What are the different lifecycle methods in a React component
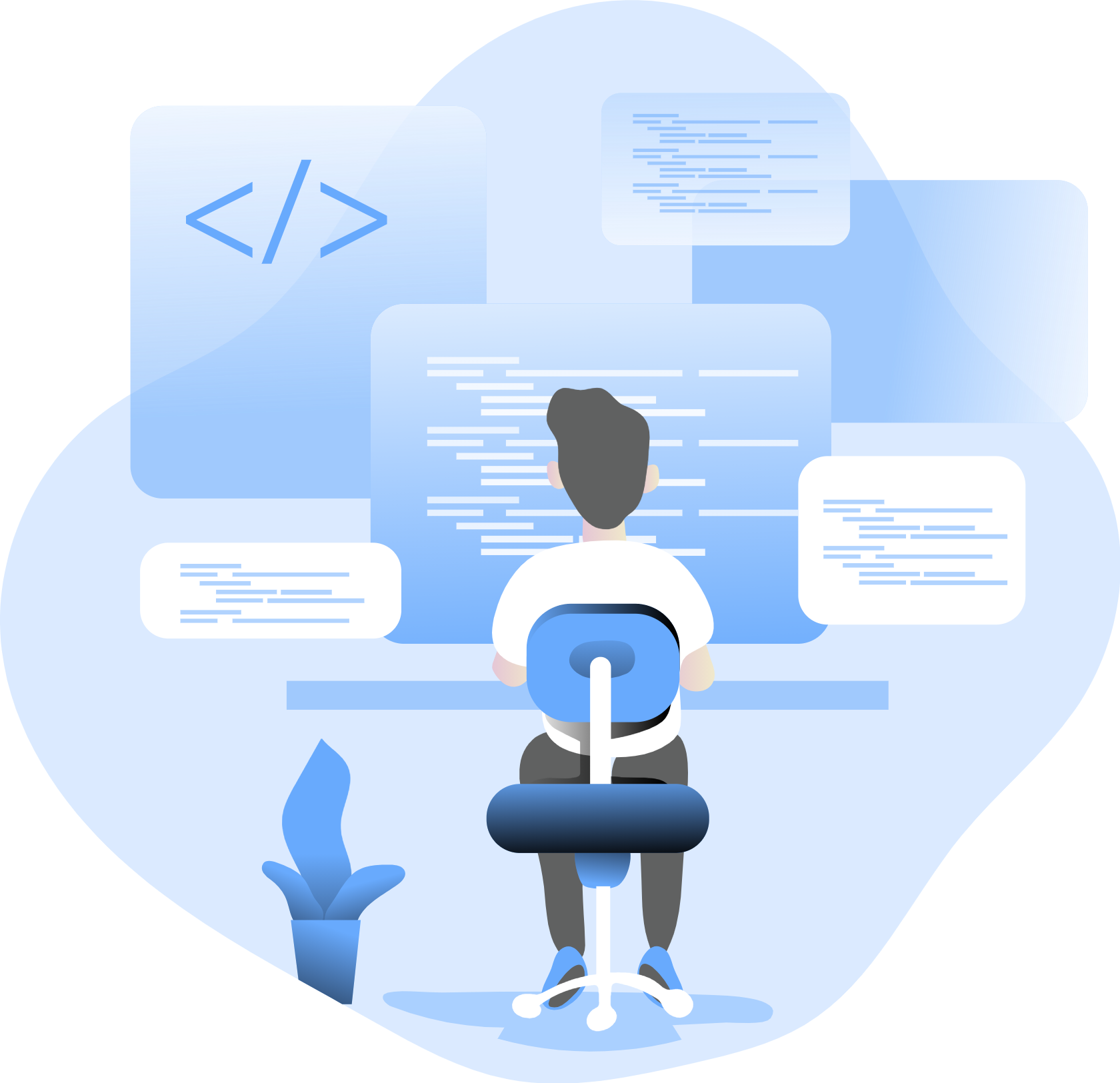
React, with its component-based architecture, provides developers with a rich set of lifecycle methods that dictate the different phases a component goes through, from initialization to destruction. Understanding these lifecycle methods is crucial for efficient state management, side effects, and overall application performance. In this comprehensive guide, we’ll delve into the various lifecycle methods in a React component, exploring their purposes, usage, and best practices for effective component development.
