How to implement a custom loading state for data fetching in a Next.js app
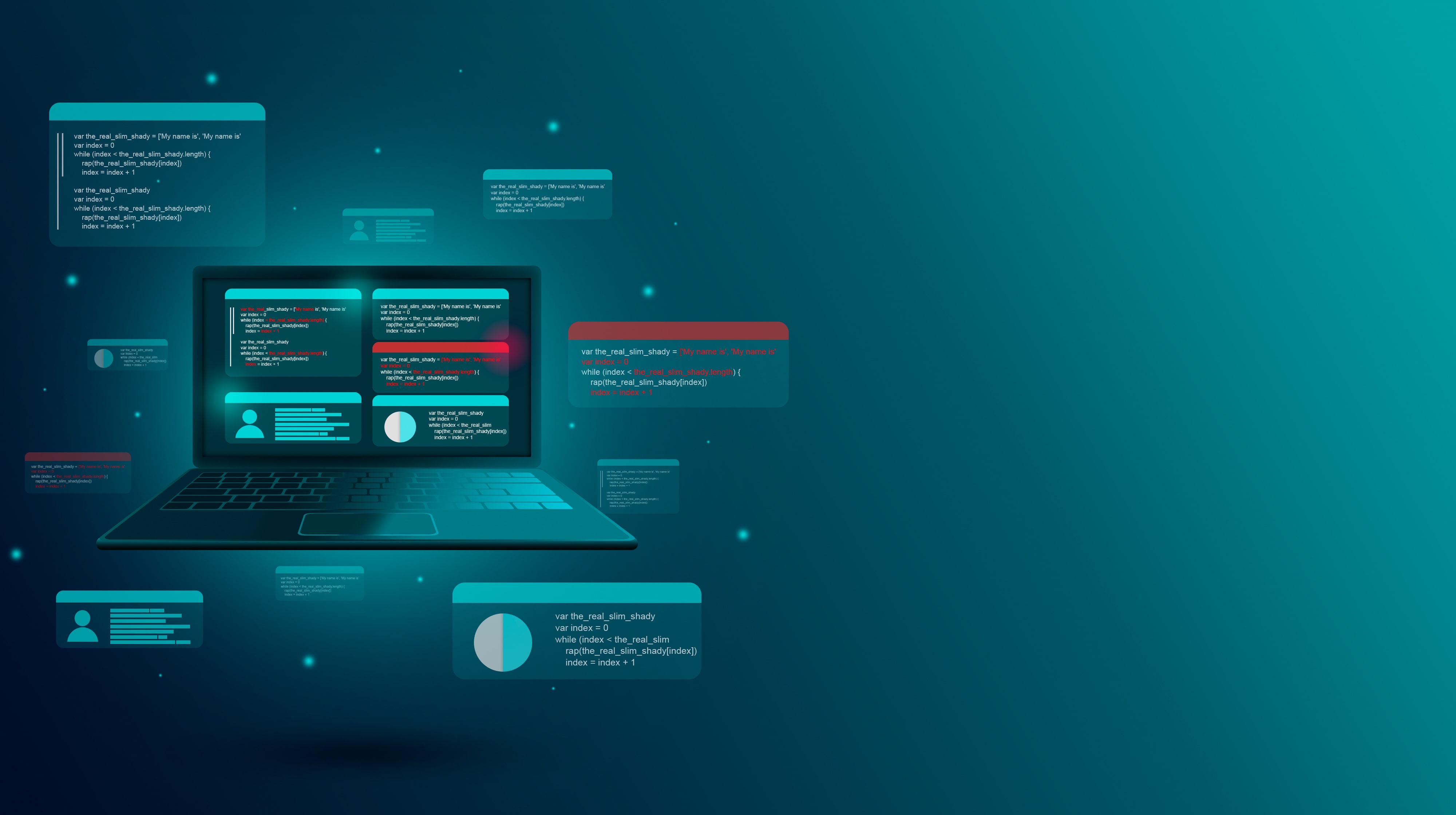
In a Next.js application, providing a smooth and engaging user experience is crucial, especially when fetching data from external sources. A custom loading state allows you to display informative feedback to users while data is being fetched, enhancing usability and reducing frustration. In this blog post, we’ll explore how to implement a custom loading state for data fetching in a Next.js app, empowering you to create a more polished and user-friendly application.
