How does Next.js handle bundling and code splitting
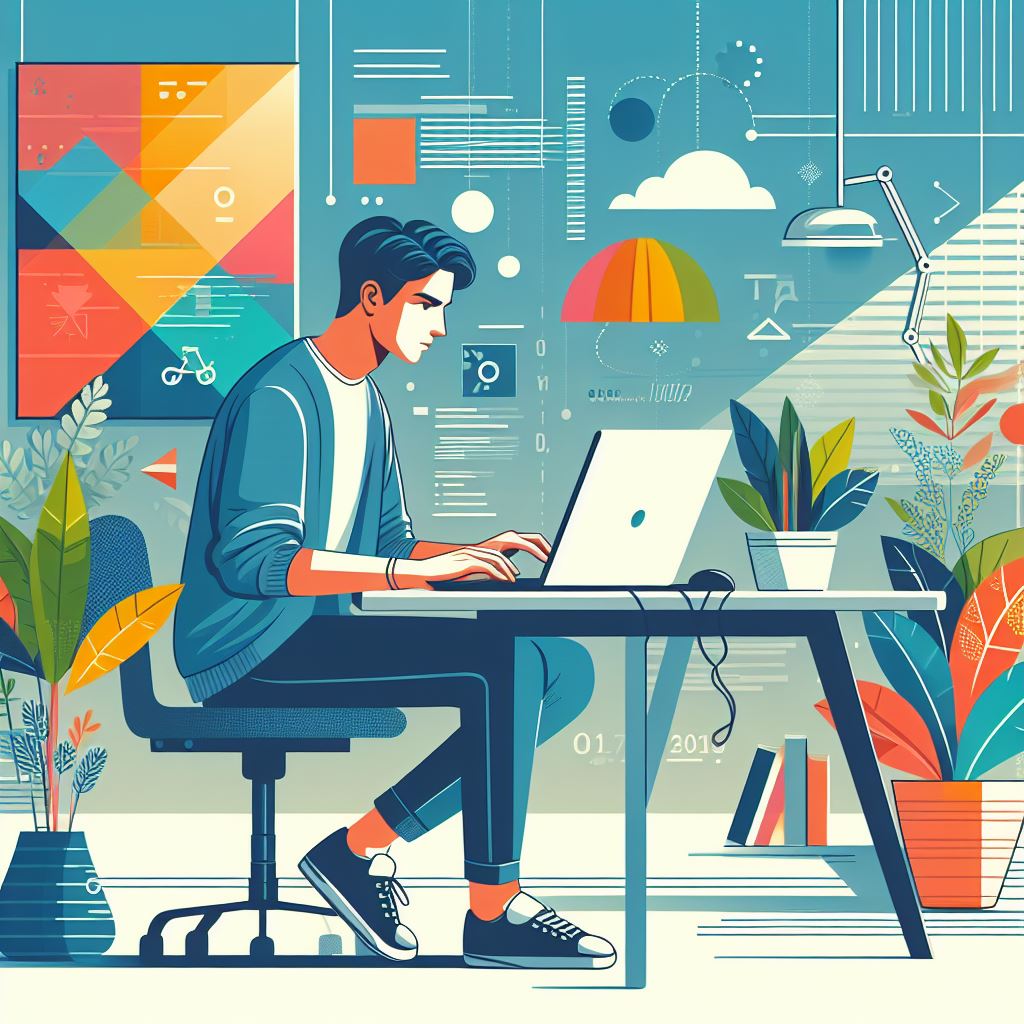
Efficient bundling and code splitting are paramount in modern web development, where optimizing performance and reducing load times play a crucial role. Next.js, a powerful React framework, has gained prominence for its ability to seamlessly handle bundling and code splitting, contributing to enhanced user experiences. In this article, we’ll delve into the inner workings of Next.js, unraveling how it manages bundling and code splitting to strike a balance between performance and functionality.
Understanding Bundling in Next.js
Bundling is the process of combining multiple JavaScript files into a single file, or bundle, for optimized delivery to the client’s browser. In traditional React applications, configuring bundling tools like Webpack is a common practice, involving manual setup and fine-tuning to ensure an efficient bundling process.
Next.js simplifies this by offering a zero-configuration approach. Out of the box, Next.js comes pre-configured with Webpack and Babel, eliminating the need for developers to delve into intricate configurations. This zero-configuration philosophy allows developers to focus on building features rather than spending time on setup, promoting a more streamlined development experience.
Automatic Code Splitting in Next.js
One of the standout features of Next.js is its automatic code splitting mechanism. Code splitting involves breaking down the JavaScript code into smaller, more manageable chunks that can be loaded on-demand. This ensures that users only download the code necessary for the specific page they are viewing, reducing the initial page load time and improving overall performance.
Next.js achieves automatic code splitting through its file-based routing system. The “pages” directory structure is central to this approach, where each file inside the “pages” directory corresponds to a route in the application. As a user navigates through the application, Next.js automatically generates bundles for each page, ensuring that only the relevant JavaScript is loaded.
getStaticProps and getServerSideProps for Efficient Data Fetching
Next.js introduces two functions, getStaticProps
and getServerSideProps
, to handle data fetching during the bundling process. These functions play a crucial role in optimizing the performance of the application by determining when and how data is fetched.