-
Initialization Phase
-
constructor()
Method: Theconstructor
method is the first lifecycle method called when a component is created. It is used for initializing state, binding event handlers, and setting up any initial configurations. Make sure to callsuper(props)
within the constructor to properly set up the component.class MyComponent extends React.Component { constructor(props) { super(props); this.state = { // Initial state setup }; // Event handler binding and other initializations } // Rest of the component code }
-
-
Mounting Phase
-
static getDerivedStateFromProps(props, state)
Method: This static method is called before every render, providing an opportunity to update the state based on changes in props. It returns an object to update the state ornull
to indicate no state update is necessary.static getDerivedStateFromProps(nextProps, prevState) { // Compare nextProps and prevState, return an object to update state or null }
-
render()
Method: Therender
method is a required lifecycle method responsible for returning the JSX that represents the component’s UI. It should be a pure function, free of side effects.render() { return ( // JSX representing the component's UI ); }
-
componentDidMount()
Method: Invoked immediately after a component is mounted (inserted into the DOM). This is a common place to initiate network requests, subscriptions, or set up timers.componentDidMount() { // Perform actions after the component is mounted }
-
-
Updating Phase
-
shouldComponentUpdate(nextProps, nextState)
Method: This method is invoked before rendering when new props or state are received. It returns a boolean indicating whether the component should re-render. ImplementingshouldComponentUpdate
can optimize performance by preventing unnecessary renders.shouldComponentUpdate(nextProps, nextState) { // Compare nextProps and nextState, return true if rendering is necessary, false otherwise }
-
static getDerivedStateFromProps(nextProps, prevState)
Method (Again): While this method is primarily used in the mounting phase, it is also called during the updating phase, providing an opportunity to update the state based on changes in props.static getDerivedStateFromProps(nextProps, prevState) { // Compare nextProps and prevState, return an object to update state or null }
-
render()
Method (Again): Therender
method is called again when the component needs to re-render due to changes in props or state. -
getSnapshotBeforeUpdate(prevProps, prevState)
Method: This method is called right before the changes from the virtual DOM are to be reflected in the actual DOM. It receives the previous props and state and can return a value that will be passed as a third parameter to thecomponentDidUpdate
method.getSnapshotBeforeUpdate(prevProps, prevState) { // Return a value that will be passed to componentDidUpdate }
-
componentDidUpdate(prevProps, prevState, snapshot)
Method: Invoked immediately after the component is updated. It receives the previous props and state, as well as the snapshot value returned bygetSnapshotBeforeUpdate
.componentDidUpdate(prevProps, prevState, snapshot) { // Perform actions after the component is updated }
-
-
Unmounting Phase
-
componentWillUnmount()
Method: Invoked immediately before a component is unmounted and destroyed. It is a good place to clean up any subscriptions, timers, or other resources to prevent memory leaks.componentWillUnmount() { // Clean up actions before the component is unmounted }
-
-
Error Handling
-
static getDerivedStateFromError(error)
Method: This static method is called when there is an error during rendering, allowing the component to catch the error and update the state. It is followed by thecomponentDidCatch
method.static getDerivedStateFromError(error) { // Update state based on the error }
-
componentDidCatch(error, info)
Method: Invoked after an error has been thrown by a descendant component. It receives the error
-
and an info object with information about which component threw the error.
```jsx
componentDidCatch(error, info) {
// Log the error or perform other actions
}
```
-
Best Practices and Considerations
- Avoid Direct State Mutation:
Directly mutating the state is not recommended. Instead, use the
setState
method to update state, ensuring proper re-rendering. - Use Functional setState for Asynchronous Updates:
When updating the state based on the previous state, use the functional form of
setState
to ensure correct behavior in asynchronous updates. - Perform Asynchronous Operations in
componentDidMount
andcomponentDidUpdate
: Asynchronous operations, such as data fetching, should be performed incomponentDidMount
andcomponentDidUpdate
to avoid blocking the rendering process. - Clean Up Resources in
componentWillUnmount
: Any resources, subscriptions, or timers created incomponentDidMount
should be cleaned up incomponentWillUnmount
to prevent memory leaks. - Optimize with
shouldComponentUpdate
: ImplementingshouldComponentUpdate
can significantly optimize performance by preventing unnecessary renders.
- Avoid Direct State Mutation:
Directly mutating the state is not recommended. Instead, use the
-
Conclusion
React component lifecycles provide a structured way to manage the different phases a component goes through, from initialization to destruction. By understanding and utilizing these lifecycle methods, developers can optimize performance, manage side effects, and create robust and efficient React applications. Implementing best practices ensures a clean and maintainable codebase, enhancing the overall development experience in the world of React.
How to use Bootstrap’s utility classes for flexbox alignment
How does React differ from other JavaScript frameworks
What is JSX, and how does it differ from regular JavaScript syntax
Uncover React’s Virtual DOM: Exploring Its Crucial Role and Advantages
What are the key features of React
What are the utility classes for responsive design in Tailwind CSS