How can you handle form submissions in a Next.js application
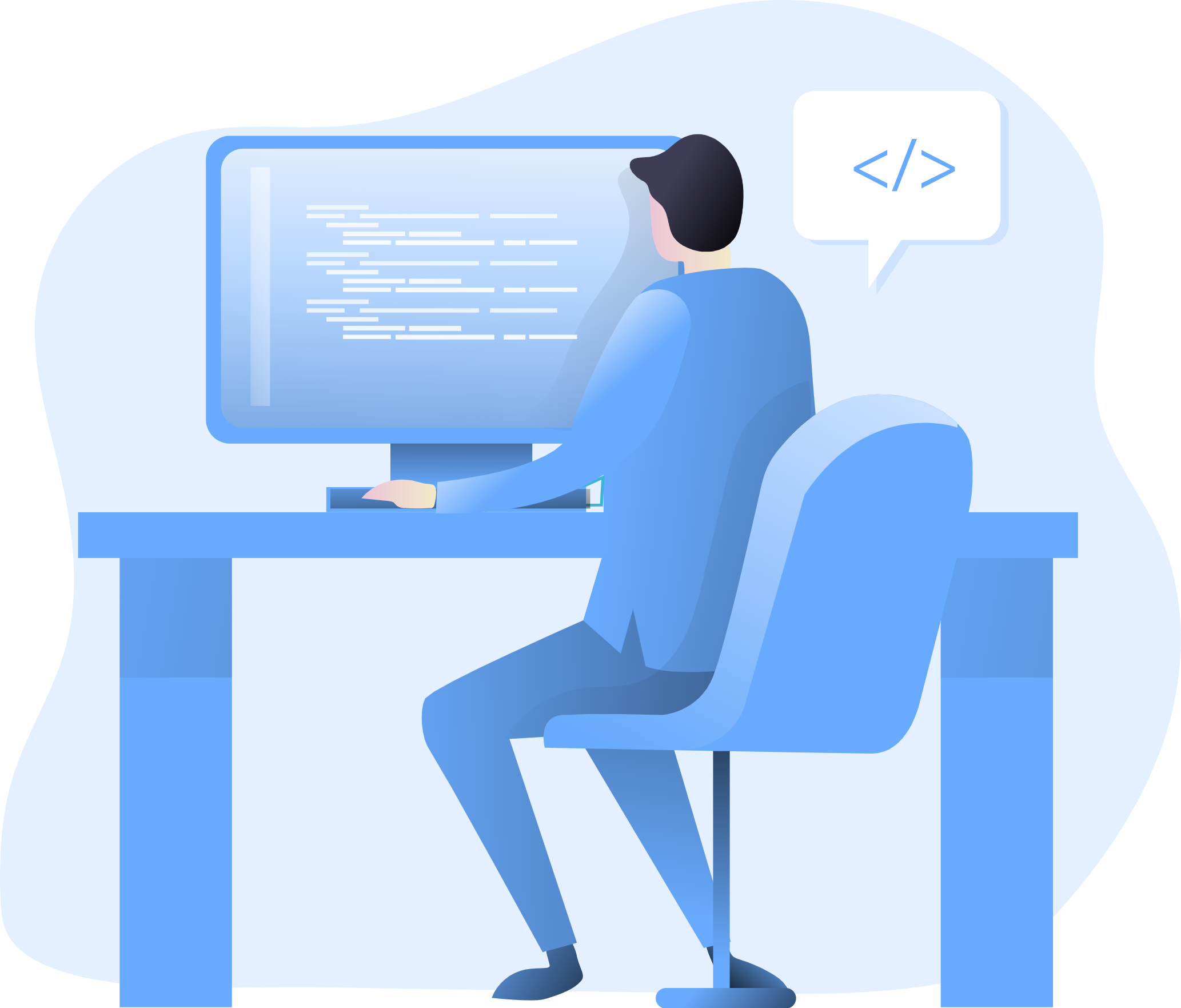
Forms are an integral part of web development, facilitating user interaction and data input. In a Next.js application, handling form submissions efficiently is crucial for creating seamless user experiences. In this guide, we’ll explore various strategies and best practices for handling form submissions in a Next.js application.
