What is the purpose of the useMemo hook in React
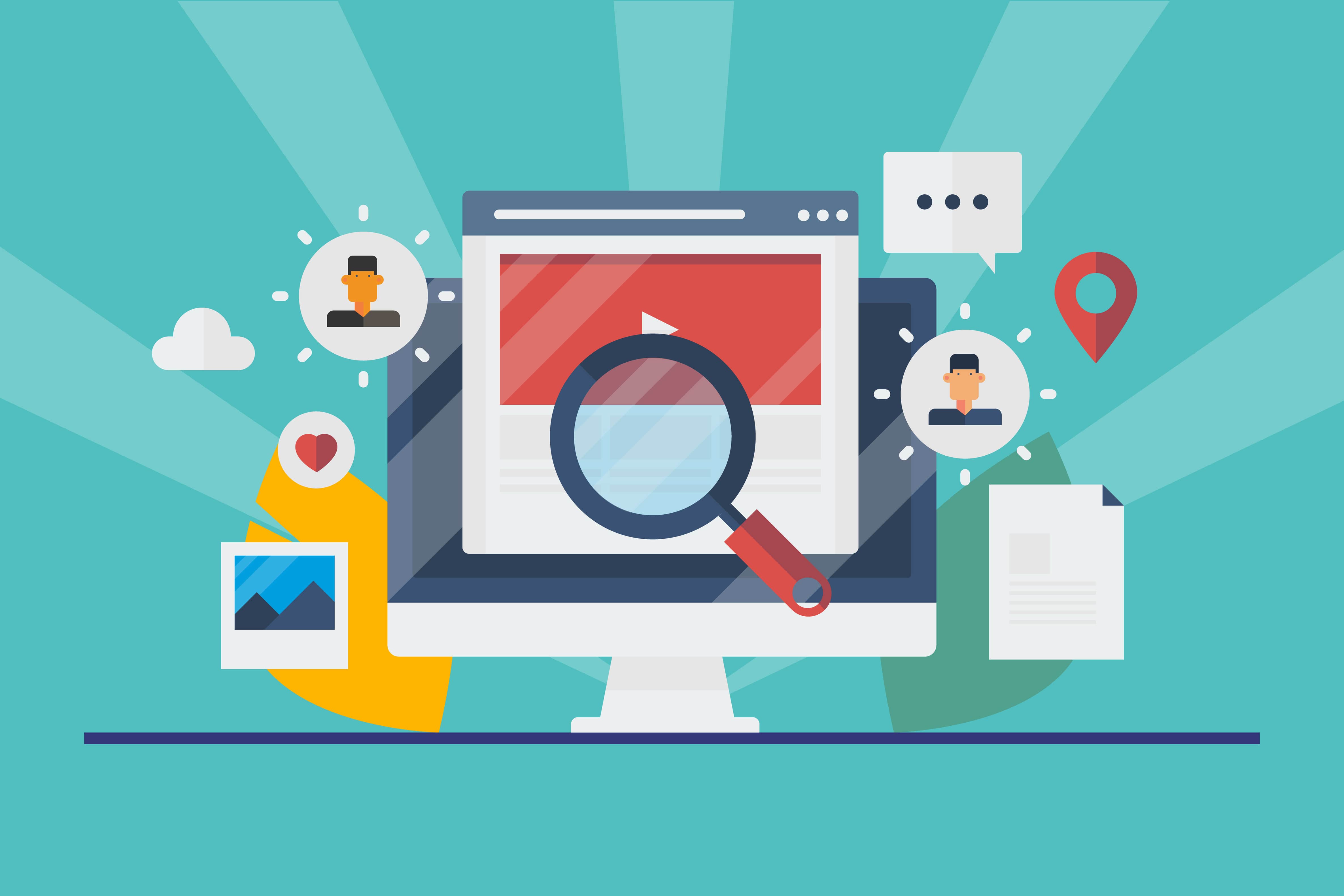
React, a JavaScript library for building user interfaces, provides developers with a powerful set of tools to create efficient and performant applications. One such tool is the useMemo hook, a feature introduced in React 16.8. In this article, we’ll delve into the purpose of the useMemo hook, exploring how it enhances the performance of React applications by optimizing the computation of expensive calculations.
