How can you implement a loading spinner for data fetching in Next.js
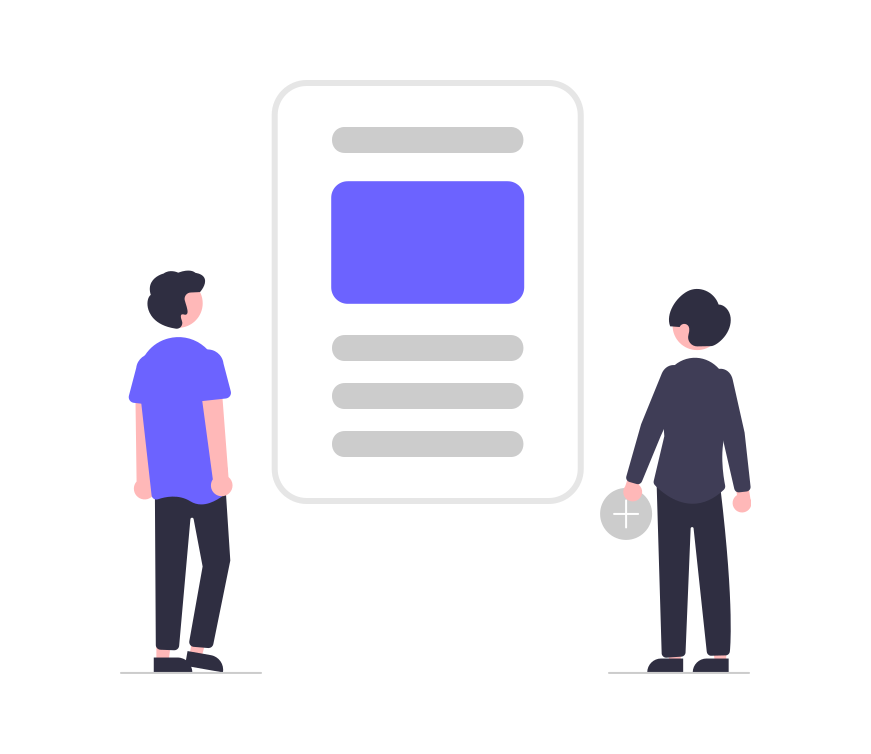
In modern web development, providing a smooth and responsive user experience is paramount. When dealing with data fetching operations, especially in Next.js applications, it’s crucial to handle loading states gracefully. One effective way to enhance user experience during data fetching is by implementing a loading spinner. In this article, we’ll explore how to integrate a loading spinner into a Next.js application to indicate ongoing data fetching processes.
Understanding Next.js Data Fetching
Next.js offers various methods for fetching data, including Static Generation (SG), Server-Side Rendering (SSR), and Client-Side Data Fetching. Each method has its use cases and implications on performance and user experience. However, regardless of the data fetching approach, incorporating loading indicators enhances the perceived performance and usability of your application.
Why Use Loading Spinners
Loading spinners, also known as loaders or spinners, are visual indicators that inform users about ongoing processes, such as data fetching. They provide immediate feedback, indicating to users that the application is working on their request, thus reducing frustration associated with waiting times. Incorporating loading spinners aligns with the principles of user-centered design, contributing to a more satisfying user experience.
Implementing a Loading Spinner in Next.js
1. Design Your Loading Spinner
Before integrating the loading spinner into your Next.js application, design the spinner itself. You can create custom spinners using CSS animations or utilize pre-made spinner libraries like SpinKit or React Spinners. Ensure the spinner’s design aligns with your application’s aesthetics and branding.
2. Define Loading State
Next.js provides various hooks and lifecycle methods for managing component states. Define a loading state variable to track the status of data fetching operations. For instance, you can use React’s useState
hook to manage the loading state:
import React, { useState } from 'react';
const MyComponent = () => {
const [isLoading, setIsLoading] = useState(false);
// Your component logic here
return (
<>
{/* Render loading spinner conditionally */}
{isLoading && <Spinner />}
{/* Rest of your component */}
</>
);
};
export default MyComponent;
3. Toggle Loading State During Data Fetching
When initiating data fetching operations, toggle the loading state to true. This informs the component to render the loading spinner. After data fetching completes, set the loading state back to false to hide the spinner. You can achieve this using asynchronous functions, promises, or React’s useEffect
hook.
import React, { useState, useEffect } from 'react';
import fetchDataFunction from './fetchDataFunction'; // Your data fetching function
const MyComponent = () => {
const [isLoading, setIsLoading] = useState(false);
useEffect(() => {
const fetchData = async () => {
setIsLoading(true);
try {
await fetchDataFunction(); // Call your data fetching function
// Data fetching succeeded
} catch (error) {
// Handle errors
} finally {
setIsLoading(false);
}
};
fetchData();
}, []); // Empty dependency array ensures useEffect runs only once
return (
<>
{/* Render loading spinner conditionally */}
{isLoading && <Spinner />}
{/* Rest of your component */}
</>
);
};
export default MyComponent;
4. Display Loading Spinner Conditionally
Finally, conditionally render the loading spinner based on the loading state. When isLoading
is true, display the loading spinner; otherwise, render the component’s content.
import React, { useState, useEffect } from 'react';
import Spinner from './Spinner'; // Your loading spinner component
import fetchDataFunction from './fetchDataFunction'; // Your data fetching function
const MyComponent = () => {
const [isLoading, setIsLoading] = useState(false);
useEffect(() => {
const fetchData = async () => {
setIsLoading(true);
try {
await fetchDataFunction(); // Call your data fetching function
// Data fetching succeeded
} catch (error) {
// Handle errors
} finally {
setIsLoading(false);
}
};
fetchData();
}, []); // Empty dependency array ensures useEffect runs only once
return (
<>
{/* Render loading spinner conditionally */}
{isLoading && <Spinner />}
{/* Rest of your component */}
</>
);
};
export default MyComponent;
Conclusion
Integrating a loading spinner into your Next.js application significantly improves the user experience during data fetching operations. By following the steps outlined in this article, you can effectively implement a loading spinner, providing users with clear feedback and reducing perceived wait times. Remember to design your spinner to match your application’s aesthetics and ensure seamless integration with Next.js components. Enhance your Next.js applications today by incorporating loading spinners for a smoother user experience.
How does Next.js differ from traditional React applications
How does client-side rendering (CSR) work in Next.js
How does Next.js handle bundling and code splitting
What is incremental static regeneration in Next.js
Can a Next.js application use both server-side rendering and client-side rendering
How does Next.js handle SEO for dynamic pages
How can you implement custom 404 error handling for API routes