Basic Form Setup
Before diving into form submission handling, let’s start with setting up a basic form in a Next.js component. Here’s a simple example of a form component in Next.js:
import React, { useState } from 'react';
const MyForm = () => {
const [formData, setFormData] = useState({
email: '',
password: '',
});
const handleChange = (e) => {
const { name, value } = e.target;
setFormData((prevData) => ({
...prevData,
[name]: value,
}));
};
const handleSubmit = (e) => {
e.preventDefault();
// Handle form submission logic here
console.log(formData);
};
return (
<form onSubmit={handleSubmit}>
<input
type="email"
name="email"
value={formData.email}
onChange={handleChange}
placeholder="Email"
/>
<input
type="password"
name="password"
value={formData.password}
onChange={handleChange}
placeholder="Password"
/>
<button type="submit">Submit</button>
</form>
);
};
export default MyForm;
This basic form component captures user input for email and password fields and logs the form data upon submission.
Handling Form Submission
1. Client-Side Handling
For client-side form handling, you can utilize JavaScript events like onSubmit
to intercept form submissions before they trigger a page reload. In the example above, handleSubmit
function prevents the default form submission behavior using e.preventDefault()
and then performs any necessary logic, such as data validation or sending data to an API.
2. Server-Side Handling
In cases where server-side processing is required, such as authentication or saving form data to a database, Next.js provides several options:
a. API Routes
Next.js allows you to create API routes within your application using the /pages/api
directory. You can define server-side logic for handling form submissions in these API routes, which can then be accessed from your client-side components.
Example of an API route for handling form submissions (pages/api/login.js
):
// pages/api/login.js
export default function handler(req, res) {
if (req.method === 'POST') {
// Handle form submission logic here
const { email, password } = req.body;
console.log('Received form data:', { email, password });
// Perform authentication, database operations, etc.
res.status(200).json({ message: 'Form submitted successfully' });
} else {
res.status(405).json({ message: 'Method Not Allowed' });
}
}
In your form component, you can then make a POST request to this API route to handle form submissions.
b. External APIs
For more complex scenarios or integrations with external services, you can make HTTP requests directly from your Next.js components using libraries like fetch
or Axios.
import { useState } from 'react';
const MyForm = () => {
const [formData, setFormData] = useState({
email: '',
password: '',
});
const handleChange = (e) => {
const { name, value } = e.target;
setFormData((prevData) => ({
...prevData,
[name]: value,
}));
};
const handleSubmit = async (e) => {
e.preventDefault();
try {
const response = await fetch('/api/login', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(formData),
});
if (response.ok) {
console.log('Form submitted successfully');
} else {
console.error('Form submission failed');
}
} catch (error) {
console.error('Error submitting form:', error);
}
};
return (
<form onSubmit={handleSubmit}>
<input
type="email"
name="email"
value={formData.email}
onChange={handleChange}
placeholder="Email"
/>
<input
type="password"
name="password"
value={formData.password}
onChange={handleChange}
placeholder="Password"
/>
<button type="submit">Submit</button>
</form>
);
};
export default MyForm;
Conclusion
Handling form submissions in a Next.js application involves a combination of client-side and server-side techniques, depending on the requirements of your application. By following best practices and leveraging Next.js features such as API routes, you can efficiently manage form data, perform necessary processing, and create seamless user experiences. Whether it’s authentication, data validation, or integration with external services, Next.js provides the flexibility and tools needed to handle form submissions effectively.
How to create a responsive pricing table with Bootstrap
How to implement a split-screen layout with Bootstrap
How to implement a card flip animation with Bootstrap
How to use Bootstrap’s table-responsive class
How to create a responsive login form with Bootstrap
How to use Bootstrap’s media objects
How to integrate Bootstrap with JavaScript libraries like jQuery
How to implement a modal/popup with Bootstrap