What is the purpose of the "purge" option in the Tailwind CSS configuration
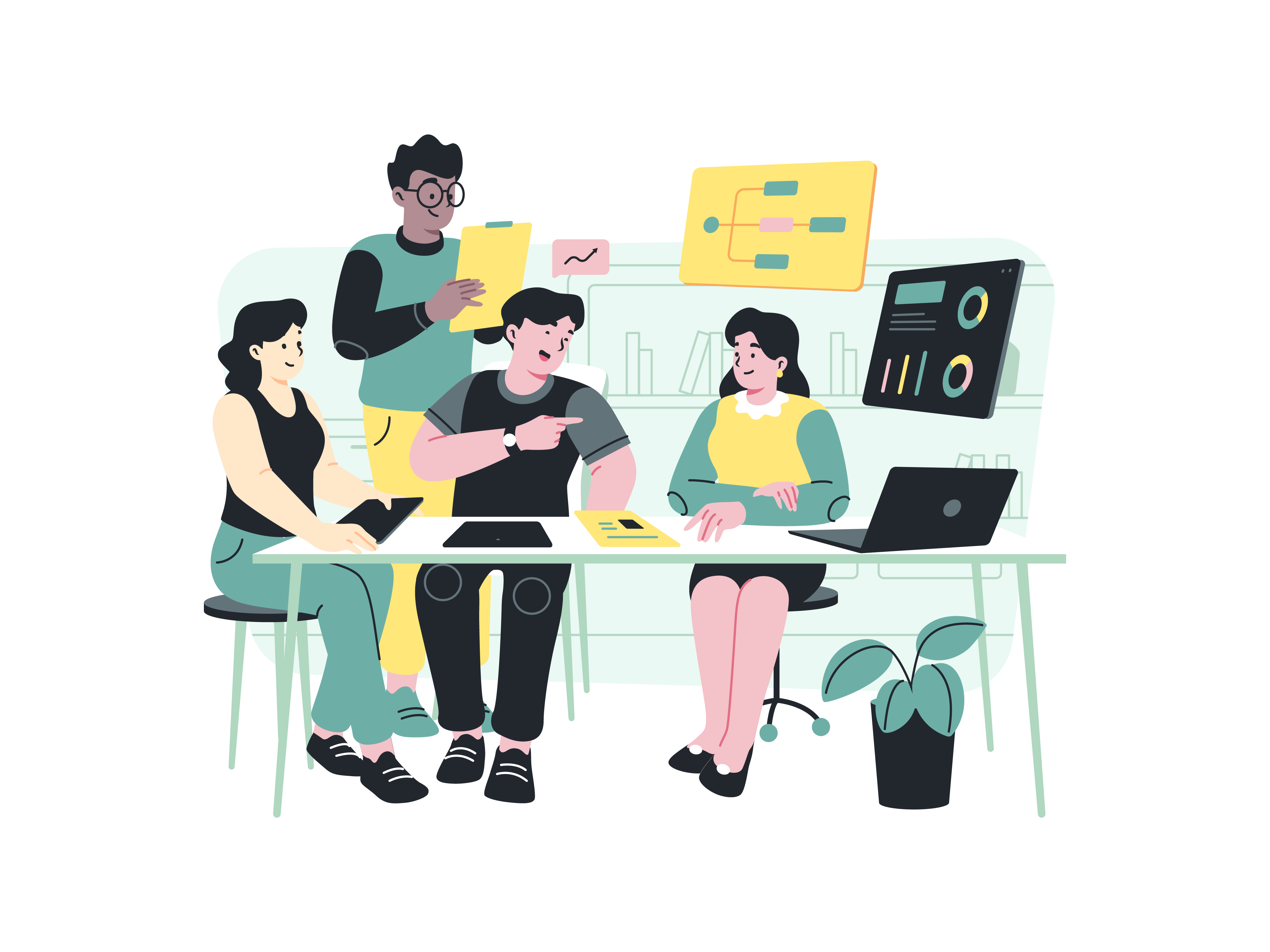
Introduction to CSS Optimization
In the world of web development, performance is paramount. One of the most significant factors affecting a website’s speed and efficiency is the size of its CSS files. Tailwind CSS, a utility-first CSS framework, addresses this challenge through its powerful purge mechanism. This comprehensive guide will dive deep into the purge option, exploring its functionality, implementation, and critical role in web performance optimization.
What is the Purge Option?
