How can you handle state management in a Next.js application
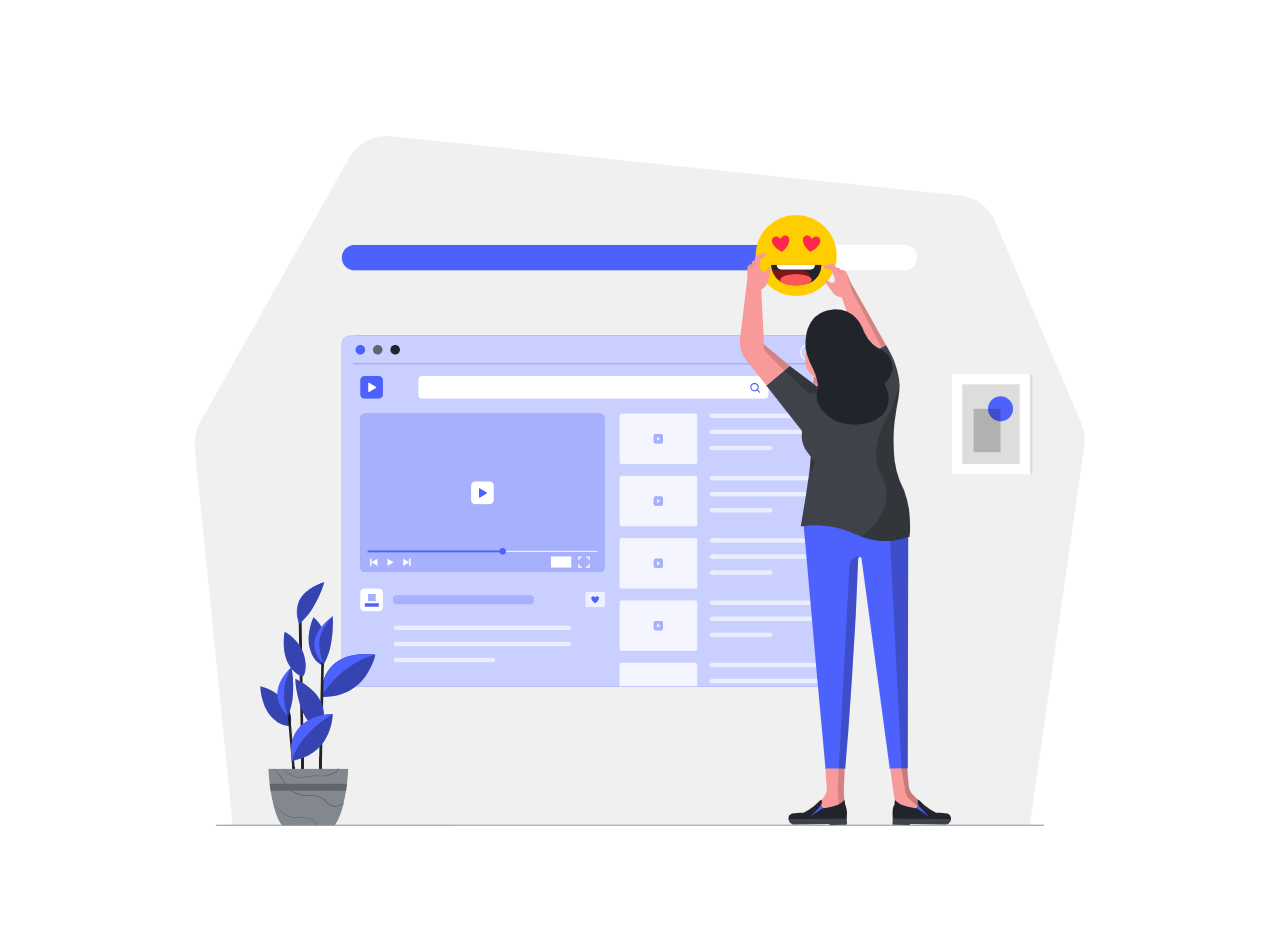
State management is a crucial aspect of building robust web applications, including those developed using Next.js. With Next.js’s flexible architecture and support for server-side rendering (SSR) and client-side rendering (CSR), choosing the right state management approach is essential for ensuring scalability, maintainability, and performance. In this comprehensive guide, we’ll explore various techniques and best practices for handling state management in Next.js applications.
