How does React handle memoization
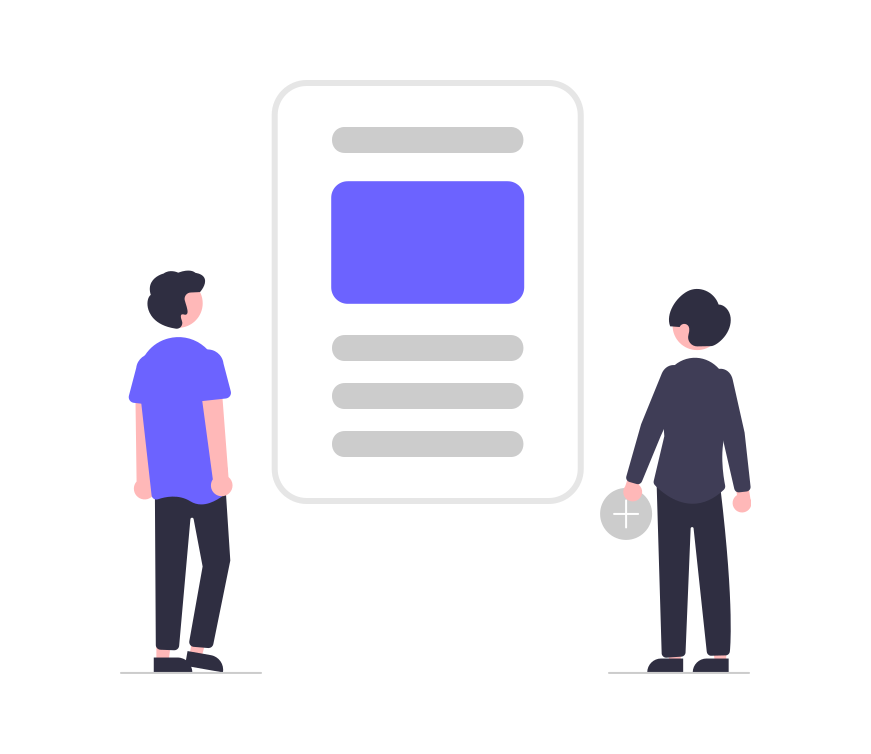
In the dynamic landscape of web development, crafting efficient and high-performance React applications is a top priority. React, a popular JavaScript library developed by Facebook, employs various optimization techniques to enhance rendering speed and minimize unnecessary computations. One such technique is memoization, a process that significantly contributes to React’s ability to efficiently handle components and improve overall application performance.
Understanding Memoization in React
Memoization is a concept deeply rooted in computer science, and in the context of React, it refers to the optimization strategy of storing the results of expensive function calls and returning the cached result when the same inputs occur again. React utilizes memoization to prevent unnecessary rendering of components and to enhance the overall responsiveness of applications.
Memoization and Functional Components
In React, functional components are the building blocks of user interfaces. Prior to React 16.3, functional components were stateless and lacked the ability to manage local state or lifecycle methods. However, with the introduction of hooks, particularly the useState
and useEffect
hooks, functional components gained the capability to manage state and perform side effects.
When it comes to memoization in functional components, React provides the React.memo
higher-order component (HOC) to wrap a component and memoize its result. This means that if the component’s props remain unchanged, React can skip the rendering process, optimizing performance by avoiding unnecessary re-renders.
import React from 'react';
const MemoizedComponent = React.memo(({ prop1, prop2 }) => {
// Component logic here
return <div>{prop1} - {prop2}</div>;
});
In this example, the MemoizedComponent
will only re-render if either prop1
or prop2
changes. If the props remain the same, React will reuse the previously memoized result.
