What is the significance of the useEffect hook in functional components
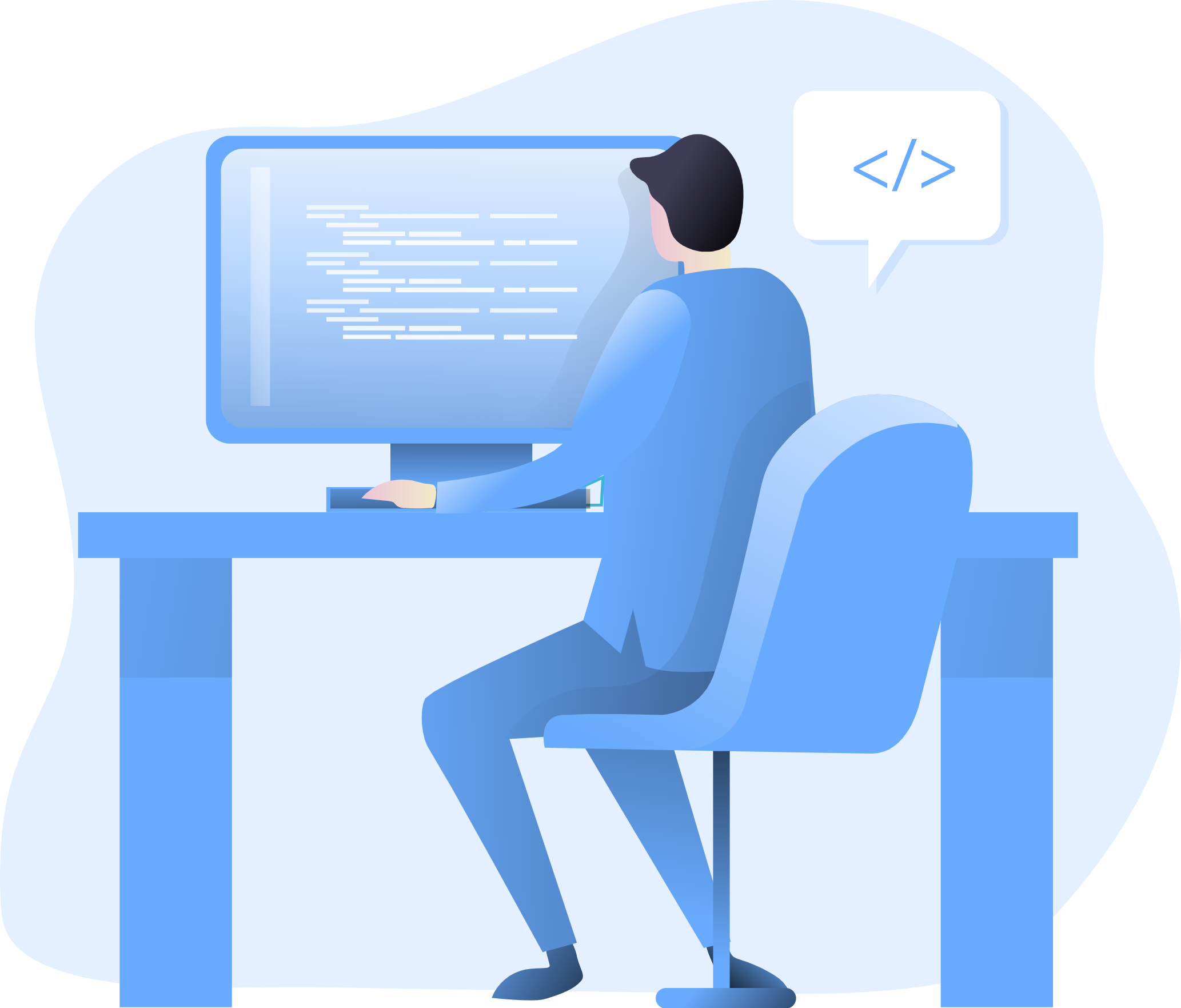
React’s functional components have undergone a transformative evolution with the introduction of hooks, among which the useEffect
hook stands out as a cornerstone. This powerful hook addresses the lifecycle and side effect management in functional components, unlocking a myriad of possibilities for handling asynchronous operations, subscriptions, and other essential tasks. In this comprehensive exploration, we delve into the significance of the useEffect
hook, unraveling its capabilities and showcasing its pivotal role in React development.
Understanding Functional Component Lifecycle
In the realm of class components, developers were accustomed to lifecycle methods like componentDidMount
, componentDidUpdate
, and componentWillUnmount
to manage side effects and asynchronous operations. However, functional components, introduced in React 16.8, lacked these lifecycle methods.
The useEffect
hook emerged as a robust solution to address this gap, enabling developers to manage side effects in functional components with unparalleled flexibility.
The Basics of useEffect
The useEffect
hook allows developers to perform side effects in functional components. Side effects encompass actions such as data fetching, subscriptions, manual DOM manipulations, or any operation that involves interactions with the outside world.