- getStaticProps: This function is used for static site generation (SSG). It allows developers to fetch data at build time, and the retrieved data is then injected into the page component during the bundling process. This results in pre-rendered HTML pages with embedded data, providing a faster and more efficient user experience.
- getServerSideProps: For scenarios requiring server-side rendering (SSR), this function fetches data on each request. While it may impact performance compared to SSG, it ensures that the content is always up-to-date, making it a suitable choice for dynamic or frequently changing data.
Dynamic Routing and Code Splitting
Next.js supports dynamic routing, a powerful feature that complements code splitting. Dynamic routes allow developers to create routes with parameters, facilitating the generation of pages based on user input or external data.
When combined with code splitting, dynamic routing enables the creation of optimized bundles for each dynamic route. This ensures that users only download the JavaScript code necessary for the specific dynamic page they are accessing, leading to a more efficient and responsive user experience.
The Importance of useEffect and Lazy Loading
In the realm of client-side rendering (CSR), the useEffect
hook becomes pivotal for managing asynchronous operations. This includes handling data fetching, side effects, and interactions after the initial rendering.
Next.js encourages the use of libraries like react-query
and swr
to efficiently manage data fetching. These libraries integrate seamlessly with the useEffect
hook, ensuring that data is loaded asynchronously, avoiding blocking the main thread and contributing to a smoother user experience.
Lazy loading is another technique employed by Next.js to enhance code splitting. With lazy loading, modules are loaded only when they are needed, reducing the initial payload and improving page load times. This is particularly beneficial for applications with large codebases, as it allows for more granular control over when and how modules are loaded.
Analyzing the Pros and Cons of Next.js Bundling and Code Splitting
As with any development approach, it’s crucial to weigh the pros and cons of Next.js bundling and code splitting to make informed decisions based on project requirements.
Pros
- Automatic Code Splitting: Next.js’s file-based routing system automates code splitting, ensuring that only the necessary JavaScript bundles are loaded for each page, optimizing performance.
- Efficient Data Fetching: The use of
getStaticProps
andgetServerSideProps
functions allows for efficient data fetching strategies, contributing to faster page loads and a smoother user experience. - Zero Configuration: Next.js’s zero-configuration approach simplifies bundling and code splitting, minimizing manual setup and allowing developers to focus on building features.
Cons
- Initial Page Load Time: While code splitting enhances subsequent page loads, the initial load time might still be impacted, especially in applications with extensive dependencies or large codebases.
- JavaScript Dependency: Code splitting relies heavily on JavaScript execution in the browser. Users may not get the best experience when JavaScript is disabled or has performance problems.
- Complexity in Dynamic Applications: Applications with complex dynamic routing and data dependencies may require careful consideration to optimize code splitting and maintain a responsive user experience.
Best Practices for Optimizing Bundling and Code Splitting in Next.js
To harness the full potential of Next.js bundling and code splitting, developers should adhere to some best practices;
- Optimize Data Fetching: Utilize
getStaticProps
andgetServerSideProps
judiciously to optimize data fetching based on the nature of the application and the required level of dynamism. - Dynamic Routing Considerations: When working with dynamic routes, carefully plan and structure the application to ensure efficient code splitting and optimal performance.
- Lazy Loading: Embrace lazy loading for components and modules that are not immediately required, allowing for more granular control over when code is loaded.
- Monitor Bundle Sizes: Keep a close eye on the size of JavaScript bundles, especially in larger applications. Use tools like Webpack Bundle Analyzer to identify and optimize large dependencies.
- Progressive Enhancement: Implement a progressive enhancement strategy to ensure a baseline experience for users with less capable browsers or disabled JavaScript.
Conclusion
Next.js’s approach to bundling and code splitting encapsulates the framework’s commitment to simplicity and performance. By automating code splitting through file-based routing, providing efficient data fetching strategies, and promoting lazy loading, Next.js empowers developers to create fast, responsive web applications with minimal configuration overhead.
As web development continues to evolve, the importance of optimizing bundling and code splitting cannot be overstated. In the dynamic landscape of user expectations and technological advancements, Next.js stands out as a tool that not only simplifies these complexities but also enhances the overall development experience. As developers delve into the intricacies of Next.js bundling and code splitting, they unlock the potential to create web applications that seamlessly balance functionality and performance, ensuring a delightful user experience in the digital realm.
How can I integrate Tailwind CSS with a CSS-in-JS solution like Emotion or Styled Components
What is the difference between the minified and unminified versions of Tailwind CSS
How do I install Tailwind CSS in my project
How do I integrate Tailwind CSS with a JavaScript framework like React or Vue.js
How do I create a responsive layout with Tailwind CSS
How do I vertically center an element in Tailwind CSS
How do I customize the spacing like padding and margin between elements in Tailwind CSS