Memoization and Hooks
Hooks, introduced in React 16.8, revolutionized state and side effect management in functional components. The most commonly used hooks include useState
, useEffect
, useContext
, and useReducer
. When it comes to memoization, the useMemo
and useCallback
hooks take center stage.
useMemo
The useMemo
hook allows you to memoize the result of expensive calculations within a component. It takes a function and an array of dependencies, and it will only recompute the memoized value when the dependencies change.
import React, { useMemo } from 'react';
const MemoizedComponent = ({ prop1, prop2 }) => {
const result = useMemo(() => {
// Expensive calculation based on prop1 and prop2
return prop1 + prop2;
}, [prop1, prop2]);
return <div>{result}</div>;
};
Here, the result of the expensive calculation is memoized, and the calculation will only be recomputed if either prop1
or prop2
changes.
useCallback
The useCallback
hook is specifically designed for memoizing functions. It is particularly useful when passing functions to child components to prevent unnecessary re-renders.
import React, { useCallback } from 'react';
const ParentComponent = () => {
const handleClick = useCallback(() => {
// Handle click logic
}, []);
return <ChildComponent onClick={handleClick} />;
};
const ChildComponent = React.memo(({ onClick }) => {
// Component logic here
return <button onClick={onClick}>Click me</button>;
});
In this example, the handleClick
function is memoized using useCallback
, ensuring that the function reference remains the same unless dependencies change. This prevents unnecessary re-renders of the ChildComponent
.
Context and Memoization
React’s context API provides a way to pass data through the component tree without manually passing props at each level. When using context in conjunction with memoization, it’s crucial to consider how changes in context values affect memoized components.
import React, { createContext, useContext } from 'react';
const MyContext = createContext();
const ParentComponent = () => {
const contextValue = 'Context Value';
return (
<MyContext.Provider value={contextValue}>
<MemoizedChildComponent />
</MyContext.Provider>
);
};
const MemoizedChildComponent = React.memo(() => {
const contextValue = useContext(MyContext);
return <div>{contextValue}</div>;
});
In this example, the MemoizedChildComponent
will only re-render if the contextValue
changes. If the context value remains the same, the component will reuse the memoized result.
When to Use Memoization
While memoization is a powerful tool for optimizing React applications, it’s essential to use it judiciously. Applying memoization to every component or function can lead to unnecessary complexity and hinder development speed. Here are some scenarios where memoization is particularly beneficial:
- Expensive Computations: Memoization is most effective when dealing with computations that are resource-intensive or time-consuming. By memoizing the results, you prevent unnecessary recalculations.
- Preventing Unnecessary Renders: Memoization shines when you want to avoid unnecessary renders of components. By memoizing components or functions, React can skip rendering if the input values (props or dependencies) remain the same.
- Optimizing Callbacks: When passing callbacks to child components, using
useCallback
ensures that the function reference remains constant, preventing child components from re-rendering unnecessarily.
Challenges and Considerations
While memoization is a powerful optimization strategy, it’s essential to be aware of potential challenges and considerations:
- Equality of Dependencies: React relies on the equality of dependencies to determine whether to recompute memoized values. If dependencies are objects or arrays, make sure to handle equality correctly.
- Nested Memoization: Avoid unnecessary nested memoization. In some cases, deeply nested memoization can lead to performance issues. Only memoize what is necessary for the specific use case.
- Trade-offs: Memoization involves a trade-off between memory and computation. While it can save computational resources, it also consumes memory to store the memoized results. Consider the balance based on the nature of your application.
Conclusion
In the dynamic world of React, where performance is a critical consideration, memoization emerges as a powerful tool for optimizing components and functions. Whether through the use of React.memo
, useMemo
, or useCallback
, understanding and strategically applying memoization techniques can significantly contribute to the responsiveness and efficiency of your React applications.
As you embark on your React development journey, consider memoization as a valuable ally in your quest for high-performance web applications. By mastering the art of memoization, you’ll not only enhance the user experience but also gain insights into the nuanced intricacies of optimizing React components for peak efficiency.
What are controlled components in React
How does React handle conditional rendering
What is the significance of the useEffect hook in functional components
How to use Bootstrap’s utility classes for flexbox alignment
How does React differ from other JavaScript frameworks
How do I install Tailwind CSS in my project
How do I integrate Tailwind CSS with a JavaScript framework like React or Vue.js