Basic Syntax:
import React, { useEffect } from 'react';
const MyComponent = () => {
useEffect(() => {
// Side effect code goes here
console.log('Effect executed');
}, []); // Dependency array - empty means effect runs once after initial render
return <p>Hello, useEffect!</p>;
};
In this example, the useEffect
hook takes two arguments: a function containing the side effect code and a dependency array. The function will be executed after every render, and the dependency array dictates when the effect should run.
useEffect Lifecycle Equivalent
Understanding the lifecycle equivalence of useEffect
helps bridge the gap between class and functional components.
- componentDidMount: Runs after the initial render.
useEffect(() => {
// componentDidMount logic
}, []);
- componentDidUpdate: Runs after every update.
useEffect(() => {
// componentDidUpdate logic
});
- componentWillUnmount: Cleans up before the component unmounts.
useEffect(() => {
return () => {
// componentWillUnmount logic
};
}, []);
Significance of useEffect in Functional Components
1. Managing Side Effects
The primary purpose of the useEffect
hook is to manage side effects seamlessly. This includes data fetching, subscriptions, or any operation that extends beyond the component’s render.
useEffect(() => {
// Data fetching logic
const fetchData = async () => {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
};
fetchData();
}, []);
2. Dependency Management
The dependency array in useEffect
allows developers to control when the effect should run. By specifying dependencies, you ensure that the effect runs only when those dependencies change.
const MyComponent = ({ userId }) => {
useEffect(() => {
// Fetch user data when userId changes
const fetchUserData = async () => {
const response = await fetch(`https://api.example.com/user/${userId}`);
const userData = await response.json();
console.log(userData);
};
fetchUserData();
}, [userId]);
};
This fine-grained control is crucial for optimizing performance and avoiding unnecessary side effects.
3. Cleanup Operations
The useEffect
hook allows for cleanup operations, especially useful when dealing with subscriptions or resource management. The cleanup function runs before the component unmounts or before the next effect is executed.
useEffect(() => {
const subscription = subscribeToData();
return () => {
// Cleanup logic (unsubscribe, clear intervals, etc.)
subscription.unsubscribe();
};
}, [/* dependencies */]);
This ensures that resources are properly released, preventing memory leaks.
4. Asynchronous Operations
Managing asynchronous code is simplified with useEffect
. You can use asynchronous functions directly within the effect, making it concise and readable.
useEffect(() => {
const fetchData = async () => {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error fetching data:', error);
}
};
fetchData();
}, []);
This asynchronous syntax is more concise and readable compared to traditional approaches.
5. Dynamic DOM Manipulation
For operations involving the DOM, such as setting document titles or manipulating elements, useEffect
comes in handy. It ensures that these operations occur after the render, preventing interference with the rendering process.
useEffect(() => {
document.title = 'Dynamic Page Title';
}, []);
This is especially beneficial when dealing with dynamic content updates.
6. Global State Integration
The useEffect
hook seamlessly integrates with global state management libraries like Redux. It allows components to react to changes in the global state and perform corresponding side effects.
import { useDispatch, useSelector } from 'react-redux';
const MyComponent = () => {
const dispatch = useDispatch();
const data = useSelector(state => state.data);
useEffect(() => {
// Perform side effects based on global state
console.log('Global state changed:', data);
}, [data]);
};
This enables efficient communication between components and the global state.
7. Fetching Data on Mount
Fetching data when a component mounts is a common use case, and useEffect
simplifies this process by executing the effect after the initial render.
useEffect(() => {
const fetchData = async () => {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
};
fetchData();
}, []);
This ensures that data is fetched as soon as the component is mounted.
8. Preventing Infinite Loops
Without proper dependency management, a component might enter into an infinite loop of rendering and effect execution. The dependency array in useEffect
helps prevent this by specifying the conditions under which the effect should run.
useEffect(() => {
// Effect logic
// This effect will only run once after the initial render
}, []);
By providing an empty dependency array, you ensure that the effect runs once after the initial render.
Conclusion: Harnessing the Power of useEffect
The useEffect
hook in React functional components serves as a versatile and powerful tool for managing side effects, asynchronous operations, and the component lifecycle. Its flexibility, combined with the ability to handle dependencies and cleanup operations, makes it an indispensable part of modern React development.
Understanding the significance of useEffect
empowers developers to create efficient, responsive, and scalable applications. Whether dealing with data fetching, dynamic DOM manipulations, or integrating with global state management, the useEffect
hook plays a pivotal role in orchestrating these operations within the functional component paradigm.
As you embark on your React journey, mastering the intricacies of useEffect
will undoubtedly enhance your ability to create sophisticated and performant applications in the dynamic landscape of web development.
How do you handle events in React
What are controlled components in React
How does React handle conditional rendering
How do I create a custom plugin in Tailwind CSS
How can I integrate Tailwind CSS with a CSS-in-JS solution like Emotion or Styled Components
How to use Bootstrap’s table-responsive class
How to create a responsive login form with Bootstrap