How can you implement a custom loading indicator for dynamic routes
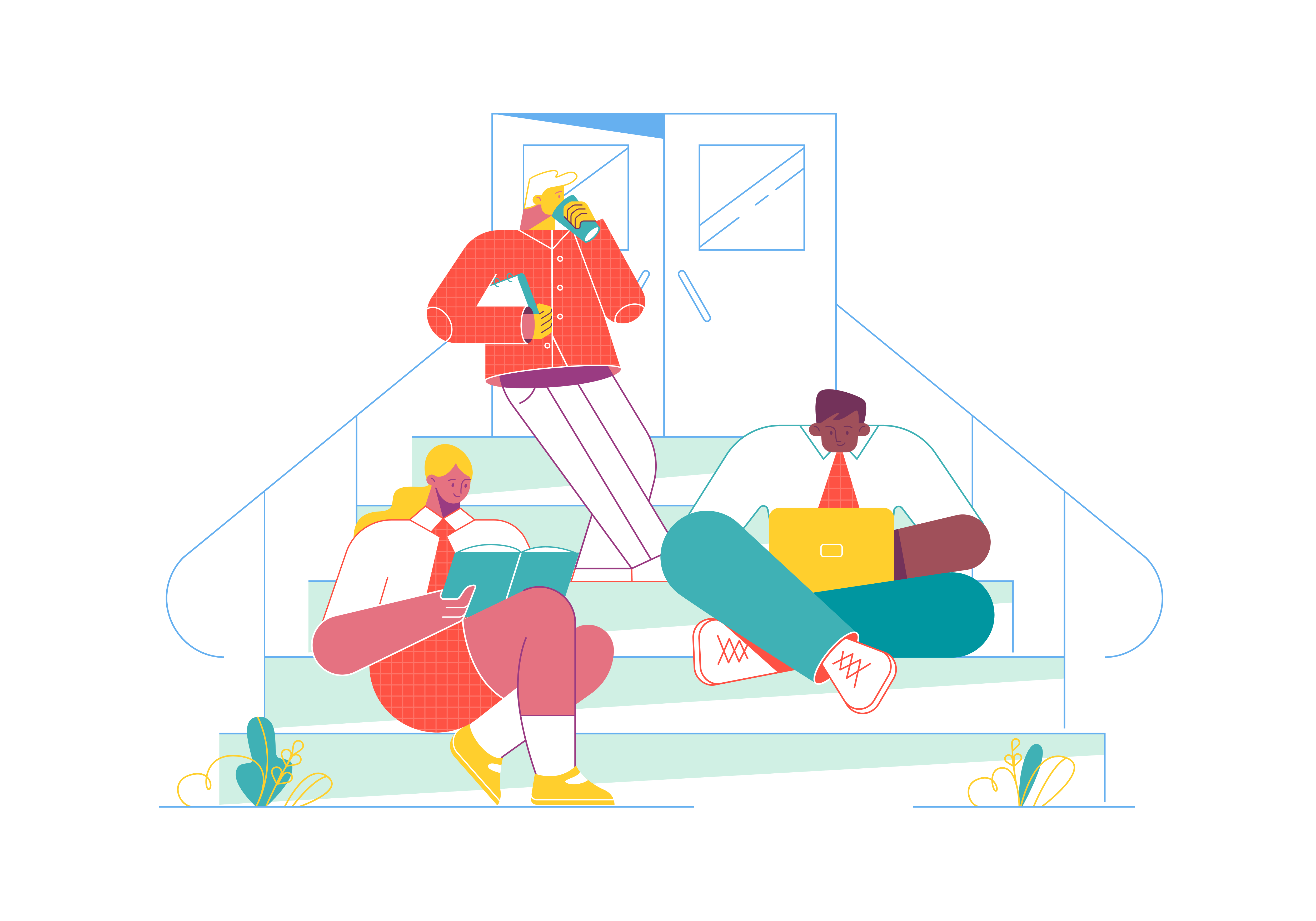
Dynamic routes in Next.js offer powerful capabilities for building dynamic and data-driven web applications. However, ensuring a smooth and seamless user experience during page transitions can be challenging, especially when fetching data asynchronously. Implementing a custom loading indicator for dynamic routes is essential for providing users with visual feedback and enhancing perceived performance. In this comprehensive guide, we’ll explore how you can implement a custom loading indicator for dynamic routes in Next.js, covering various techniques, best practices, and examples.
