What is the purpose of middleware in Redux
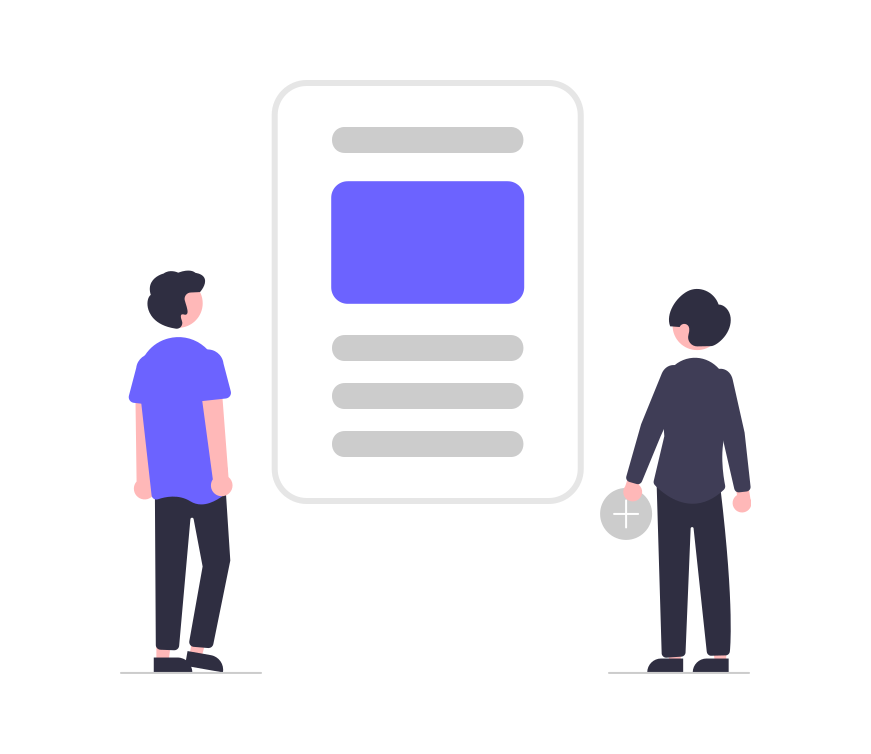
Redux, a powerful state management library for JavaScript applications, provides a predictable and centralized way to manage the state of an application. At the heart of Redux lies middleware, a crucial concept that allows developers to extend and customize the behavior of Redux’s dispatch process. In this comprehensive guide, we will delve into the purpose of middleware in Redux, understanding its role, and exploring how it empowers developers to handle asynchronous actions, log data, and implement various cross-cutting concerns.
Understanding Redux Middleware
1. Middleware Defined
Middleware in Redux acts as a bridge between the moment an action is dispatched and the moment it reaches the reducer. It intercepts actions, allowing developers to introduce additional functionality before the action reaches the reducer or after the reducer has processed the action.
2. The Middleware Chain
The middleware mechanism operates in the form of a chain, where each middleware in the chain has the ability to process an action and pass it along to the next middleware or terminate the chain. This enables the sequential execution of middleware functions.
const middleware1 = (store) => (next) => (action) => {
// Middleware logic before the action reaches the reducer
next(action);
// Middleware logic after the action is processed by the reducer
};
const middleware2 = (store) => (next) => (action) => {
// Middleware logic
next(action);
};
// Creating the middleware chain
const middlewareChain = [middleware1, middleware2];
The next
function represents the next middleware in the chain or the final dispatch function if there are no more middlewares.
3. Common Use Cases
Middleware in Redux is incredibly versatile and can be employed for various purposes, including:
- Handling asynchronous actions.
- Logging data and actions.
- Implementing authentication and authorization checks.
- Modifying or intercepting actions based on certain conditions.
- Integrating with third-party libraries.
