How do you handle forms in React with controlled and uncontrolled components
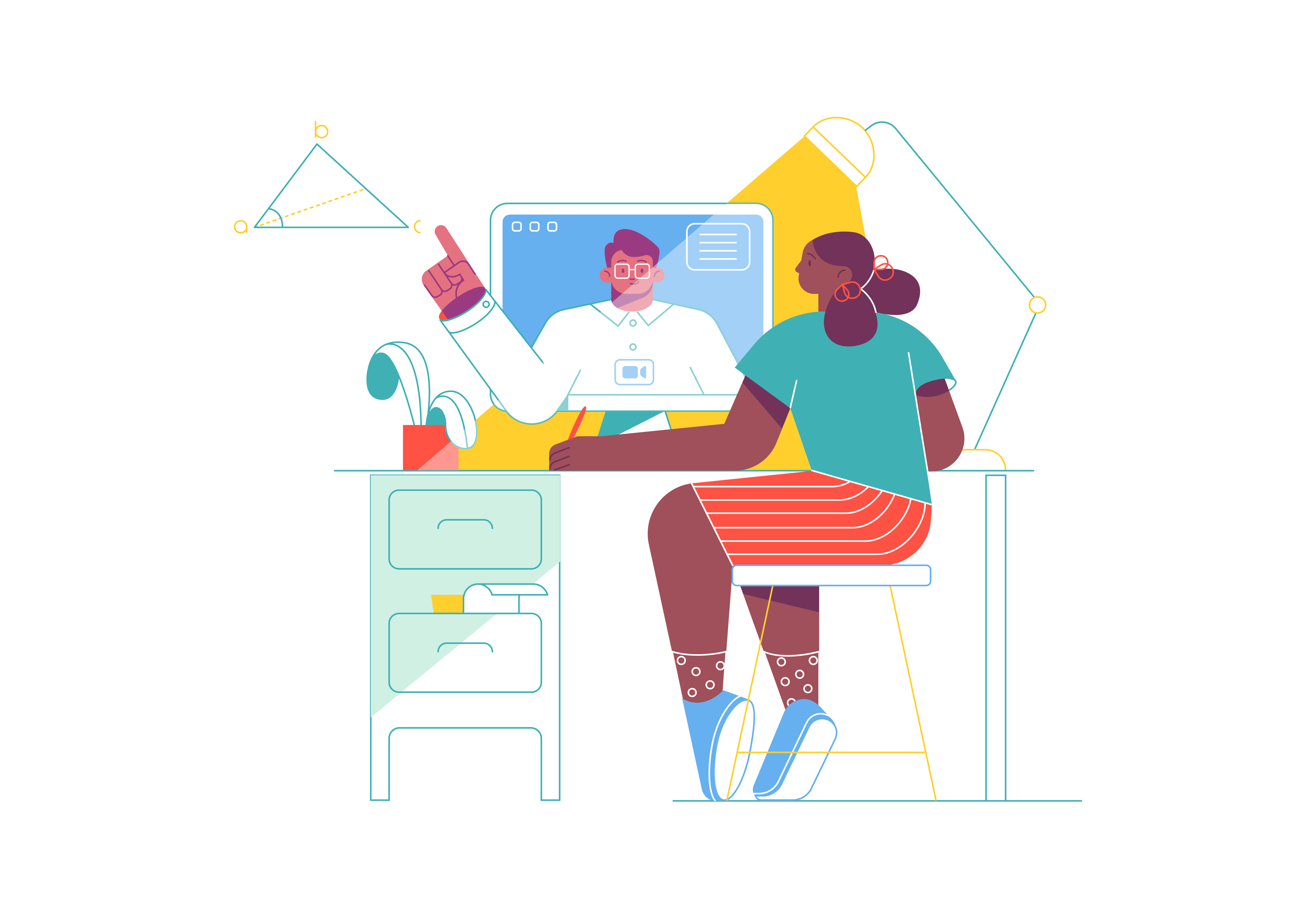
In the realm of React development, efficiently handling forms is a crucial skill for building interactive and user-friendly applications. React provides developers with two primary approaches for form management: controlled components and uncontrolled components. In this comprehensive guide, we’ll explore the nuances of both methods, helping you navigate the intricacies of form handling in React.
Understanding Controlled Components
Controlled components in React maintain their state in the parent component, typically through the use of the useState
hook. The state of the form elements (such as input fields, checkboxes, and radio buttons) is controlled and updated through the state property and associated functions.
Creating a Controlled Component
Let’s consider a simple example of a controlled component managing a form input field.
import React, { useState } from 'react';
const ControlledForm = () => {
const [inputValue, setInputValue] = useState('');
const handleInputChange = (e) => {
setInputValue(e.target.value);
};
const handleSubmit = (e) => {
e.preventDefault();
// Process the form data
console.log('Form submitted with value:', inputValue);
};
return (
<form onSubmit={handleSubmit}>
<label>
Enter Text:
<input type="text" value={inputValue} onChange={handleInputChange} />
</label>
<button type="submit">Submit</button>
</form>
);
};
export default ControlledForm;
In this example, the inputValue
state is controlled by the component, and any changes to the input field trigger the handleInputChange
function, updating the state accordingly. The value of the input field is explicitly set to inputValue
, making it a controlled component.
