Explain the purpose of the publicRuntimeConfig and serverRuntimeConfig in next.config.js
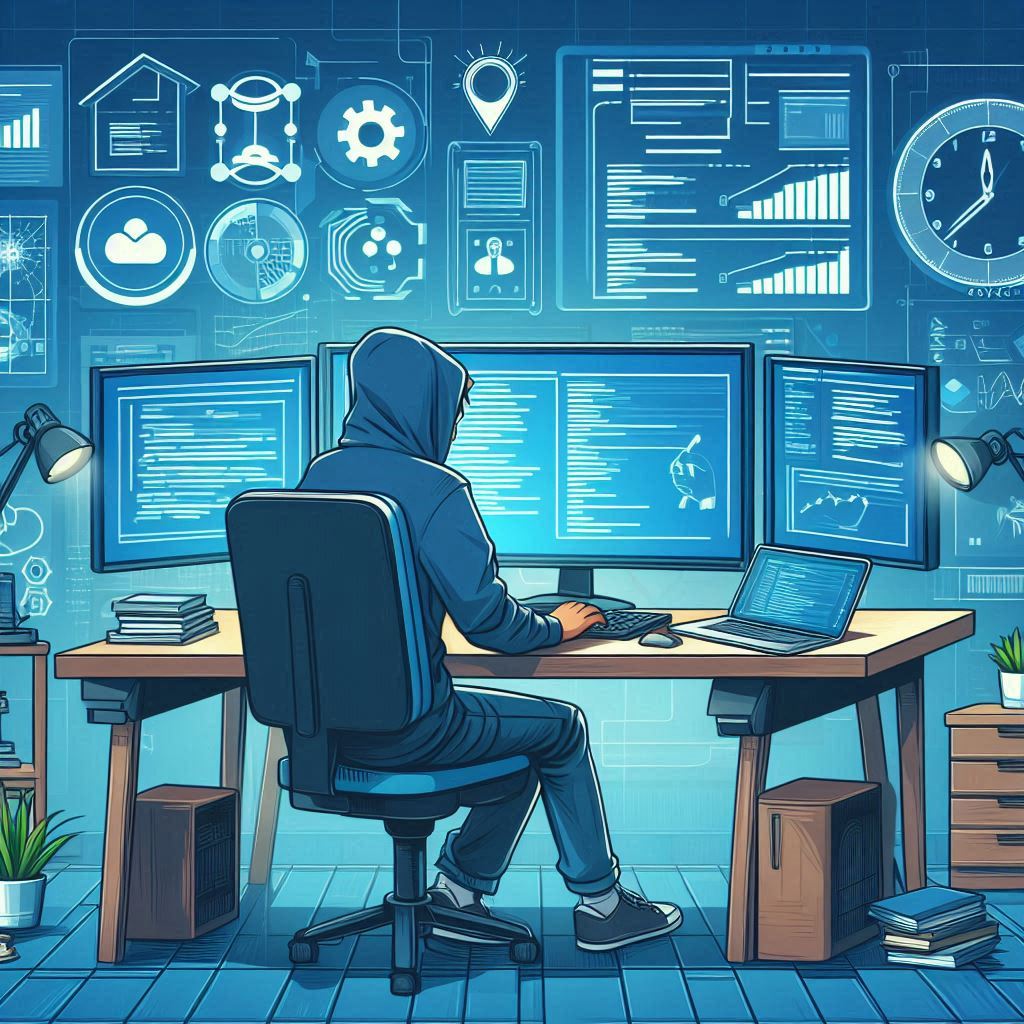
When building a Next.js application, one of the key aspects to consider is how to manage and configure environment variables and runtime configurations. Next.js provides a flexible way to define and access these configurations through the next.config.js
file. Two important properties within this file are publicRuntimeConfig
and serverRuntimeConfig
. These properties help you manage configurations that are either public or restricted to the server.
In this article, we’ll explore the purpose of publicRuntimeConfig
and serverRuntimeConfig
, how they work, and when to use them in your Next.js projects.
What is next.config.js
?
Before diving into the specifics of publicRuntimeConfig
and serverRuntimeConfig
, it’s important to understand the role of next.config.js
in a Next.js application.
next.config.js
is a configuration file that allows you to customize the behavior of your Next.js application. It is used to define various settings, such as custom Webpack configurations, environment variables, image optimization settings, and more. The configurations specified in next.config.js
are available both during the build phase and at runtime.
A basic next.config.js
file might look like this:
// next.config.js
module.exports = {
reactStrictMode: true,
};
Now, let’s explore the publicRuntimeConfig
and serverRuntimeConfig
properties in more detail.
What is publicRuntimeConfig
?
publicRuntimeConfig
is a property within next.config.js
that allows you to define runtime configuration values that are accessible on both the client and server side of your Next.js application. These values are exposed to the client and can be safely used in the browser.
Purpose of publicRuntimeConfig
The primary purpose of publicRuntimeConfig
is to store configuration values that you want to expose to the client while maintaining flexibility in defining them at runtime. This is particularly useful for environment-specific settings, such as API endpoints, feature flags, and other client-side configurations.
Example Usage
Let’s consider an example where you want to define an API endpoint that varies based on the environment (e.g., development, staging, production). You can define this endpoint in publicRuntimeConfig
as follows:
// next.config.js
module.exports = {
publicRuntimeConfig: {
apiEndpoint: process.env.API_ENDPOINT,
},
};
In this example, the apiEndpoint
configuration value is defined in publicRuntimeConfig
, allowing it to be accessed both on the client and server side. The value of process.env.API_ENDPOINT
can be set differently for each environment.
