How does React handle forms and form validation
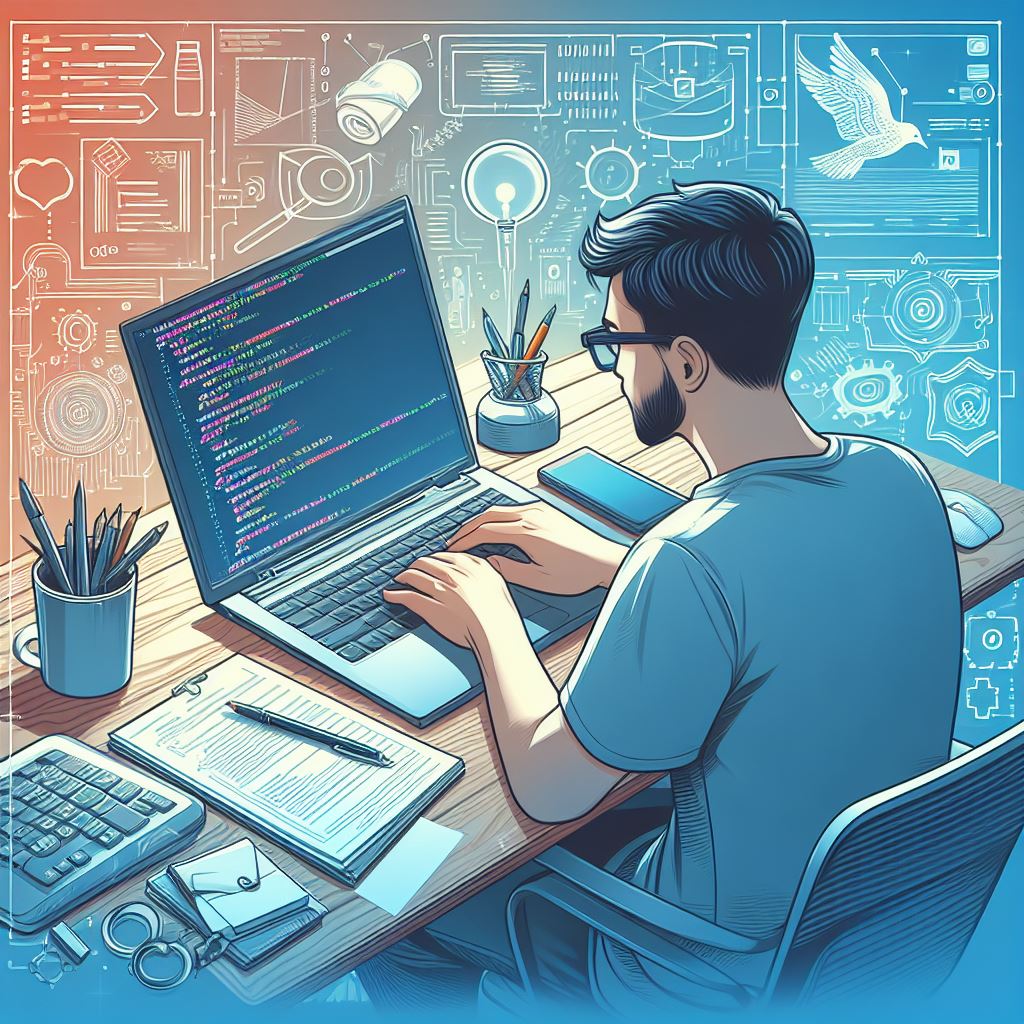
In the realm of web development, forms play a crucial role in user interaction and data submission. React, with its declarative and component-based approach, provides an efficient mechanism for handling forms and implementing validation. In this comprehensive guide, we will explore how React handles forms, the principles behind form validation, and best practices for creating robust and user-friendly form experiences.
Understanding Form Handling in React
1. Controlled Components
React introduces the concept of controlled components for handling forms. In a controlled component, form elements like input fields are controlled by the component’s state. This means that the value of the input is tied to the component’s state, and any change is reflected in the component’s state, triggering a re-render.
import React, { useState } from 'react';
const MyForm = () => {
const [inputValue, setInputValue] = useState('');
const handleChange = (e) => {
setInputValue(e.target.value);
};
return (
<form>
<input type="text" value={inputValue} onChange={handleChange} />
</form>
);
};
2. Uncontrolled Components
Alternatively, React allows the use of uncontrolled components, where the form elements maintain their own state. While less common, uncontrolled components can be useful in certain scenarios, particularly when integrating with non-React code or libraries.
import React, { useRef } from 'react';
const MyForm = () => {
const inputRef = useRef();
const handleSubmit = (e) => {
e.preventDefault();
console.log('Input Value:', inputRef.current.value);
};
return (
<form onSubmit={handleSubmit}>
<input type="text" ref={inputRef} />
<button type="submit">Submit</button>
</form>
);
};
Implementing Form Validation
1. Using State for Validation
Form validation often involves checking whether the input meets specific criteria. React leverages state to store not only the input values but also the validation status.
const MyForm = () => {
const [inputValue, setInputValue] = useState('');
const [isValid, setIsValid] = useState(true);
const handleChange = (e) => {
const value = e.target.value;
setInputValue(value);
setIsValid(value.length > 5); // Example: Validate input length
};
return (
<form>
<input
type="text"
value={inputValue}
onChange={handleChange}
className={isValid ? 'valid' : 'invalid'}
/>
<button type="submit" disabled={!isValid}>
Submit
</button>
</form>
);
};
2. Real-Time Validation Feedback
Providing users with real-time feedback on the validity of their input enhances the user experience. This can be achieved by dynamically updating the UI based on the validation status.
