How can you create dynamic routes in Next.js
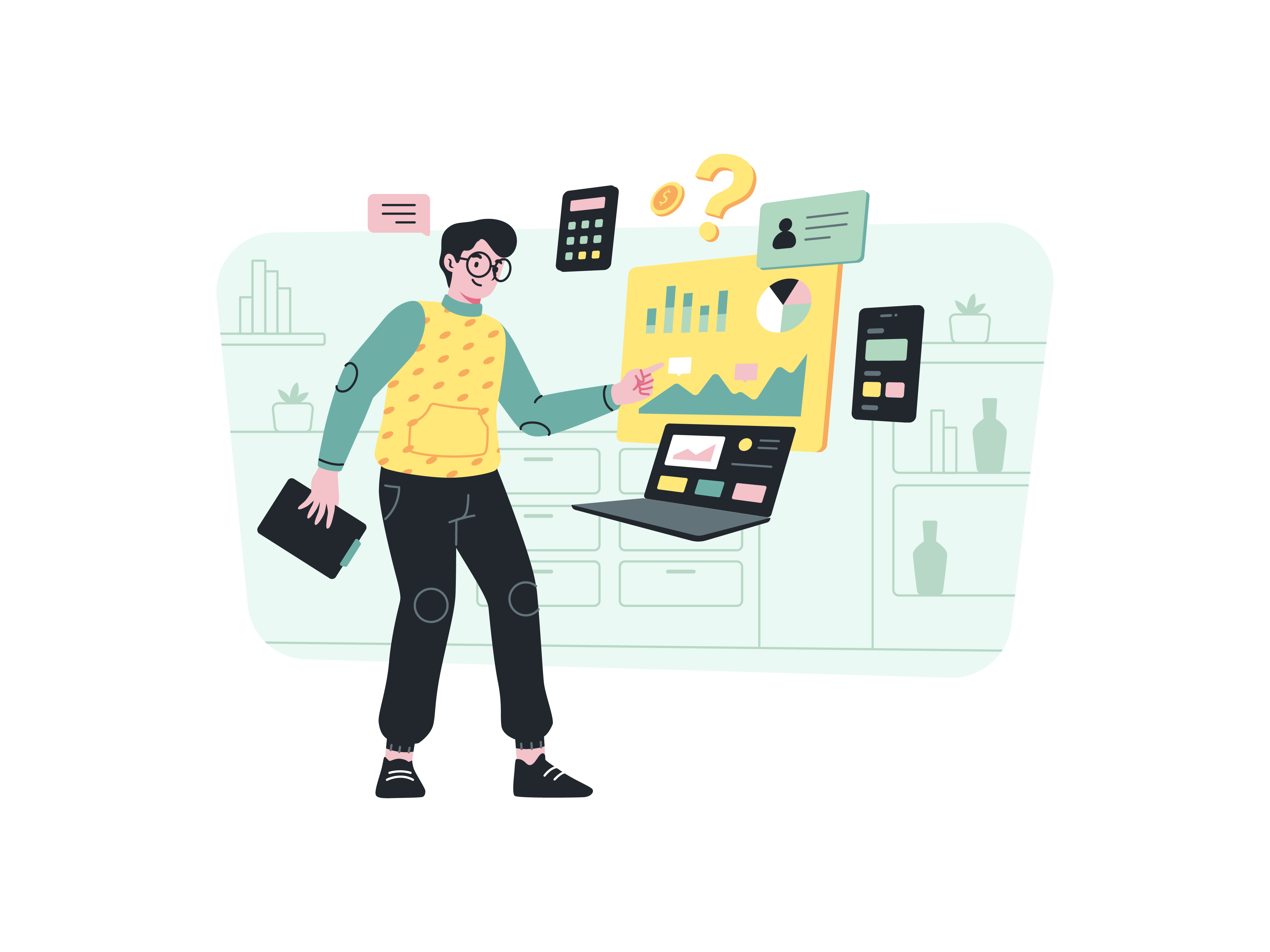
Dynamic routing is a powerful feature of Next.js that allows developers to create dynamic, data-driven web applications with flexible routing patterns. By defining dynamic routes, developers can create pages that respond to user input, parameters, or data from external sources. In this comprehensive guide, we’ll explore how you can create dynamic routes in Next.js, covering various techniques, best practices, and examples.
Understanding Dynamic Routes in Next.js
Before we dive into creating dynamic routes, let’s establish a foundational understanding of dynamic routing in Next.js:
- File-Based Routing: Next.js uses a file-based routing system, where each page component corresponds to a route in the application. Pages are automatically mapped to their respective routes based on the file structure within the
pages
directory. - Dynamic Parameters: Dynamic routes allow developers to define routes with parameters or placeholders that can capture dynamic segments of the URL. These parameters can be accessed programmatically within the page component and used to fetch data or customize the content dynamically.
