What is the purpose of the next.config.js file
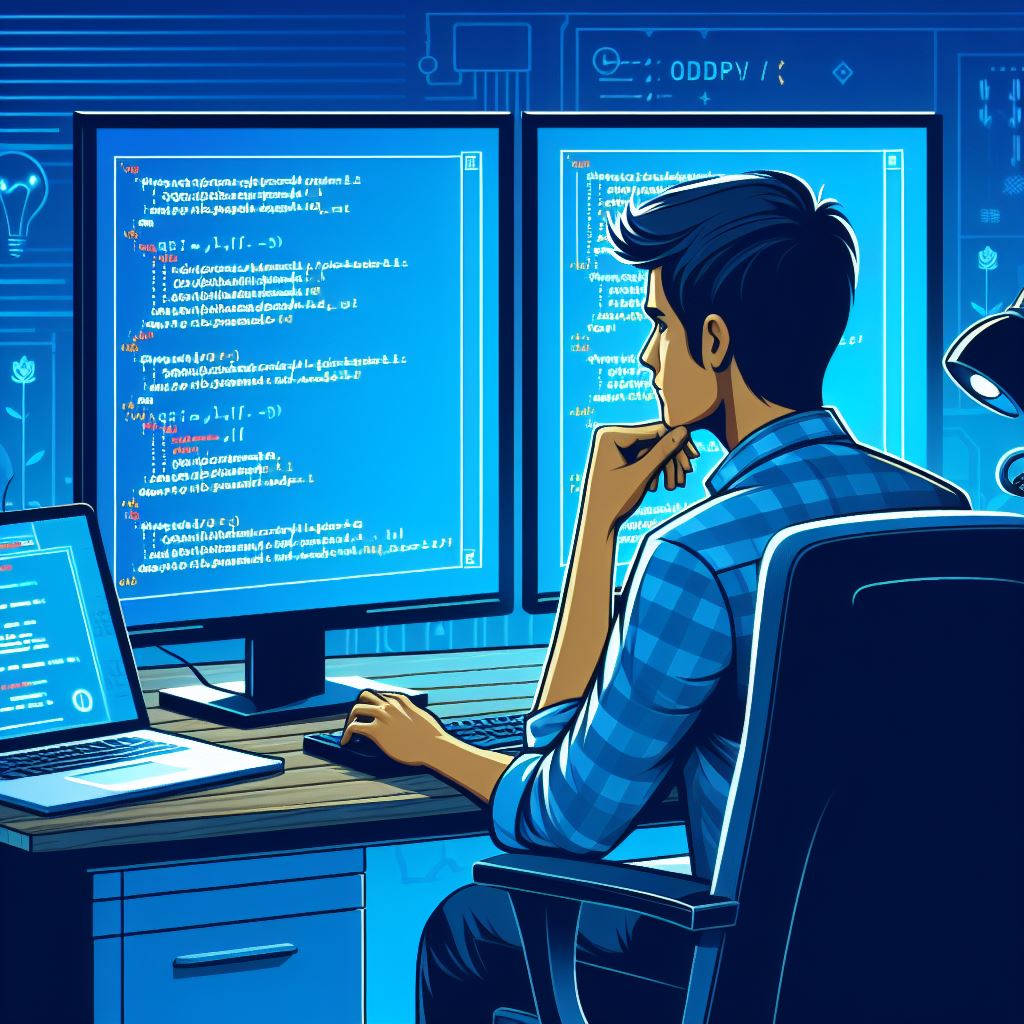
The next.config.js
file is a powerful configuration file in Next.js that allows developers to customize various aspects of their Next.js applications. In this comprehensive guide, we’ll delve into the purpose of the next.config.js
file, its features, common configurations, and best practices for leveraging it effectively in your Next.js projects.
Introduction to the next.config.js
File
The next.config.js
file serves as the configuration file for Next.js applications, allowing developers to modify default settings, add custom configurations, and extend the functionality of Next.js. It provides a centralized location for configuring features such as asset optimization, webpack customization, environment variables, and more.