Accessing publicRuntimeConfig
To access publicRuntimeConfig
in your application, you can use the getConfig
function provided by Next.js:
import getConfig from 'next/config';
const { publicRuntimeConfig } = getConfig();
console.log(publicRuntimeConfig.apiEndpoint);
This code will log the value of apiEndpoint
to the console, regardless of whether it’s executed on the server or client.
Use Cases for publicRuntimeConfig
Here are some common use cases for publicRuntimeConfig
:
- API Endpoints: Defining different API endpoints for different environments (e.g., development, staging, production).
- Feature Flags: Enabling or disabling features based on runtime configurations.
- Third-Party Service Keys: Storing public keys or IDs for third-party services that need to be accessed on the client side.
What is serverRuntimeConfig
?
serverRuntimeConfig
is another property within next.config.js
that allows you to define runtime configuration values that are only accessible on the server side. These values are not exposed to the client and are kept secure on the server.
Purpose of serverRuntimeConfig
The primary purpose of serverRuntimeConfig
is to store sensitive or server-specific configuration values that should not be exposed to the client. This is particularly useful for storing API keys, database connection strings, and other sensitive information.
Example Usage
Let’s consider an example where you want to define a secret API key that should only be accessible on the server side:
// next.config.js
module.exports = {
serverRuntimeConfig: {
secretApiKey: process.env.SECRET_API_KEY,
},
};
In this example, the secretApiKey
configuration value is defined in serverRuntimeConfig
, ensuring that it remains secure and is not exposed to the client.
Accessing serverRuntimeConfig
To access serverRuntimeConfig
in your application, you can use the getConfig
function, similar to publicRuntimeConfig
:
import getConfig from 'next/config';
const { serverRuntimeConfig } = getConfig();
console.log(serverRuntimeConfig.secretApiKey);
This code will log the value of secretApiKey
to the console, but only when executed on the server side. If you try to access serverRuntimeConfig
on the client side, it will be undefined.
Use Cases for serverRuntimeConfig
Here are some common use cases for serverRuntimeConfig
:
- API Keys: Storing sensitive API keys that should not be exposed to the client.
- Database Connection Strings: Storing database connection strings or credentials that are only needed on the server side.
- Private Configuration Values: Any other configuration values that should remain private and secure on the server.
Combining publicRuntimeConfig
and serverRuntimeConfig
In many cases, you may need to use both publicRuntimeConfig
and serverRuntimeConfig
in the same application. Next.js allows you to define both properties in next.config.js
and access them as needed.
Example
Here’s an example of how you might use both publicRuntimeConfig
and serverRuntimeConfig
in a single next.config.js
file:
// next.config.js
module.exports = {
publicRuntimeConfig: {
apiEndpoint: process.env.API_ENDPOINT,
},
serverRuntimeConfig: {
secretApiKey: process.env.SECRET_API_KEY,
},
};
In this example, apiEndpoint
is defined in publicRuntimeConfig
and can be accessed on both the client and server sides, while secretApiKey
is defined in serverRuntimeConfig
and is only accessible on the server side.
Accessing Both Configurations
You can access both publicRuntimeConfig
and serverRuntimeConfig
using the getConfig
function:
import getConfig from 'next/config';
const { publicRuntimeConfig, serverRuntimeConfig } = getConfig();
console.log(publicRuntimeConfig.apiEndpoint);
console.log(serverRuntimeConfig.secretApiKey);
This code will log both the apiEndpoint
and secretApiKey
values, but remember that serverRuntimeConfig
will only be available on the server side.
Best Practices for Using publicRuntimeConfig
and serverRuntimeConfig
When working with publicRuntimeConfig
and serverRuntimeConfig
, it’s important to follow best practices to ensure your application is secure, maintainable, and performant.
1. Avoid Exposing Sensitive Information
Be mindful of the information you store in publicRuntimeConfig
. Since these values are exposed to the client, they should not include any sensitive data, such as API keys or credentials.
2. Use Environment Variables
Leverage environment variables to define your configurations. This allows you to easily manage different settings for different environments (e.g., development, staging, production) without hardcoding values in your codebase.
3. Keep Configurations Modular
If your application has a large number of configurations, consider modularizing them by grouping related settings together. This can make your next.config.js
file more organized and easier to manage.
4. Document Your Configurations
Ensure that your configurations are well-documented. This helps other developers understand the purpose and usage of each configuration value, reducing the likelihood of errors or misconfigurations.
5. Use Server-Side Configurations for Sensitive Data
Always use serverRuntimeConfig
for sensitive data that should not be exposed to the client. This ensures that your sensitive information remains secure and is only accessible on the server side.
Conclusion
Understanding the purpose and usage of publicRuntimeConfig
and serverRuntimeConfig
in next.config.js
is crucial for effectively managing runtime configurations in your Next.js application. By using publicRuntimeConfig
, you can define configuration values that are accessible on both the client and server sides, while serverRuntimeConfig
allows you to keep sensitive data secure and only accessible on the server.
By following best practices and leveraging these properties, you can build a secure, maintainable, and performant Next.js application that adapts to different environments and use cases. Whether you’re defining API endpoints, managing feature flags, or securing sensitive data, publicRuntimeConfig
and serverRuntimeConfig
provide the flexibility and control you need to handle runtime configurations with confidence.
How does React handle conditional rendering
What is the significance of the useEffect hook in functional components
How do you manage state in a Redux store
How does React handle memoization
How do you handle forms in React with controlled and uncontrolled components
What is the purpose of the useMemo hook in React