How does React handle routing
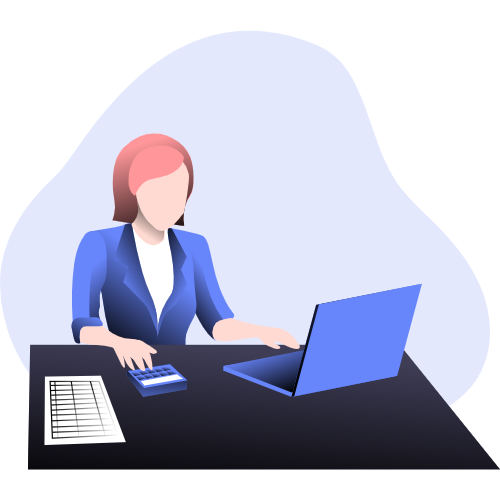
In the ever-evolving landscape of web development, building single-page applications (SPAs) has become increasingly popular. SPAs provide a seamless user experience by loading a single HTML page and dynamically updating content as the user interacts with the application. React, a JavaScript library developed by Facebook, is a powerful tool for building SPAs, and one crucial aspect of creating a smooth user experience is navigation within the application.
Introduction to React Routing
Routing is the process of determining which components to display based on the user’s interaction with the application. In traditional multi-page applications, navigation is handled by the browser, which reloads the entire page when a new URL is visited. However, SPAs require a different approach to provide a fluid user experience without the need for full-page reloads.
React Router, a popular library for handling navigation in React applications, allows developers to create a navigation structure similar to what you would find in a multi-page application but within the confines of a single page. It enables the creation of a navigation flow that updates the URL and renders different components based on the URL, all without triggering a full page reload.
Installation of React Router
Before delving into how React handles routing, it’s essential to set up React Router in your project. You can install it using npm or yarn:
npm install react-router-dom
or
yarn add react-router-dom
Once installed, you need to import the necessary components and wrap your application in the BrowserRouter
component, which provides the routing functionality:
import React from 'react';
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
const App = () => {
return (
<Router>
{/* Your application components go here */}
</Router>
);
};
export default App;
Basic Concepts of React Router
Route Component
The Route
component is a fundamental building block of React Router. It renders UI components based on the URL. The path
prop in the Route
component specifies the URL path that should trigger the rendering of the associated component.
import React from 'react';
import { Route } from 'react-router-dom';
const Home = () => <div>Home Page</div>;
const App = () => {
return (
<Router>
<Route path="/" component={Home} />
</Router>
);
};
export default App;
In this example, when the URL matches ‘/’, the Home
component will be rendered.
Switch Component
The Switch
component is used to render only the first Route
that matches the current location. This is particularly useful when you have multiple routes defined, and you want to ensure that only one route is matched.
import React from 'react';
import { Route, Switch } from 'react-router-dom';
const Home = () => <div>Home Page</div>;
const About = () => <div>About Page</div>;
const App = () => {
return (
<Router>
<Switch>
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
</Switch>
</Router>
);
};
export default App;
In this example, the exact
prop ensures that only the exact path match triggers the rendering of the Home
component.
Link Component
To create navigation links within your application, React Router provides the Link
component. It works similarly to an anchor (<a>
) tag but prevents the browser from reloading the entire page.
import React from 'react';
import { Link } from 'react-router-dom';
const Navigation = () => {
return (
<nav>
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
</ul>
</nav>
);
};
const App = () => {
return (
<Router>
<Navigation />
<Switch>
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
</Switch>
</Router>
);
};
export default App;
The to
prop in the Link
component specifies the path to navigate to.
Dynamic Routing and Route Parameters
React Router allows for dynamic routing by using parameters in the URL. Parameters are specified in the Route
component using a colon (:
) followed by the parameter name.
import React from 'react';
import { Route } from 'react-router-dom';
const UserProfile = ({ match }) => {
const { username } = match.params;
return <div>User Profile: {username}</div>;
};
const App = () => {
return (
<Router>
<Route path="/profile/:username" component={UserProfile} />
</Router>
);
};
export default App;
In this example, accessing a URL like /profile/johndoe
would render the UserProfile
component with the username parameter set to ‘johndoe’.
Programmatic Navigation
React Router also provides a useHistory
hook and a history
object, which allows for programmatic navigation, such as redirecting users or navigating based on application logic.
import React from 'react';
import { useHistory } from 'react-router-dom';
const Login = () => {
const history = useHistory();
const handleLogin = () => {
// Perform login logic
// Redirect to the home page after login
history.push('/');
};
return (
<div>
<h2>Login Page</h2>
<button onClick={handleLogin}>Login</button>
</div>
);
};
const App = () => {
return (
<Router>
<Switch>
<Route path="/login" component={Login} />
<Route path="/" exact component={Home} />
</Switch>
</Router>
);
};
export default App;
In this example, clicking the “Login” button triggers the handleLogin
function, which uses the history.push('/')
method to navigate to the home page.
Route Guards and Navigation Hooks
React Router provides additional features for route guarding and navigation hooks. Route guards allow developers to control access to certain routes based on conditions, while navigation hooks enable the execution of logic before and after navigation events.
import React from 'react';
import { Route, Redirect, useHistory } from 'react-router-dom';
const PrivateRoute = ({ component: Component, ...rest }) => {
const isAuthenticated = /* logic to check if the user is authenticated */;
return (
<Route
{...rest}
render={(props) =>
isAuthenticated ? (
<Component {...props} />
) : (
<Redirect to="/login" />
)
}
/>
);
};
const Dashboard = () => <div>Dashboard Page</div>;
const App = () => {
return (
<Router>
<Switch>
<PrivateRoute path="/dashboard" component={Dashboard} />
<Route path="/login" component={Login} />
<Route path="/" exact component={Home} />
</Switch>
</Router>
);
};
export default App;
``
`
In this example, the `PrivateRoute` component acts as a route guard, redirecting unauthenticated users to the login page.
## Conclusion
React Router plays a crucial role in handling navigation within React applications, allowing developers to create SPAs with a smooth and seamless user experience. By providing components like `Route`, `Switch`, `Link`, and hooks like `useHistory`, React Router simplifies the process of managing navigation, enabling the creation of complex and dynamic single-page applications.
Understanding the basics of React Router is essential for React developers, as it provides the foundation for building modern, interactive web applications. As the React ecosystem continues to evolve, React Router remains a fundamental tool for managing navigation and enhancing the overall user experience in React applications.
How do I handle dark mode in Tailwind CSS
How can I add a new font to my Tailwind CSS project
What is the purpose of the “purge” option in the Tailwind CSS configuration
How to create a responsive form layout with Bootstrap
How to implement a parallax scrolling effect with Bootstrap
Explain the concept of lifting state up in React