Key Features of the next.config.js
File
Let’s explore some of the key features and capabilities of the next.config.js
file:
1. Custom Webpack Configuration
Next.js uses webpack under the hood to bundle and optimize assets for the browser. The next.config.js
file allows developers to customize webpack configurations using the webpack
property. This enables advanced customization such as adding loaders, plugins, aliases, and other optimizations to tailor webpack behavior to specific project requirements.
// next.config.js
module.exports = {
webpack: (config, { isServer }) => {
// Add custom webpack configurations
return config;
},
};
2. Environment Variables
The next.config.js
file provides a convenient way to define environment variables for your Next.js application. Environment variables can be accessed at build time or runtime using the process.env
object. This is useful for managing sensitive data, configuration values, API keys, and other environment-specific settings.
// next.config.js
module.exports = {
env: {
API_URL: process.env.API_URL,
},
};
3. Asset Optimization
Next.js offers built-in support for optimizing assets such as images, fonts, and CSS files. The next.config.js
file allows developers to configure asset optimization settings using the images
, fonts
, and experimental
properties. This includes features like automatic image optimization, font preloading, CSS optimization, and more.
// next.config.js
module.exports = {
images: {
domains: ['example.com'],
},
};
4. Routing Configuration
Next.js uses a file-based routing system by default, where each file in the pages
directory corresponds to a route in the application. The next.config.js
file enables developers to customize routing behavior using the exportPathMap
function. This allows for dynamic route generation, custom route handling, and more advanced routing configurations.
// next.config.js
module.exports = {
exportPathMap: async function () {
return {
'/': { page: '/' },
'/about': { page: '/about' },
};
},
};
Common Configurations in next.config.js
Here are some common configurations that developers often include in their next.config.js
files:
- Custom Webpack Plugins: Adding custom webpack plugins for tasks such as code splitting, bundle analysis, and optimization.
- CSS Preprocessing: Configuring CSS preprocessing libraries like Sass, Less, or PostCSS for styling.
- Bundle Size Optimization: Implementing strategies to reduce bundle size through code splitting, tree shaking, and other optimization techniques.
- Environment-specific Configuration: Managing environment-specific configuration values for development, staging, and production environments.
- Asset Handling: Configuring asset optimization settings for images, fonts, and other static assets.
- Middleware: Adding middleware functions for server-side rendering (SSR), API routes, authentication, and other server-side logic.
Best Practices for Using the next.config.js
File
To make the most of the next.config.js
file, consider the following best practices:
- Keep It Organized: Maintain a clean and organized
next.config.js
file by grouping related configurations and separating concerns. - Document Configuration: Document your configurations with comments or external documentation to make it easier for other developers to understand and maintain.
- Version Control: Ensure that your
next.config.js
file is version-controlled along with the rest of your project to track changes and collaborate effectively. - Testing: Test your configurations thoroughly across different environments and scenarios to ensure that they behave as expected and meet project requirements.
- Stay Updated: Keep up with updates and changes to Next.js and its ecosystem to leverage new features, optimizations, and best practices in your configurations.
Conclusion
The next.config.js
file is a crucial component of Next.js applications, providing a centralized location for configuring various aspects of your Next.js projects. By customizing webpack configurations, defining environment variables, optimizing assets, and configuring routing behavior, developers can tailor Next.js applications to meet specific requirements, optimize performance, and enhance developer productivity. Embrace best practices, experiment with different configurations, and leverage the power of the next.config.js
file to build fast, efficient, and scalable web applications with Next.js.
How does Next.js handle state persistence between page navigations
How does Next.js handle routing
What is the purpose of the pages directory in a Next.js project
What is the purpose of the next.config.js option env
What are the considerations for securing a Next.js application
How can you implement A/B testing in a Next.js application
What is the purpose of the disableStaticImages option in next.config.js
How does Next.js handle static assets like images, fonts, and CSS files
How can you implement a custom loading indicator for dynamic routes