Understanding the Importance of a Custom Loading State
When fetching data asynchronously in a Next.js app, there’s a brief period during which users may experience a delay before the data is displayed. During this time, it’s essential to provide feedback to users, indicating that the application is working on their request. A custom loading state serves this purpose by displaying a loading indicator or message, reassuring users that their action is being processed.
Implementing a Custom Loading State in Next.js
Let’s walk through the steps to implement a custom loading state for data fetching in a Next.js app:
1. Set Up State Management
Start by setting up state management in your Next.js app to track the loading state. You can use React’s built-in useState
hook or a state management library like Redux, depending on your project’s requirements.
// components/ExampleComponent.js
import { useState } from 'react';
const ExampleComponent = () => {
const [isLoading, setIsLoading] = useState(false);
// Other component logic
return (
<div>
{/* Render loading indicator if isLoading is true */}
{isLoading && <div>Loading...</div>}
{/* Render content based on data */}
{!isLoading && <div>Content Here</div>}
</div>
);
};
export default ExampleComponent;
2. Fetch Data
Next, implement the data fetching logic in your component using fetch
or any other method of your choice. While fetching data, update the loading state to true
to indicate that the request is in progress.
// components/ExampleComponent.js
import { useState, useEffect } from 'react';
const ExampleComponent = () => {
const [isLoading, setIsLoading] = useState(false);
const [data, setData] = useState(null);
useEffect(() => {
const fetchData = async () => {
setIsLoading(true);
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
setData(data);
} catch (error) {
console.error('Error fetching data:', error);
} finally {
setIsLoading(false);
}
};
fetchData();
}, []);
return (
<div>
{/* Render loading indicator if isLoading is true */}
{isLoading && <div>Loading...</div>}
{/* Render content based on data */}
{!isLoading && data && <div>{JSON.stringify(data)}</div>}
</div>
);
};
export default ExampleComponent;
3. Display Loading State
Finally, update your component to conditionally render the loading indicator or message based on the loading state.
// components/ExampleComponent.js
import { useState, useEffect } from 'react';
const ExampleComponent = () => {
const [isLoading, setIsLoading] = useState(false);
const [data, setData] = useState(null);
useEffect(() => {
const fetchData = async () => {
setIsLoading(true);
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
setData(data);
} catch (error) {
console.error('Error fetching data:', error);
} finally {
setIsLoading(false);
}
};
fetchData();
}, []);
return (
<div>
{/* Render loading indicator if isLoading is true */}
{isLoading && <div>Loading...</div>}
{/* Render content based on data */}
{!isLoading && data && <div>{JSON.stringify(data)}</div>}
</div>
);
};
export default ExampleComponent;
Conclusion
Implementing a custom loading state for data fetching in a Next.js app is essential for providing a seamless user experience. By setting up state management, fetching data asynchronously, and displaying informative loading indicators, you can keep users informed and engaged while waiting for data to load. Incorporate these techniques into your Next.js applications to enhance usability and create a more polished user interface.
How does Next.js differ from traditional React applications
How does client-side rendering (CSR) work in Next.js
How does Next.js handle bundling and code splitting
What is incremental static regeneration in Next.js
Can a Next.js application use both server-side rendering and client-side rendering
How does Next.js handle SEO for dynamic pages
How can you implement custom 404 error handling for API routes
What is the purpose of the styled-jsx library in Next.js
What is the purpose of the getStaticPaths function in dynamic routes