The Basics of React Hooks
Before we dive into useMemo, let’s briefly review the fundamentals of React hooks. Hooks are functions that enable developers to use state and lifecycle features in functional components. Unlike class components, functional components traditionally lacked state and lifecycle methods. With the advent of hooks, React developers gained the ability to manage state and perform side effects in functional components.
One of the most common hooks is useState, which allows components to manage state. Another important hook is useEffect, which handles side effects like data fetching, subscriptions, or manually changing the DOM. While these hooks contribute significantly to the development of robust and scalable applications, they may not always be the most efficient when dealing with computationally expensive tasks.
The Problem with Unnecessary Recalculation
In React, when a component renders, all the code within its functional body is executed. This includes calculations, data fetching, and other computations. However, if a component is rendering frequently, it may lead to unnecessary recalculations of values that remain unchanged between renders.
Consider a scenario where a component is responsible for rendering a list of items, and a costly calculation is performed for each item. Without optimization, this calculation is re-executed every time the component renders, even if the list remains unchanged. This can result in performance bottlenecks, especially in larger applications or when dealing with complex computations.
Introducing useMemo
The useMemo hook addresses this issue by memoizing the result of a computation and only recomputing it when the inputs to the computation change. In other words, it allows developers to optimize the performance of their applications by memoizing the results of expensive function calls.
The basic syntax of useMemo is as follows:
const memoizedValue = useMemo(() => computeExpensiveValue(a, b), [a, b]);
Here, computeExpensiveValue
is the function whose result we want to memoize, and [a, b]
is the array of dependencies. The memoized value is then stored and only recalculated if any of the dependencies (a
or b
in this case) change.
Use Case: Expensive Calculations
Let’s explore a practical example to illustrate the benefits of useMemo. Consider a React component that calculates the factorial of a given number:
import React, { useState, useMemo } from 'react';
const FactorialCalculator = ({ number }) => {
const calculateFactorial = (num) => {
let result = 1;
for (let i = 1; i <= num; i++) {
result *= i;
}
return result;
};
const factorial = useMemo(() => calculateFactorial(number), [number]);
return (
<div>
<p>{`Factorial of ${number}: ${factorial}`}</p>
</div>
);
};
In this example, the calculateFactorial
function is potentially an expensive computation, especially for larger numbers. Without the use of useMemo, the factorial would be recalculated every time the component renders, even if the number
prop remains the same. By wrapping the calculation in useMemo with [number]
as a dependency, the computation is only performed when number
changes, preventing unnecessary recalculations.
Performance Optimization in List Rendering
Another common use case for useMemo is in optimizing the rendering of lists, where each item requires a time-consuming computation. Let’s imagine a scenario where a component renders a list of items, and each item requires a complex and resource-intensive calculation:
import React, { useMemo } from 'react';
const ItemList = ({ items }) => {
const calculateItemValue = (item) => {
// Complex and expensive calculation based on item data
// ...
return /* result of computation */;
};
const memoizedItems = useMemo(
() => items.map((item) => calculateItemValue(item)),
[items]
);
return (
<ul>
{memoizedItems.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
};
By utilizing useMemo, the expensive calculations for each item are only performed when the items
array changes. This prevents redundant computations and significantly improves the performance of the component, especially in scenarios with long lists.
Caveats and Considerations
While useMemo is a powerful tool for optimizing performance, it’s essential to use it judiciously. Memoization introduces some overhead, and in certain cases, the cost of maintaining the memoized value may outweigh the benefits, particularly for simple or fast calculations.
Developers should also be cautious when memoizing functions with dependencies that are objects or arrays, as shallow comparisons are performed to determine if the values have changed. In such cases, consider using libraries like lodash.isEqual
for deep equality checks.
Conclusion
The useMemo hook in React is a valuable addition to the developer’s toolkit, offering a means to optimize the performance of applications by memoizing the results of expensive computations. By selectively recalculating values only when their dependencies change, developers can enhance the efficiency of their components, especially in scenarios involving complex calculations or list rendering.
When used appropriately, useMemo contributes to a smoother and more responsive user experience, making it an indispensable tool for React developers seeking to strike a balance between functionality and performance in their applications. As always, understanding the specific use cases and applying useMemo judiciously will empower developers to create high-performance React applications.
How to implement a card flip animation with Bootstrap
How to use Bootstrap’s table-responsive class
How to create a responsive login form with Bootstrap
How to use Bootstrap’s media objects
How does React handle conditional rendering
What is the significance of the useEffect hook in functional components