How can you implement custom 404 error handling for API routes
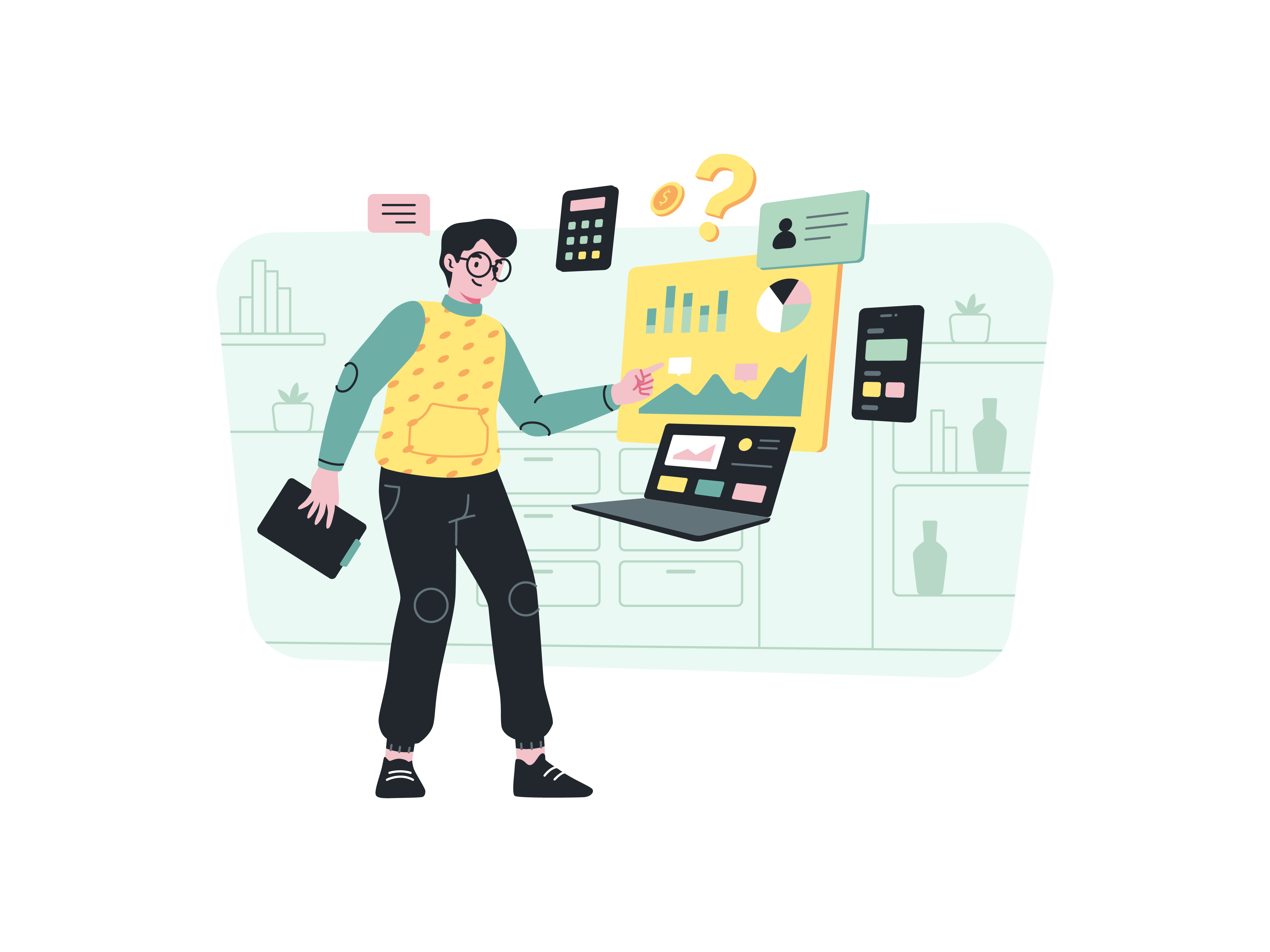
Error handling is a crucial aspect of web development, ensuring a smooth and user-friendly experience even when things go awry. In the context of API routes in Next.js, providing a custom 404 error page is essential for guiding users when they encounter non-existent endpoints or unexpected errors. In this article, we’ll explore the steps and best practices for implementing custom 404 error handling for API routes in Next.js, enhancing both functionality and user satisfaction.