Practical Applications of Middleware
1. Handling Asynchronous Actions
One of the primary use cases for middleware in Redux is handling asynchronous actions. Traditional Redux flow relies on synchronous actions, but middleware allows for asynchronous operations, such as API calls or timeouts.
const asyncMiddleware = (store) => (next) => (action) => {
if (typeof action === 'function') {
return action(store.dispatch, store.getState);
}
return next(action);
};
This middleware checks if the action is a function (common in asynchronous actions) and invokes it with the dispatch
and getState
functions.
2. Logging Actions
Middleware can be instrumental in logging information about dispatched actions, aiding in debugging and monitoring application behavior.
const loggerMiddleware = (store) => (next) => (action) => {
console.log('Dispatching:', action);
next(action);
console.log('State after dispatch:', store.getState());
};
The loggerMiddleware
logs information before and after an action is processed by the reducer.
3. Authentication and Authorization
Middleware can implement security-related concerns, such as authentication and authorization checks before allowing certain actions to proceed.
const authMiddleware = (store) => (next) => (action) => {
if (action.requiresAuth && !isUserAuthenticated()) {
console.error('Unauthorized access. Action aborted.');
return;
}
next(action);
};
Here, the middleware checks if the action requires authentication and prevents the action if the user is not authenticated.
Implementing Custom Middleware
1. Creating Custom Middleware
Developers can create custom middleware tailored to the specific needs of their application. Custom middleware follows the same pattern of receiving store
and next
as arguments and returning a function that receives action
.
const customMiddleware = (store) => (next) => (action) => {
// Custom middleware logic
next(action);
};
2. Adding Middleware to the Store
Middleware is added to the Redux store during its creation using the applyMiddleware
function from the Redux library.
import { createStore, applyMiddleware } from 'redux';
const store = createStore(
rootReducer,
initialState,
applyMiddleware(middleware1, middleware2, customMiddleware)
);
The middleware functions are passed as arguments to applyMiddleware
.
Best Practices and Considerations
1. Order of Middleware Matters
The order in which middleware is applied can impact the behavior of the application. Consider the sequence carefully, as it determines the order in which middleware functions will be executed.
2. Keep Middleware Simple
Middleware functions are most effective when they perform focused, specific tasks. Avoid creating monolithic middleware that handles too many concerns.
3. Testing Middleware
Test middleware independently to ensure it behaves as expected. Libraries like redux-mock-store
can assist in testing the interaction between middleware and the store.
4. Understand the Dispatch Process
Familiarize yourself with the dispatch process in Redux to make informed decisions about where to place middleware in the chain and how to interact with actions.
Limitations and Future Developments
1. Middleware Composition
While middleware allows for sequential execution, composing middleware can become complex. Future developments in Redux may introduce improvements or alternative patterns for middleware composition.
2. Async/Await in Middleware
Although middleware can handle asynchronous actions, the use of async/await
directly within middleware functions may not work as expected. Developers should consider alternative patterns for handling asynchronous logic.
3. Redux Toolkit and Middleware
The Redux Toolkit introduces a simplified syntax for creating stores and reducers, and its createSlice
function includes an extraReducers
option for handling additional logic. This may influence the role and usage of middleware in future Redux applications.
Conclusion
Middleware in Redux plays a pivotal role in extending and customizing the dispatch process, enabling developers to handle asynchronous actions, log data, and implement a variety of cross-cutting concerns. By understanding the purpose and applications of middleware, Redux developers can optimize state management and enhance the overall functionality of their applications.
As the landscape of web development evolves, so too may the role and capabilities of middleware in Redux. Staying informed about best practices and potential advancements ensures that developers are equipped to harness the full power of middleware for effective state management in their React applications.
How does React differ from other JavaScript frameworks
What is JSX, and how does it differ from regular JavaScript syntax
Uncover React’s Virtual DOM: Exploring Its Crucial Role and Advantages
What are the key features of React
How does React handle component-based architecture
What is the significance of state in React
How can you optimize performance in React applications
How does React handle the key prop when components are re-ordered in a list