Understanding State Management
Before delving into specific state management strategies in Next.js, let’s briefly discuss the concept of state management itself. In web development, state refers to the data that represents the current condition or context of an application. State management involves how this data is stored, updated, and accessed throughout the application’s lifecycle.
State can be categorized into two main types:
- Local State: State that is confined to a specific component or page and does not need to be shared with other parts of the application.
- Global State: State that needs to be accessed and modified by multiple components or pages within the application.
Next.js applications commonly require both local and global state management solutions to handle various requirements effectively.
Local State Management
1. React’s useState Hook
Next.js, being built on top of React, leverages React’s state management capabilities. The useState
hook allows components to manage their local state in a straightforward manner. This is suitable for simple components or when the state does not need to be shared with other components.
import React, { useState } from 'react';
const MyComponent = () => {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
};
export default MyComponent;
2. React Context
React Context is a powerful tool for sharing global state across multiple components without prop drilling. In Next.js applications, React Context can be used to manage global state that needs to be accessed by various components, such as user authentication status or theme preferences.
import React, { createContext, useContext, useState } from 'react';
const MyContext = createContext();
export const useMyContext = () => useContext(MyContext);
export const MyContextProvider = ({ children }) => {
const [theme, setTheme] = useState('light');
const toggleTheme = () => {
setTheme(theme === 'light' ? 'dark' : 'light');
};
return (
<MyContext.Provider value={{ theme, toggleTheme }}>
{children}
</MyContext.Provider>
);
};
Global State Management
1. Redux
Redux is a popular state management library that provides a predictable state container for JavaScript applications. It’s particularly useful for managing complex global state that needs to be shared across multiple components or pages in a Next.js application.
npm install redux react-redux
Redux follows a unidirectional data flow architecture, making it easier to understand and debug application state changes. Actions are dispatched to update the state, and components subscribe to the state changes they’re interested in.
2. MobX
MobX is another state management library that offers a more flexible and reactive approach compared to Redux. It allows you to define observable state and automatically track dependencies, making it easier to manage state updates in Next.js applications.
npm install mobx mobx-react
MobX simplifies state management by directly annotating the state with observables and automatically updating components when the state changes. It’s particularly suitable for applications with complex data flows or frequent state updates.
Integrating State Management with Next.js
Once you’ve chosen the appropriate state management solution for your Next.js application, integrating it is straightforward. For global state management libraries like Redux or MobX, you can initialize the state container in the root of your application and wrap your components with providers to access the global state.
// pages/_app.js
import { Provider } from 'react-redux';
import { store } from '../redux/store'; // Redux store configuration
function MyApp({ Component, pageProps }) {
return (
<Provider store={store}>
<Component {...pageProps} />
</Provider>
);
}
export default MyApp;
For React Context-based solutions, you can wrap your components with context providers similarly to how it’s done with Redux or MobX.
Conclusion
State management is a fundamental aspect of building scalable and maintainable web applications, and Next.js offers a variety of options for handling state effectively. By understanding the different state management techniques available, you can choose the approach that best fits your application’s requirements and development preferences. Whether it’s managing local state with React hooks or implementing a global state container with Redux or MobX, Next.js provides the flexibility and tools necessary to build powerful and efficient web applications.
How do I create a custom plugin in Tailwind CSS
How can I integrate Tailwind CSS with a CSS-in-JS solution like Emotion or Styled Components
What is the difference between the minified and unminified versions of Tailwind CSS
How do I install Tailwind CSS in my project
How do I integrate Tailwind CSS with a JavaScript framework like React or Vue.js
How do I create a responsive layout with Tailwind CSS
How do I vertically center an element in Tailwind CSS
How do I customize the spacing like padding and margin between elements in Tailwind CSS