const MyForm = () => {
const [inputValue, setInputValue] = useState('');
const [isValid, setIsValid] = useState(true);
const handleChange = (e) => {
const value = e.target.value;
setInputValue(value);
setIsValid(value.length > 5);
};
return (
<form>
<input
type="text"
value={inputValue}
onChange={handleChange}
className={isValid ? 'valid' : 'invalid'}
/>
<span className={`validation-message ${isValid ? 'valid' : 'invalid'}`}>
{isValid ? 'Valid Input' : 'Input must be at least 6 characters'}
</span>
<button type="submit" disabled={!isValid}>
Submit
</button>
</form>
);
};
Best Practices for Form Handling and Validation
1. Use Descriptive Labels
Clearly label form elements to guide users in providing the required information. Descriptive labels improve accessibility and user understanding.
2. Provide Feedback
Offer real-time feedback on the validity of user input. Whether through color changes, error messages, or icons, visual cues help users understand the status of their input.
3. Handle Form Submission
Prevent the default form submission behavior and handle it through a function. This allows for additional processing, such as making API calls, before navigating away from the current page.
4. Consider Accessibility
Ensure that your forms are accessible to users with disabilities. This includes providing meaningful error messages, using ARIA attributes, and maintaining a logical tab order.
Libraries for Form Handling
1. Formik
Formik is a popular library for managing forms in React. It simplifies form creation, validation, and submission, reducing boilerplate code.
import { Formik, Form, Field, ErrorMessage } from 'formik';
const MyForm = () => {
return (
<Formik
initialValues={{ email: '' }}
validate={(values) => {
const errors = {};
if (!values.email) {
errors.email = 'Required';
}
return errors;
}}
onSubmit={(values, { setSubmitting }) => {
// Handle form submission
setSubmitting(false);
}}
>
<Form>
<Field type="email" name="email" />
<ErrorMessage name="email" component="div" />
<button type="submit">Submit</button>
</Form>
</Formik>
);
};
2. React Hook Form
React Hook Form is another library that simplifies form management by leveraging React hooks. It focuses on performance and flexibility.
import { useForm, Controller } from 'react-hook-form';
const MyForm = () => {
const { control, handleSubmit, formState } = useForm();
const onSubmit = (data) => {
// Handle form submission
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
<Controller
control={control}
name="email"
render={({ field }) => <input {...field} />}
rules={{ required: 'This field is required' }}
/>
{formState.errors.email && <div>{formState.errors.email.message}</div>}
<button type="submit">Submit</button>
</form>
);
};
Handling Complex Forms and Advanced Validation
1. Dynamic Form Fields
React allows for dynamic rendering of form fields based on user input. This is particularly useful for forms with dynamic requirements.
const DynamicForm = () => {
const [formFields, setFormFields] = useState([]);
const addFormField = () => {
setFormFields([...formFields, { name: '', value: '' }]);
};
const handleFieldChange = (index, e) => {
const newFormFields = [...formFields];
newFormFields[index][e.target.name] = e.target.value;
setFormFields(newFormFields);
};
return (
<form>
{formFields
.map((field, index) => (
<div key={index}>
<input
type="text"
name="name"
value={field.name}
onChange={(e) => handleFieldChange(index, e)}
/>
<input
type="text"
name="value"
value={field.value}
onChange={(e) => handleFieldChange(index, e)}
/>
</div>
))}
<button type="button" onClick={addFormField}>
Add Field
</button>
<button type="submit">Submit</button>
</form>
);
};
2. Formik Yup Integration for Schema Validation
Formik can be integrated with the Yup validation library to enforce a schema for form validation, offering a more structured and scalable approach.
import { Formik, Form, Field, ErrorMessage } from 'formik';
import * as Yup from 'yup';
const MyForm = () => {
const validationSchema = Yup.object().shape({
email: Yup.string().email('Invalid email').required('Required'),
password: Yup.string().min(6, 'Password must be at least 6 characters').required('Required'),
});
return (
<Formik
initialValues={{ email: '', password: '' }}
validationSchema={validationSchema}
onSubmit={(values, { setSubmitting }) => {
// Handle form submission
setSubmitting(false);
}}
>
<Form>
<Field type="email" name="email" />
<ErrorMessage name="email" component="div" />
<Field type="password" name="password" />
<ErrorMessage name="password" component="div" />
<button type="submit">Submit</button>
</Form>
</Formik>
);
};
Conclusion
Mastering form handling and validation in React is essential for building interactive and user-friendly web applications. By understanding the principles of controlled components, form validation techniques, and leveraging popular libraries like Formik or React Hook Form, developers can streamline the development process and enhance the overall user experience.
As the landscape of web development evolves, staying informed about emerging patterns and tools ensures that developers are equipped to tackle the challenges of building robust and dynamic forms in React applications. With the right techniques and practices, developers can create forms that not only collect data but also provide a seamless and intuitive experience for users interacting with their applications.
How to use Bootstrap’s container and container-fluid classes
How to implement a sticky header with Bootstrap
How to use Bootstrap’s utility classes for flexbox alignment
How does React differ from other JavaScript frameworks
How to use Bootstrap’s responsive embed classes for videos
How to create a responsive contact form with Bootstrap
What is the difference between the minified and unminified versions of Tailwind CSS