using state variables
};
4. Immutability and State Updates
React state should be treated as immutable. When updating state based on its current value, use the functional form of the state update function provided by useState
. This ensures that state updates are based on the latest state and prevents race conditions.
const MyComponent = () => {
const [count, setCount] = useState(0);
const increment = () => {
setCount((prevCount) => prevCount + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
};
5. Context API for Global State
When dealing with global state that needs to be shared across multiple components, consider using React’s Context API. It provides a way to pass data through the component tree without having to pass props manually at every level.
// Context creation
const MyContext = React.createContext();
const MyProvider = ({ children }) => {
const [globalState, setGlobalState] = useState(initialState);
return (
<MyContext.Provider value={{ globalState, setGlobalState }}>
{children}
</MyContext.Provider>
);
};
// Using the context in a component
const MyComponent = () => {
const { globalState, setGlobalState } = useContext(MyContext);
// Component logic using global state
};
6. Redux for Complex State Management
For large-scale applications with complex state management needs, Redux remains a popular choice. It provides a predictable state container and enables centralized management of state through actions and reducers.
// Redux actions and reducers
const increment = () => ({ type: 'INCREMENT' });
const counterReducer = (state = 0, action) => {
switch (action.type) {
case 'INCREMENT':
return state + 1;
default:
return state;
}
};
// Redux store setup
const store = createStore(counterReducer);
// Using Redux in a component
const MyComponent = () => {
const count = useSelector((state) => state);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => dispatch(increment())}>Increment</button>
</div>
);
};
7. Memoization for Performance Optimization
To prevent unnecessary re-renders and optimize performance, consider using memoization techniques. The useMemo
hook in React allows the memoization of values that depend on specific dependencies.
const MyComponent = () => {
const expensiveCalculation = useMemo(() => {
// Perform expensive calculation
return result;
}, [dependency]);
// Component logic using memoized value
};
8. Avoid Unnecessary Component Rerenders
Optimize component rendering by avoiding unnecessary re-renders. Use the React.memo
higher-order component to memoize functional components and prevent rerenders when props haven’t changed.
const MemoizedComponent = React.memo(({ prop }) => {
// Component logic
});
9. Component Composition for Maintainability
Break down complex components into smaller, manageable pieces through component composition. This not only enhances maintainability but also allows for more granular state management within the application.
const ParentComponent = () => {
return (
<div>
<ChildComponent1 />
<ChildComponent2 />
</div>
);
};
10. Thorough Testing of Stateful Components
Ensure the reliability of stateful components by
writing comprehensive unit tests. Libraries like Jest and React Testing Library provide tools to simulate component behavior and validate state changes.
test('component updates state correctly', () => {
render(<MyComponent />);
fireEvent.click(screen.getByText('Increment'));
expect(screen.getByText('Count: 1')).toBeInTheDocument();
});
Conclusion
Effective state management is a foundational aspect of React development, influencing the performance, scalability, and maintainability of applications. By adhering to best practices such as using functional components with hooks, embracing immutability, and considering context or Redux for state elevation, developers can create React applications that are not only efficient but also scalable and maintainable as they grow in complexity.
As the React ecosystem continues to evolve, staying informed about emerging patterns and tools ensures that developers can leverage the latest advancements to optimize state management in their applications. With a solid understanding of state management best practices, developers are well-equipped to build robust and responsive React applications that meet the demands of modern web development.
How does React handle the key prop when components are re-ordered in a list
What is Redux, and how does it integrate with React
Explain the concept of higher-order components (HOCs) in React
What is the purpose of middleware in Redux