Understanding API Routes in Next.js
API routes in Next.js are special files located in the pages/api
directory that define serverless functions. These functions handle incoming HTTP requests and can be utilized for various purposes, such as fetching data, processing forms, or interacting with a database. Ensuring proper error handling within these routes contributes to a polished and reliable application.
The Default 404 Page
Next.js provides a default 404 error page that is automatically rendered when a route is not found. However, for API routes, the default behavior may not align with the desired user experience. Implementing a custom 404 error handling mechanism allows developers to tailor the response and provide more informative and user-friendly messages.
Steps to Implement Custom 404 Error Handling
1. Create a Custom 404 API Route
- Begin by creating a custom 404 API route in the
pages/api
directory. For example, create a file namednotFound.js
.
// pages/api/notFound.js
export default (req, res) => {
res.status(404).json({ error: 'Not Found' });
};
2. Modify API Routes to Redirect to Custom 404 Route
- In each API route that requires custom error handling, redirect to the custom 404 route when the necessary conditions are met. For instance, if a specific resource is not found, redirect to the
notFound
route.
// pages/api/specificRoute.js
export default (req, res) => {
const resource = /* logic to fetch or process resource */;
if (!resource) {
return res.redirect(307, '/api/notFound');
}
// Process the request and send a response
res.json({ result: 'Success', data: resource });
};
3. Handle Different Error Scenarios
- Customize the
notFound
API route to handle different error scenarios based on the specific needs of your application. This may include providing additional details, logging errors, or redirecting users to a more relevant page.
// pages/api/notFound.js
export default (req, res) => {
const { error } = req.query;
switch (error) {
case 'resourceNotFound':
return res.status(404).json({ errorMessage: 'Resource not found' });
case 'invalidRequest':
return res.status(400).json({ errorMessage: 'Invalid request' });
// Add more cases as needed
default:
return res.status(404).json({ errorMessage: 'Not Found' });
}
};
Best Practices for Custom 404 Error Handling
Provide Clear and Informative Messages
- Craft error messages that clearly communicate the nature of the issue. Users should be able to understand why the error occurred and how to proceed.
Log Errors for Debugging
- Implement logging mechanisms within custom error handling routes to capture details of the errors. This information can be invaluable for debugging and improving the application.
Redirect with Relevant HTTP Status Codes
- Use appropriate HTTP status codes when redirecting to the custom 404 route. For instance, a
307 Temporary Redirect
indicates that the requested resource is temporarily unavailable.
Consider Internationalization
- If your application supports multiple languages, consider implementing internationalized error messages to cater to users from different linguistic backgrounds.
Test Edge Cases
- Thoroughly test the custom error handling mechanisms by simulating various scenarios, including resource not found, invalid requests, and unexpected errors. Ensure that the responses align with the expected behavior.
Integrate with Front-End Error Handling
- Coordinate custom error handling on the API routes with error handling mechanisms on the front end. This ensures a cohesive and consistent user experience in the face of errors.
Conclusion
Implementing custom 404 error handling for API routes in Next.js is a crucial step toward providing a seamless and user-friendly web application. By tailoring error responses to specific scenarios, developers can guide users effectively and enhance the overall experience. Whether redirecting users to a custom 404 page or providing detailed error messages, the flexibility offered by Next.js empowers developers to create resilient and user-centric applications. By following best practices and considering various error scenarios, developers can ensure that their custom 404 error handling mechanisms align with the expectations of both users and stakeholders, contributing to a more robust and reliable web application.
How do you implement server-side rendering with React and Node.js
How do you implement server-side rendering with React and Express
How does Next.js differ from traditional React applications
How does client-side rendering (CSR) work in Next.js
How does Next.js handle bundling and code splitting
What is incremental static regeneration in Next.js
Can a Next.js application use both server-side rendering and client-side rendering