The purge option in Tailwind CSS is a sophisticated optimization technique designed to dramatically reduce the size of your CSS files by removing unused utility classes. In traditional CSS frameworks, developers often end up with massive stylesheet files containing thousands of utility classes, most of which are never used in the actual application. Tailwind’s purge feature solves this problem by intelligently scanning your project files and eliminating any CSS classes that aren’t actively used.
The Problem of Unused CSS
To understand the importance of the purge option, let’s first examine the typical CSS bloat problem:
- Large File Sizes: A standard Tailwind CSS file can be several hundred kilobytes in size.
- Unnecessary Network Overhead: Sending unused CSS to the browser wastes bandwidth.
- Increased Page Load Times: Larger CSS files take longer to download and parse.
How the Purge Option Works
The purge mechanism operates through a sophisticated process of class detection and elimination:
1. Source File Scanning
Tailwind scans specified files in your project, looking for any utility classes that are:
- Directly used in HTML
- Dynamically generated in JavaScript
- Referenced in template files
2. Class Extraction
During the build process, Tailwind:
- Identifies all unique classes used in your project
- Creates a whitelist of these classes
- Prepares to remove any classes not on this whitelist
3. CSS Optimization
The final step involves:
- Removing unused utility classes
- Generating a minimized CSS file
- Preserving only the classes actively used in your project
Configuration Examples
Basic Configuration
module.exports = {
purge: [
'./src/**/*.html',
'./src/**/*.js',
'./src/**/*.vue',
'./src/**/*.jsx',
],
// Other configuration options
};
This basic configuration tells Tailwind to scan all HTML, JavaScript, Vue, and JSX files in the src
directory and its subdirectories.
Advanced Configuration
module.exports = {
purge: {
content: [
'./src/**/*.html',
'./src/**/*.js',
'./src/**/*.vue',
'./components/**/*.vue'
],
// Optionally, specify classes to always keep
safelist: [
'bg-red-500',
'text-blue-600',
/^text-/, // Preserve all text-related classes
],
// Specify whether to run in development mode
enabled: process.env.NODE_ENV === 'production'
},
// Other configuration options
};
Handling Dynamic Classes
One common challenge with purge is handling dynamically generated classes. Tailwind provides several strategies:
1. Safelist Option
module.exports = {
purge: {
safelist: [
'bg-red-500',
'bg-blue-500',
/^text-/, // Preserves all text classes
]
}
};
2. Explicit Class Preservation
For classes generated dynamically:
// This ensures the class is preserved
const dynamicClass = `text-${color}-500`;
// Add a comment to explicitly mark for preservation
// tailwind-classes: text-red-500 text-blue-500 text-green-500
Performance Implications
Benchmarks and Improvements
Typical performance gains from purging include:
- File Size Reduction: 70-90% smaller CSS files
- Load Time Improvement: Faster initial page render
- Bandwidth Savings: Reduced data transfer
Real-World Example
Consider a typical Tailwind CSS file:
- Before Purge: 500 KB
- After Purge: 50-100 KB
Common Pitfalls and Solutions
1. Missed Classes
Problem: Some dynamically generated classes are accidentally removed. Solution: Use safelisting or explicit preservation techniques.
2. Development vs. Production
Tip: Typically, you want purge enabled only in production:
module.exports = {
purge: {
enabled: process.env.NODE_ENV === 'production',
content: [/* file paths */]
}
};
Best Practices
- Always Test: Verify your site looks correct after purging
- Use Version Control: Commit purge configuration changes
- Monitor Performance: Use browser dev tools to check CSS file size
- Consider Framework Specifics: Adjust configuration for React, Vue, or other frameworks
Future of CSS Optimization
Tailwind’s purge option represents a significant step in CSS optimization. As web applications become more complex, techniques like these will become increasingly important for maintaining performance.
Conclusion
The purge option in Tailwind CSS is more than just a configuration setting—it’s a powerful optimization technique that can significantly improve your web application’s performance. By intelligently removing unused CSS classes, you can create faster, more efficient websites with minimal effort.
Key Takeaways
- Purge dramatically reduces CSS file size
- Configuration is flexible and framework-agnostic
- Always test and verify after implementation
- Performance optimization is an ongoing process
Additional Resources
How does Next.js differ from traditional React applications
How does client-side rendering (CSR) work in Next.js
How does Next.js handle bundling and code splitting
What is incremental static regeneration in Next.js
Can a Next.js application use both server-side rendering and client-side rendering
How does Next.js handle SEO for dynamic pages
How can you implement custom 404 error handling for API routes