- Data Fetching: Dynamic routes often involve fetching data asynchronously based on the parameters or context provided by the route. Next.js provides built-in functions like
getStaticProps
andgetServerSideProps
to fetch data at build time or request time, respectively.
Creating Dynamic Routes in Next.js
Next.js offers several approaches for creating dynamic routes, including:
1. Basic Dynamic Routes
To create a basic dynamic route in Next.js, simply create a page component with a filename that includes dynamic parameters enclosed in square brackets ([]
). For example, to create a dynamic route for blog posts with a postId
parameter:
// pages/posts/[postId].js
const Post = ({ postId }) => {
return <h1>Post #{postId}</h1>;
};
export default Post;
export async function getStaticPaths() {
return {
paths: [{ params: { postId: '1' } }, { params: { postId: '2' } }],
fallback: false,
};
}
export async function getStaticProps({ params }) {
const { postId } = params;
return { props: { postId } };
}
2. Catch-All Routes
Next.js also supports catch-all routes, which match any URL segment beyond a specified parameter. To create a catch-all route, use an ellipsis (...
) in the filename within the pages
directory. For example, to create a catch-all route for blog categories:
// pages/posts/[...category].js
const PostsByCategory = ({ category }) => {
return <h1>Posts in Category: {category.join('/')}</h1>;
};
export default PostsByCategory;
export async function getStaticPaths() {
return {
paths: [
{ params: { category: ['react'] } },
{ params: { category: ['nextjs'] } },
],
fallback: false,
};
}
export async function getStaticProps({ params }) {
const { category } = params;
return { props: { category } };
}
3. Nested Routes
Next.js supports nested routes, allowing developers to create hierarchical route structures by nesting directories within the pages
directory. Each nested directory corresponds to a segment of the URL path. For example, to create nested routes for blog posts organized by category:
pages/
|-- posts/
| |-- [category]/
| |-- [postId].js
4. Dynamic Route Parameters
Access dynamic route parameters programmatically within the page component using the useRouter
hook from next/router
. Dynamic parameters are available via the query
object in the router instance. For example, to access the postId
parameter:
import { useRouter } from 'next/router';
const Post = () => {
const router = useRouter();
const { postId } = router.query;
return <h1>Post #{postId}</h1>;
};
export default Post;
Best Practices and Considerations
When creating dynamic routes in Next.js, consider the following best practices and considerations:
- SEO: Ensure that dynamic routes are SEO-friendly by providing descriptive URLs, meta tags, and structured data. Use server-side rendering (
getServerSideProps
) or static generation (getStaticProps
) to pre-render dynamic pages with optimized content for search engines. - Fallbacks: Consider using fallback behavior for dynamic routes to handle scenarios where data may not be available at build time. Fallbacks enable incremental static generation and allow Next.js to serve a skeleton or loading state while fetching data asynchronously.
- Prefetching: Leverage Next.js’s built-in prefetching capabilities to prefetch data or resources for dynamic routes. Use the
next/link
component with theprefetch
attribute to prefetch data in the background and improve navigation performance. - Parameter Validation: Validate dynamic route parameters to ensure that they meet specific criteria or constraints. Use parameter validation libraries or custom validation logic to prevent malicious or unexpected inputs.
- Testing: Test dynamic routes thoroughly across different scenarios, parameter values, and edge cases to ensure robustness and reliability. Consider using automated testing frameworks or tools to streamline testing workflows and catch potential issues early.
Conclusion
Creating dynamic routes in Next.js enables developers to build powerful and flexible web applications with dynamic content and personalized user experiences. By leveraging Next.js’s built-in features for dynamic routing, including basic dynamic routes, catch-all routes, nested routes, and dynamic route parameters, developers can create dynamic and data-driven applications with ease. Embrace best practices, prioritize SEO, performance, and accessibility, and test rigorously to ensure that dynamic routes enhance the overall user experience and contribute to the success of your Next.js projects.
How can you handle state management in a Next.js application
How does data fetching work in Next.js
How does Next.js handle state persistence between page navigations
How does Next.js handle routing
What is the purpose of the pages directory in a Next.js project
What is the purpose of the next.config.js option env
What are the considerations for securing a Next.js application
How can you implement A/B testing in a Next.js application
What is the purpose of the disableStaticImages option in next.config.js
How does Next.js handle static assets like images, fonts, and CSS files