Explain the purpose of the Image component in Next.js
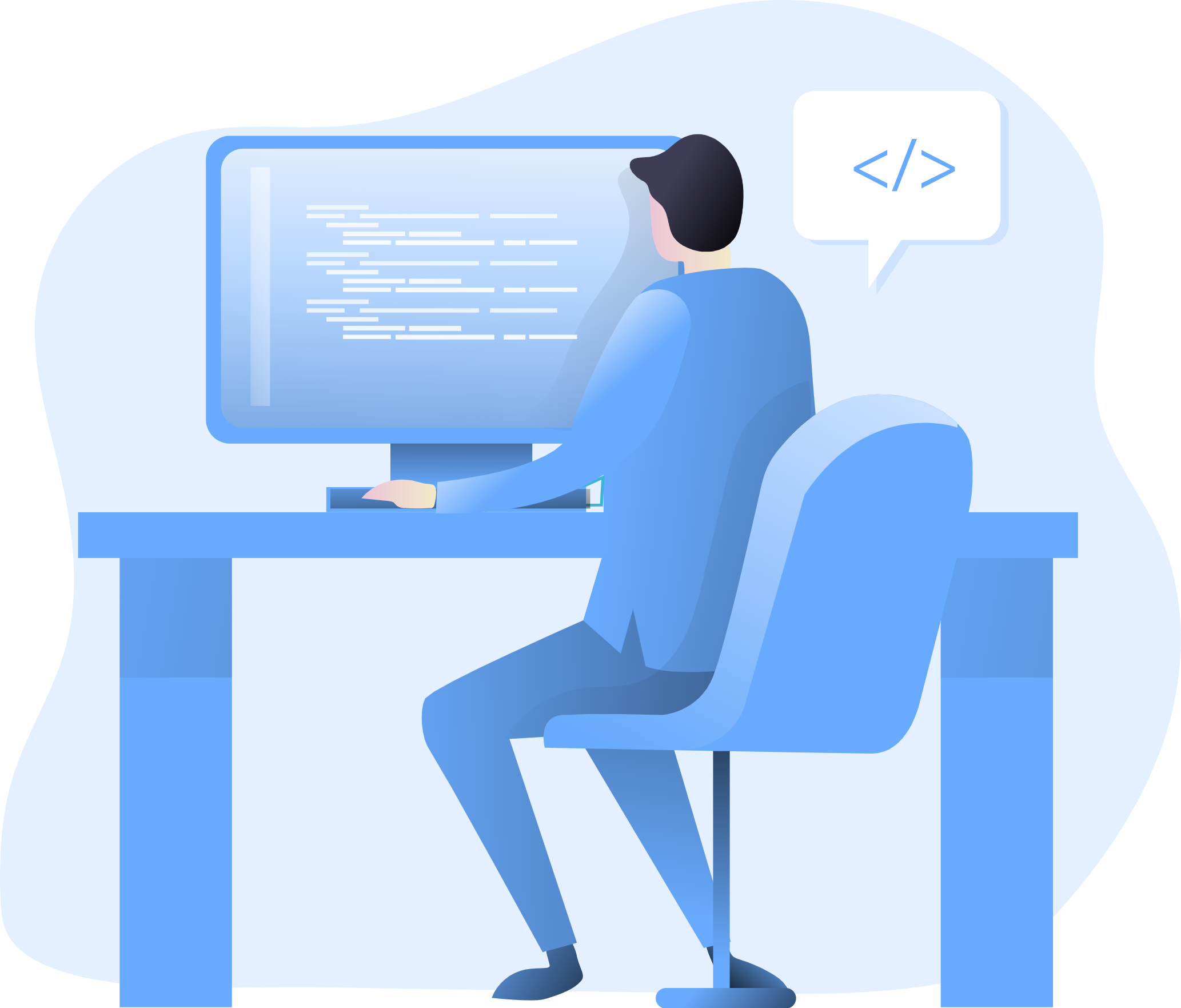
Next.js, a popular React framework, provides developers with powerful tools and components to streamline the development of modern web applications. One such component is the Image component, which offers a convenient and optimized way to handle images in Next.js applications. In this blog post, we’ll delve into the purpose of the Image component in Next.js and explore its features, benefits, and best practices for usage.
Understanding the Importance of Optimized Images
Before we delve into the Image component in Next.js, let’s first understand why optimized images are crucial for web performance and user experience.
- Improved Page Load Times: Large, unoptimized images can significantly slow down page load times, leading to a poor user experience and higher bounce rates.
- Bandwidth Efficiency: Optimized images reduce the amount of data transferred between the server and the client, making the website more bandwidth-efficient, especially on mobile devices with limited data plans.
- Better SEO: Search engines take into account page load times when ranking websites, so optimizing images can indirectly improve search engine optimization (SEO) and visibility.
Introducing the Image Component in Next.js
The Image component in Next.js is designed to address the challenges associated with handling images in web applications. It provides a declarative and efficient way to display images while automatically optimizing them for performance.
Key Features of the Image Component
- Automatic Optimization: The Image component automatically optimizes images by using advanced techniques such as lazy loading, responsive resizing, and image optimization based on the device’s screen size and resolution.
- Optimized Loading: Images are lazy-loaded by default, meaning they are only loaded when they enter the viewport, reducing initial page load times and improving performance.
- Automatic Image Optimization: Next.js automatically optimizes images using the next/image plugin, which provides support for various image formats, including JPEG, PNG, WebP, and SVG.
Usage of the Image Component
Using the Image component in Next.js is straightforward and requires only minimal configuration. Here’s a basic example of how to use the Image component to display an image on a Next.js page:
import Image from 'next/image';
const MyImage = () => {
return (
<div>
<Image
src="/path/to/image.jpg"
alt="Description of the image"
width={500}
height={300}
/>
</div>
);
};
export default MyImage;
In this example, we’re importing the Image component from Next.js and passing it the src
, alt
, width
, and height
props to specify the image source, alternative text for accessibility, and dimensions of the image.
Benefits of Using the Image Component
- Improved Performance: By automatically optimizing images and lazy-loading them, the Image component in Next.js helps improve page load times and overall performance.
- Accessibility: The Image component supports accessibility features such as providing alternative text (
alt
) for screen readers, ensuring that images are accessible to all users, including those with disabilities. - Responsive Design: The Image component supports responsive design out of the box, allowing images to adapt to different screen sizes and resolutions without manual intervention.
Conclusion
The Image component in Next.js is a powerful tool for handling images in web applications, offering automatic optimization, lazy loading, and support for responsive design. By leveraging the Image component, developers can improve page load times, enhance accessibility, and ensure a better user experience for their Next.js applications. Whether you’re building a portfolio website, an e-commerce platform, or a blog, the Image component in Next.js is a valuable asset for delivering high-quality images efficiently and effectively.
What are React fragments, and when would you use them
How does React handle animations
How do you handle authentication in a React application
What is the purpose of the useCallback hook in React
What are the different lifecycle methods in a React component
How do you handle code splitting with React Router
How does React handle code splitting with React.lazy, Suspense, and webpack 5