What is the significance of the _document.js file in a Next.js project
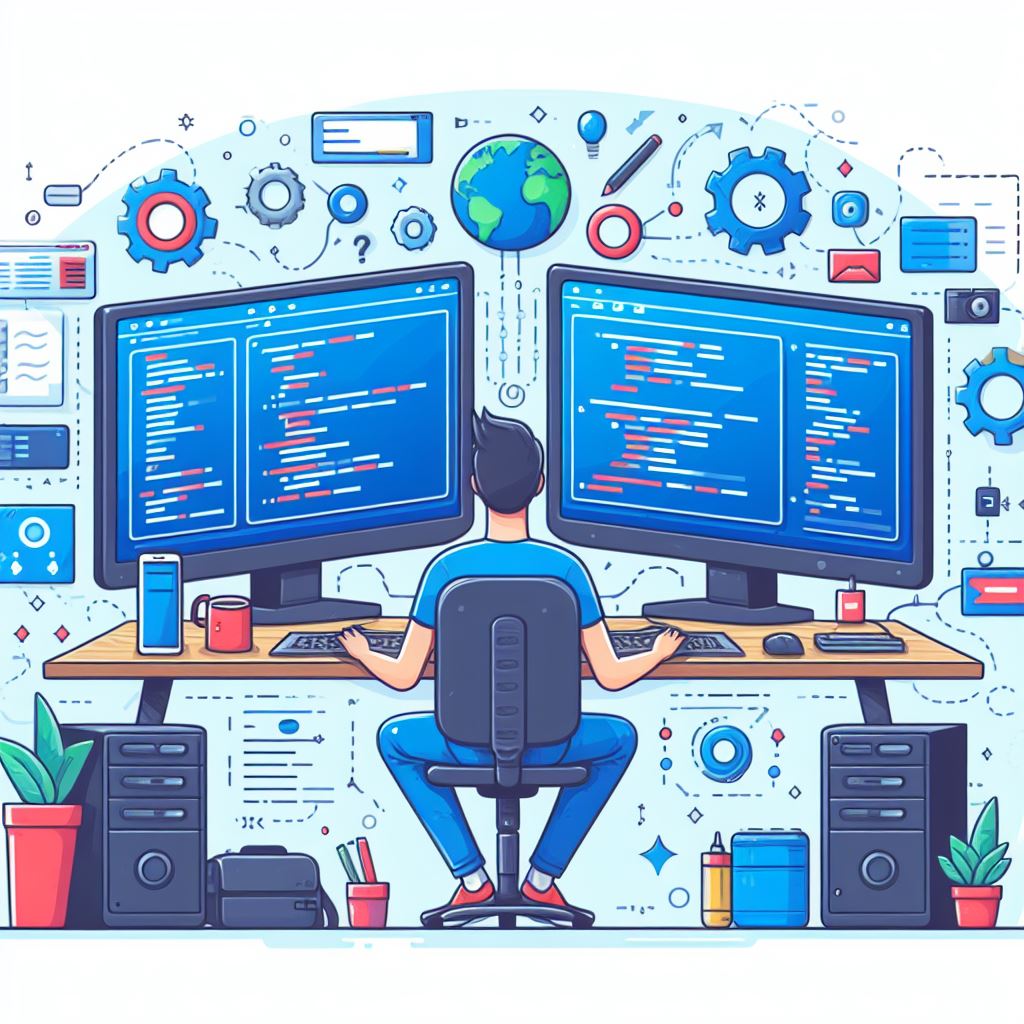
In the realm of Next.js development, understanding every aspect of the project structure is crucial for harnessing its full potential. Among the various files and configurations, the _document.js
file holds a special significance. In this comprehensive guide, we’ll unravel the mysteries surrounding the _document.js
file, exploring its purpose, functionalities, and how it contributes to building robust and customized Next.js applications.
Introduction to the _document.js
File
The _document.js
file plays a vital role in customizing the HTML document structure generated by Next.js. While most of the application’s layout and UI components are rendered dynamically on the client-side, certain elements, such as the HTML structure, <head>
contents, and initial state management, require server-side rendering (SSR) for optimal performance and SEO.
The _document.js
file provides developers with a centralized location to define these server-side rendering configurations, allowing for fine-grained control over the application’s HTML document.
Understanding the Purpose
Customizing HTML Structure
One of the primary purposes of the _document.js
file is to customize the HTML structure rendered by Next.js. By extending the default Document
class provided by Next.js, developers can override methods like render()
to inject custom markup into the HTML document.
Managing External Resources
The _document.js
file is also instrumental in managing external resources such as stylesheets, scripts, and meta tags. Developers can specify global stylesheets or scripts to be included in every page of the application, ensuring consistency and optimal performance.
Initial State Management
Another significant aspect of the _document.js
file is its role in managing the initial state of the application. By serializing the initial state into the HTML document, developers can prepopulate client-side state management libraries like Redux or Zustand, reducing the time-to-interactivity and enhancing user experience.
Implementing Customization
Let’s explore how to implement customization using the _document.js
file:
// pages/_document.js
import Document, { Html, Head, Main, NextScript } from 'next/document';
class MyDocument extends Document {
static async getInitialProps(ctx) {
const initialProps = await Document.getInitialProps(ctx);
return { ...initialProps };
}
render() {
return (
<Html lang="en">
<Head>
{/* Custom meta tags, stylesheets, and scripts */}
<meta name="description" content="Next.js Custom Document Example" />
<link rel="stylesheet" href="/styles/global.css" />
</Head>
<body>
<Main />
<NextScript />
</body>
</Html>
);
}
}
export default MyDocument;
In this example, we extend the Document
class and override the render()
method to customize the HTML document structure. We add custom meta tags, include a global stylesheet, and render the Main
component along with NextScript
for client-side hydration.
Best Practices and Considerations
- Keep It Lightweight: Avoid adding unnecessary markup or heavy scripts to the
_document.js
file, as it can impact initial page load times. - Use External Resources Wisely: Optimize the inclusion of external resources to minimize HTTP requests and maximize performance.
- Test Across Environments: Ensure compatibility and consistency by testing the customized document across different environments and browsers.
Conclusion
The _document.js
file in a Next.js project serves as a powerful tool for customizing the HTML document structure, managing external resources, and initializing client-side state. By leveraging its capabilities, developers can enhance SEO, improve performance, and create a consistent and tailored user experience. Understanding the significance of the _document.js
file is essential for mastering Next.js development and building high-quality, professional-grade applications.
How does Next.js handle static assets like images, fonts, and CSS files
How can you implement a custom loading indicator for dynamic routes
How can you create dynamic routes in Next.js
What is the purpose of the next/image component in Next.js
What is the purpose of the next.config.js file
How can you optimize and serve web fonts efficiently in a Next.js project
Explain the significance of the Link component’s replace prop in Next.js
How can you handle form submissions in a Next.js application
What are the best practices for structuring a Next.js project
What is the significance of the api folder in a Next.js project