Advantages of Controlled Components
- Predictable State: Since the state is controlled by React, the application has a predictable and centralized source of truth for the form data.
- Dynamic Updates: You have granular control over how and when the state is updated, allowing for dynamic interactions and validations.
- Easier Testing: Testing controlled components is straightforward as you can easily manipulate and assert the state in your tests.
Unleashing the Power of Uncontrolled Components
While controlled components provide a structured and predictable approach, uncontrolled components offer a more flexible and sometimes simpler solution for handling forms. In uncontrolled components, the form elements maintain their state independently, with React acting as more of an observer than a controller.
Implementing an Uncontrolled Component
Let’s look at how an uncontrolled component handles form input.
import React, { useRef } from 'react';
const UncontrolledForm = () => {
const inputRef = useRef();
const handleSubmit = (e) => {
e.preventDefault();
// Access the form data using the inputRef
console.log('Form submitted with value:', inputRef.current.value);
};
return (
<form onSubmit={handleSubmit}>
<label>
Enter Text:
<input type="text" ref={inputRef} />
</label>
<button type="submit">Submit</button>
</form>
);
};
export default UncontrolledForm;
In this example, the inputRef
is used to reference the input field directly. React does not manage the state of the input field; instead, the form data is retrieved directly from the DOM using the ref
.
Advantages of Uncontrolled Components
- Simplicity: Uncontrolled components are often simpler and require less code compared to their controlled counterparts.
- Direct DOM Manipulation: If you need direct access to the DOM or want to integrate with non-React libraries, uncontrolled components can be more convenient.
- Performance: In some scenarios, uncontrolled components might offer better performance, especially in situations where the controlled approach might lead to unnecessary re-renders.
Choosing Between Controlled and Uncontrolled Components
The decision between controlled and uncontrolled components depends on various factors, including the complexity of the form, the desired level of control, and the specific use case. Here are some considerations to help you make an informed choice:
Use Controlled Components When
- Dynamic Interactions: If your form requires dynamic interactions, such as conditional rendering or dynamic validations, controlled components provide a clear and controlled way to handle these scenarios.
- Predictable State: When having a centralized and predictable state is crucial for your application logic and state management.
- Form Validation: Controlled components make it easier to implement and manage form validation logic.
Use Uncontrolled Components When
- Simplicity Matters: For simpler forms or when minimizing boilerplate code is a priority, uncontrolled components can be a more straightforward solution.
- Integration with Non-React Code: If your form needs to integrate with non-React code or relies heavily on direct DOM manipulation, uncontrolled components might be more suitable.
- Performance Concerns: In certain situations, uncontrolled components can offer better performance, especially in large or complex forms where avoiding unnecessary re-renders is beneficial.
Combining Controlled and Uncontrolled Components
In some scenarios, a hybrid approach that combines both controlled and uncontrolled components might be the most pragmatic solution. For instance, you might use controlled components for critical form elements while opting for uncontrolled components for less critical or dynamic parts of the form.
import React, { useState, useRef } from 'react';
const HybridForm = () => {
const [controlledValue, setControlledValue] = useState('');
const uncontrolledInputRef = useRef();
const handleControlledChange = (e) => {
setControlledValue(e.target.value);
};
const handleSubmit = (e) => {
e.preventDefault();
console.log('Controlled value:', controlledValue);
console.log('Uncontrolled value:', uncontrolledInputRef.current.value);
};
return (
<form onSubmit={handleSubmit}>
<label>
Controlled Input:
<input type="text" value={controlledValue} onChange={handleControlledChange} />
</label>
<br />
<label>
Uncontrolled Input:
<input type="text" ref={uncontrolledInputRef} />
</label>
<br />
<button type="submit">Submit</button>
</form>
);
};
export default HybridForm;
In this example, the controlled and uncontrolled components coexist within the same form, showcasing the flexibility of combining these approaches based on specific form requirements.
Conclusion
Form handling in React is a nuanced art, and choosing between controlled and uncontrolled components depends on the unique characteristics of your application. Whether you opt for the structured control of state in controlled components or the flexibility of uncontrolled components, understanding the strengths and trade-offs of each approach is crucial.
As you navigate the seas of React form handling, consider the specific needs of your application and strike a balance between control and flexibility. Armed with the knowledge of controlled and uncontrolled components, you’ll be well-equipped to create forms that seamlessly integrate with your React applications, providing users with a smooth and interactive experience.
How to use Bootstrap’s form control sizing classes
How to create a responsive image carousel with captions
How to use Bootstrap’s responsive utilities for text alignment
How many states are there in React