How does client-side rendering (CSR) work in Next.js
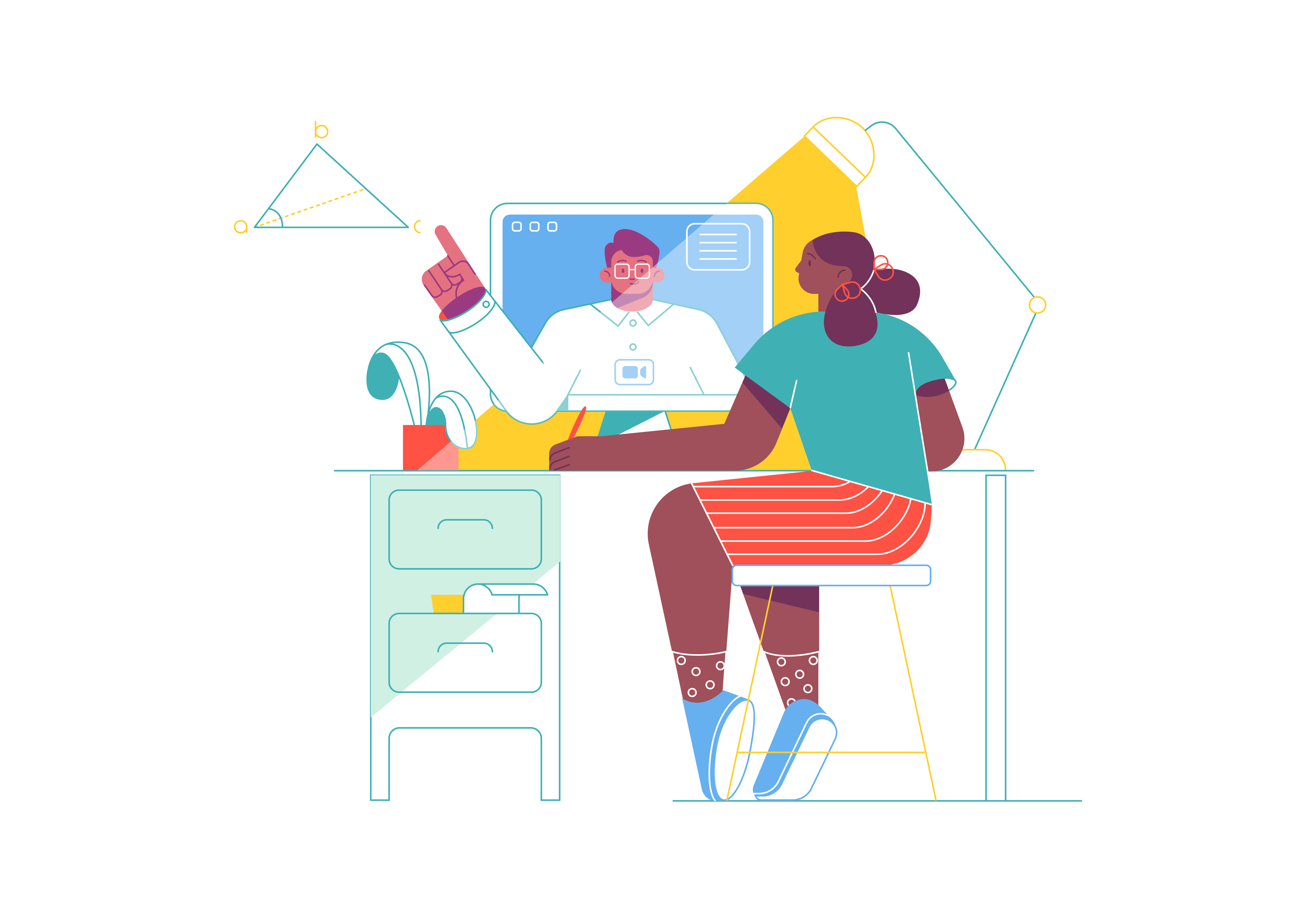
Client-side rendering (CSR) is a fundamental concept in modern web development, offering dynamic and interactive user experiences. In the realm of frameworks, Next.js has emerged as a powerful tool that seamlessly integrates CSR into its architecture. In this article, we’ll embark on a journey to unravel the mechanics of client-side rendering in Next.js, exploring its intricacies and understanding how it enhances the development of robust and responsive web applications.
Understanding Client-Side Rendering (CSR)
Before diving into how Next.js implements CSR, let’s grasp the essence of client-side rendering itself. In a nutshell, CSR involves shifting the responsibility of rendering web pages from the server to the client’s browser. This allows for a more dynamic and interactive user experience, as the browser can render and update content without requiring a full page reload.
Traditionally, server-side rendering (SSR) was the dominant approach, where the server generated the HTML for each request. While SSR is effective for improving initial page load times and SEO, CSR excels in providing a smoother, more responsive feel by offloading rendering tasks to the client.
