What is the purpose of the next/image component in Next.js
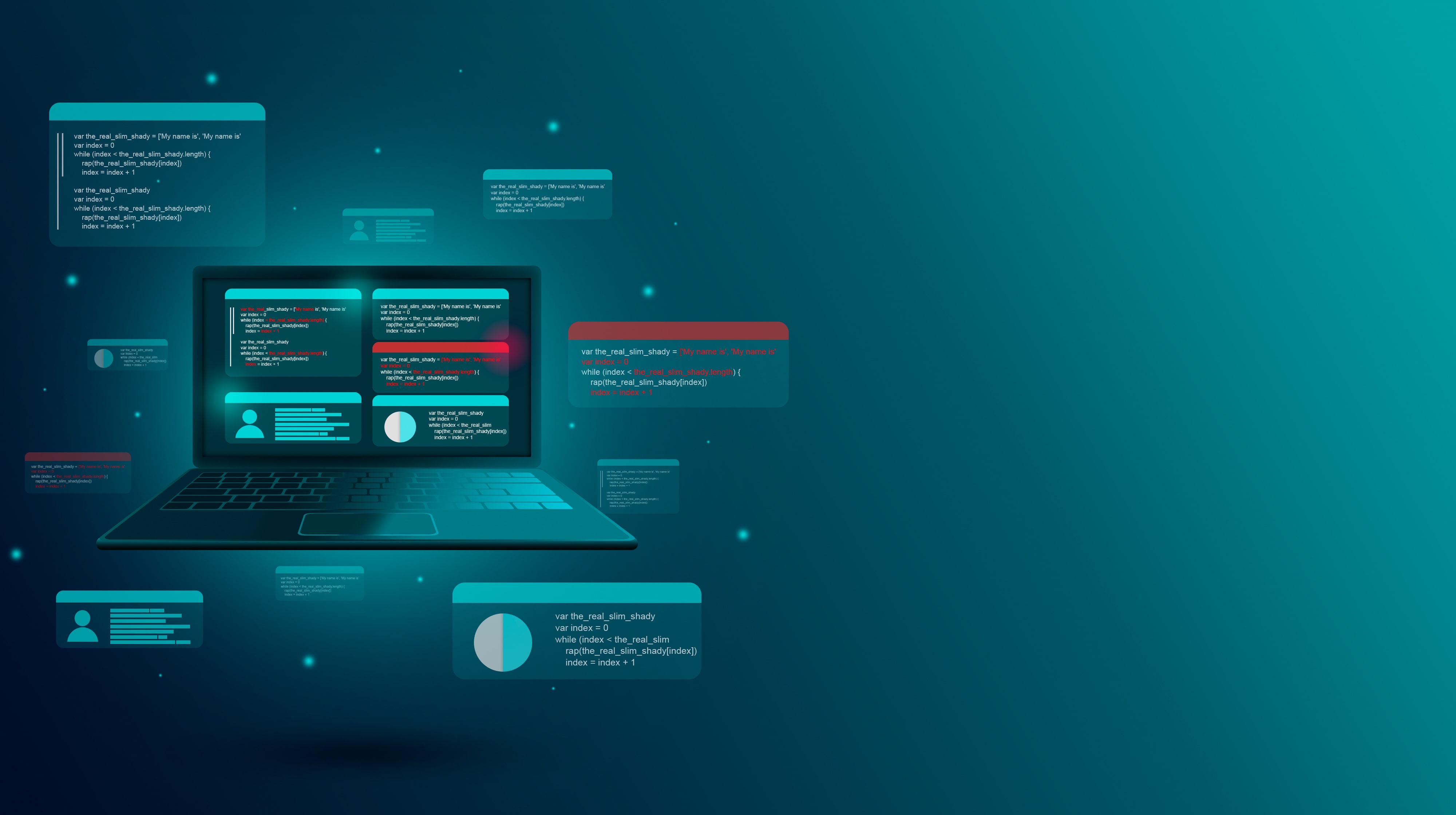
The next/image
component is a powerful addition to Next.js that provides optimized image loading and handling out of the box. In this comprehensive guide, we’ll delve into the purpose of the next/image
component, its features, benefits, and best practices for leveraging it effectively in your Next.js applications.