Understanding Dynamic Routes in Next.js
Before we dive into implementing a custom loading indicator, let’s briefly review dynamic routes in Next.js:
- Dynamic Routing: Next.js allows developers to create dynamic routes by defining file-based routes with parameters enclosed in square brackets (e.g.,
[id].js
). These parameters can be used to fetch data dynamically based on the requested route. - Data Fetching: Dynamic routes often involve fetching data asynchronously from external APIs, databases, or other sources. Next.js provides built-in functions like
getStaticProps
andgetServerSideProps
to fetch data at build time or request time, respectively. - Client-Side Navigation: Next.js enables client-side navigation using the
Link
component from thenext/link
module. When navigating between dynamic routes, Next.js automatically prefetches the required data for improved performance.
Implementing a Custom Loading Indicator
To implement a custom loading indicator for dynamic routes in Next.js, follow these steps:
1. Create a Loading Component
First, create a custom loading component that will be displayed during page transitions. This component can include a loading spinner, progress bar, or any other visual indicator to indicate that data is being fetched.
// components/LoadingIndicator.js
import React from 'react';
const LoadingIndicator = () => {
return <div className="loading-indicator">Loading...</div>;
};
export default LoadingIndicator;
2. Handle Loading State
Next, manage the loading state of dynamic routes using the useRouter
hook from next/router
. Update the loading state when navigating between routes and display the custom loading indicator accordingly.
// pages/[id].js
import { useRouter } from 'next/router';
import LoadingIndicator from '../components/LoadingIndicator';
const DynamicRoutePage = () => {
const router = useRouter();
const { isLoading } = router;
return (
<div>
{isLoading ? <LoadingIndicator /> : <h1>Dynamic Route Page</h1>}
</div>
);
};
export default DynamicRoutePage;
3. Customize Loading Indicator Styling
Style the custom loading indicator to match the design and branding of your application. You can use CSS, CSS-in-JS libraries like styled-components, or CSS frameworks like Tailwind CSS to customize the appearance of the loading indicator.
/* styles/loading-indicator.css */
.loading-indicator {
display: flex;
justify-content: center;
align-items: center;
height: 50px;
font-size: 1.5rem;
color: #333;
}
4. Optional: Add Transitions
For a smoother user experience, consider adding transitions or animations to the loading indicator to provide visual feedback during page transitions. CSS transitions or animation libraries like framer-motion can be used to achieve this effect.
// components/LoadingIndicator.js
import React from 'react';
import { motion } from 'framer-motion';
const LoadingIndicator = () => {
return (
<motion.div
className="loading-indicator"
initial={{ opacity: 0 }}
animate={{ opacity: 1 }}
exit={{ opacity: 0 }}
>
Loading...
</motion.div>
);
};
export default LoadingIndicator;
Best Practices and Considerations
- Accessibility: Ensure that the custom loading indicator is accessible to all users, including those using assistive technologies. Provide alternative text or ARIA attributes for screen readers to convey the loading status.
- Performance: Keep the loading indicator lightweight and performant to minimize its impact on page load times. Avoid heavy animations or unnecessary JavaScript that could delay rendering.
- Progressive Enhancement: Implement the loading indicator as a progressive enhancement, ensuring that the page remains functional even if JavaScript is disabled or the indicator fails to load.
- Testing: Test the loading indicator across different devices, screen sizes, and network conditions to ensure consistent behavior and performance.
Conclusion
Implementing a custom loading indicator for dynamic routes in Next.js is essential for providing users with visual feedback and enhancing perceived performance during page transitions. By creating a custom loading component, managing the loading state, customizing the styling, and optionally adding transitions, developers can create a seamless and engaging user experience for dynamic routes in Next.js applications. Embrace best practices, prioritize accessibility and performance, and test thoroughly to ensure that the loading indicator enhances the overall user experience and contributes to the success of your Next.js project.
How can you implement a loading spinner for data fetching in Next.js
How can you handle state management in a Next.js application
How does data fetching work in Next.js
How does Next.js handle state persistence between page navigations
How does Next.js handle routing
What is the purpose of the pages directory in a Next.js project
What is the purpose of the next.config.js option env
What are the considerations for securing a Next.js application
How can you implement A/B testing in a Next.js application
What is the purpose of the disableStaticImages option in next.config.js