The Next.js Approach to CSR
Next.js provides a hybrid model, allowing developers to choose between client-side rendering, server-side rendering, or a combination of both based on project requirements. To implement CSR in Next.js, the framework leverages React’s capabilities while introducing some key features to optimize the process.
File-Based Routing
A distinctive feature of Next.js is its file-based routing system, which simplifies the organization of pages. The “pages” directory structure is central to this approach. When using CSR, each file in the “pages” directory becomes a route, and the corresponding React component is rendered on the client side when navigating to that route.
This file-based routing not only enhances project structure but also facilitates automatic code splitting, ensuring that only the necessary JavaScript bundles are loaded for a given page, optimizing performance.
getStaticProps and getServerSideProps
Next.js introduces two functions, getStaticProps and getServerSideProps, to handle data fetching during the CSR process.
getStaticProps: Used for static site generation (SSG), this function allows developers to fetch data at build time. The retrieved data is then injected into the page component during the build process, resulting in pre-rendered HTML for improved performance.
getServerSideProps: For scenarios requiring server-side rendering, this function fetches data on each request, ensuring that the content is always up-to-date. While it may impact performance compared to SSG, it offers real-time data updates.
These functions provide flexibility in choosing the most suitable data fetching strategy based on the project’s requirements.
useEffect and Data Fetching
In the context of CSR, the useEffect hook becomes crucial for managing asynchronous operations. When a component is mounted on the client side, useEffect can be employed to trigger data fetching and handle side effects.
Next.js encourages developers to use libraries like swr (pronounced “swear”) for efficient data fetching and caching. This helps in avoiding redundant network requests and ensures a smoother user experience by providing up-to-date data when rendering on the client side.
Dynamic Routing
Next.js supports dynamic routing, allowing for the creation of routes with dynamic parameters. This is particularly useful when working with data that depends on user input or external sources.
With dynamic routing, pages can be generated on the client side based on dynamic parameters, enhancing the flexibility and interactivity of web applications.
Pros and Cons of CSR in Next.js
As with any architectural decision, it’s essential to weigh the pros and cons of implementing CSR in Next.js.
Pros
Improved User Experience: CSR enables a more responsive and dynamic user interface, as content updates can be handled on the client side without full page reloads.
Flexibility in Data Fetching: Next.js provides versatile options for data fetching, allowing developers to choose between static site generation and server-side rendering based on project requirements.
Efficient Code Splitting: Automatic code splitting ensures that only the necessary JavaScript bundles are loaded for a given page, optimizing performance and reducing initial load times.
Cons
Initial Page Load Time: While CSR excels in providing an interactive user experience, the initial page load time might be affected, especially when compared to static site generation.
SEO Concerns: Although Next.js mitigates SEO concerns through server-side rendering and hybrid approaches, CSR alone may not be as favorable for SEO as static site generation.
JavaScript Dependency: CSR relies heavily on JavaScript execution in the browser. In scenarios where JavaScript is disabled or faces performance issues, the user experience may be compromised.
Best Practices for Implementing CSR in Next.js
To harness the full potential of CSR in Next.js, developers should adhere to some best practices;
Efficient Data Fetching: Optimize data fetching using appropriate Next.js functions like getStaticProps and getServerSideProps and consider libraries like swr for effective data management.
Dynamic Routing: Leverage dynamic routing for enhanced user interactivity, especially when dealing with user-specific or external data.
Code Splitting: Leverage Next.js’s automatic code splitting to ensure that only essential JavaScript bundles are loaded for each page, enhancing performance.
SEO Considerations: If SEO is a priority, consider combining CSR with server-side rendering or static site generation for optimal search engine visibility.
Progressive Enhancement: Implement CSR as part of a progressive enhancement strategy, ensuring a baseline experience for users with less capable browsers or disabled JavaScript.
Conclusion
Client-side rendering in Next.js opens up a world of possibilities for developers seeking to build dynamic, interactive web applications. The framework’s flexibility, combined with its file-based routing system and efficient data fetching strategies, allows for a tailored approach to CSR. While it’s essential to weigh the pros and cons and consider best practices, Next.js empowers developers to strike a balance between performance and interactivity, providing a solid foundation for crafting modern web experiences. As web development continues to evolve, understanding the mechanics of client-side rendering in Next.js becomes increasingly crucial for developers aiming to stay at the forefront of this dynamic field.
How does React handle context switching
How do you pass data between parent and child components in React
What is the difference between functional components and class components
How do you handle authentication in a React-Redux application
How does React handle dynamic imports
How do you handle code splitting with React Router
Explain the concept of lazy loading in React
What is the significance of the forwardRef function in React
What is the significance of the react-router-dom library
How do you implement server-side rendering with React and Node.js
How do you implement server-side rendering with React and Express