Introduction to the next/image
Component
Images are a fundamental part of web development, but they can also pose challenges in terms of performance, accessibility, and responsiveness. The next/image
component addresses these challenges by offering a streamlined solution for image optimization, lazy loading, and responsive image loading in Next.js applications.
Key Features of the next/image
Component
Let’s explore some of the key features and capabilities of the next/image
component:
1. Automatic Image Optimization
One of the primary features of the next/image
component is its built-in support for automatic image optimization. When you use the next/image
component to display images in your Next.js application, Next.js automatically optimizes the images based on factors such as device size, screen resolution, and network conditions. This optimization process includes resizing, compressing, and serving images in modern formats like WebP to ensure optimal performance and user experience.
2. Lazy Loading
The next/image
component implements lazy loading by default, which means that images are only loaded when they enter the viewport. This lazy loading behavior helps reduce initial page load times and bandwidth consumption, particularly for pages with multiple images or large image assets.
3. Responsive Image Loading
Next.js provides built-in support for responsive image loading through the next/image
component. You can specify multiple image sizes and breakpoints using the srcSet
attribute, allowing the browser to choose the most appropriate image based on the device’s screen size and resolution. This responsive image loading technique ensures that users receive an optimized image tailored to their device’s capabilities.
4. Accessibility
The next/image
component prioritizes accessibility by generating optimized HTML markup with appropriate alt text for screen readers. This ensures that images are accessible to users with disabilities and comply with web accessibility standards, improving the overall accessibility and inclusivity of your Next.js application.
Benefits of Using the next/image
Component
By leveraging the next/image
component in your Next.js applications, you can reap several benefits:
- Improved Performance: Automatic image optimization and lazy loading help improve page load times and performance, particularly on devices with limited bandwidth or slower network connections.
- Enhanced User Experience: Responsive image loading ensures that users receive high-quality images tailored to their device’s screen size and resolution, enhancing the overall user experience and visual appeal of your application.
- Accessibility Compliance: The
next/image
component generates accessible HTML markup with appropriate alt text, ensuring compliance with web accessibility standards and making your application more inclusive for users with disabilities.
How to Use the next/image
Component
Using the next/image
component is straightforward. Simply import the Image
component from next/image
and specify the src
attribute to the image URL:
import Image from 'next/image';
const MyImage = () => {
return <Image src="/image.jpg" alt="Description of image" width={500} height={300} />;
};
export default MyImage;
You can also customize additional attributes such as layout
, objectFit
, and priority
to further optimize image loading and display behavior.
Best Practices for Using the next/image
Component
To maximize the benefits of the next/image
component, consider the following best practices:
- Optimize Image Assets: Before using images in your Next.js application, optimize them for web delivery by resizing, compressing, and converting them to modern formats like WebP.
- Specify Dimensions: Always specify the
width
andheight
attributes for thenext/image
component to prevent layout shifts and ensure that the browser can reserve the appropriate space for the image. - Use Responsive Image Loading: Take advantage of responsive image loading by specifying multiple sizes and breakpoints using the
srcSet
attribute to ensure that images are appropriately sized for different devices and screen resolutions. - Provide Alt Text: Always provide descriptive alt text for images using the
alt
attribute to ensure accessibility and improve search engine optimization (SEO). - Preload Critical Images: Use the
priority
attribute to preload critical images that are essential for the initial rendering of the page, such as hero images or above-the-fold content.
Conclusion
The next/image
component in Next.js offers a powerful solution for image optimization, lazy loading, and responsive image loading in web applications. By leveraging its features and benefits, you can enhance the performance, accessibility, and user experience of your Next.js applications while simplifying image handling and management. Embrace best practices, optimize your image assets, and leverage the next/image
component to create fast, efficient, and visually appealing web experiences for your users.
How does Next.js handle state persistence between page navigations
How does Next.js handle routing
What is the purpose of the pages directory in a Next.js project
What is the purpose of the next.config.js option env
What are the considerations for securing a Next.js application
How can you implement A/B testing in a Next.js application
What is the purpose of the disableStaticImages option in next.config.js
How does Next.js handle static assets like images, fonts, and CSS files
How can you implement a custom loading indicator for dynamic routes