What is the purpose of the styled-jsx library in Next.js
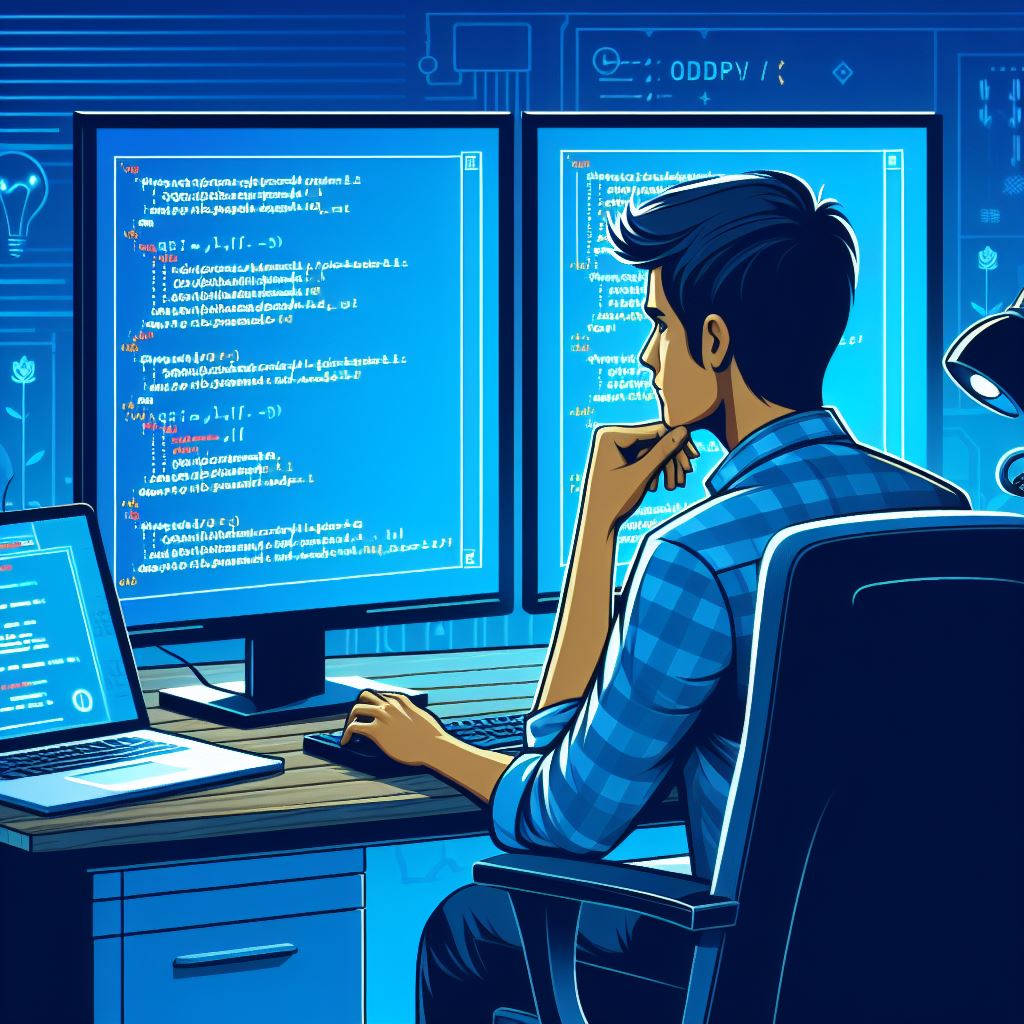
In the realm of web development, creating aesthetically pleasing and maintainable styles for your applications is a crucial aspect. As the complexity of web projects grows, so does the need for efficient and scalable styling solutions. Next.js, a popular React framework for building web applications, addresses this need by integrating the styled-jsx library. In this article, we will delve into the purpose of styled-jsx and how it enhances the styling experience in Next.js.
Understanding Styled-JSX
Styled-JSX is a CSS-in-JS library that comes bundled with Next.js, making it a seamless choice for styling React components in Next.js applications. This library allows developers to write encapsulated and scoped styles directly within their React components. The term “CSS-in-JS” signifies a paradigm where styles are written using JavaScript, providing a way to manage and encapsulate styles at the component level.
Encapsulation and Scope
One of the primary purposes of styled-jsx in Next.js is encapsulation. Traditional CSS styles can sometimes lead to global scope issues, where styles defined for one component unintentionally affect other parts of the application. Styled-JSX addresses this problem by generating unique class names for each component, ensuring that styles are encapsulated and won’t leak into other parts of the application.
Consider the following example:
// Button.js
const Button = () => (
<button>
Click me
<style jsx>{`
button {
background-color: #3498db;
color: #ffffff;
padding: 10px 20px;
border: none;
border-radius: 5px;
cursor: pointer;
}
`}</style>
</button>
);
export default Button;
In this example, the styles defined within the style jsx
block are scoped to the button
element. The class names generated by styled-jsx will only affect this specific button component, preventing unintended style interference elsewhere in the application.
Dynamic Styling and Theming
Styled-JSX shines when it comes to dynamic styling and theming in Next.js applications. React components often need to adapt their styles based on various conditions or user interactions. With styled-jsx, dynamic styling becomes intuitive and maintains the benefits of encapsulation.
Consider the following example where the button’s color changes based on a prop:
// DynamicButton.js
const DynamicButton = ({ primary }) => (
<button>
Click me
<style jsx>{`
button {
background-color: ${primary ? '#3498db' : '#2ecc71'};
color: #ffffff;
padding: 10px 20px;
border: none;
border-radius: 5px;
cursor: pointer;
}
`}</style>
</button>
);
export default DynamicButton;
In this example, the color of the button is determined by the primary
prop. The use of JavaScript within the style block allows for dynamic styles without sacrificing encapsulation. This approach is particularly beneficial in scenarios where components need to adapt to changing states or user interactions.
Global Styles
While styled-jsx encourages component-level encapsulation, there are instances where global styles are necessary. Next.js and styled-jsx provide a solution for this with the global
modifier:
// GlobalStyles.js
const GlobalStyles = () => (
<style jsx global>{`
body {
font-family: 'Helvetica, sans-serif';
background-color: #f8f8f8;
}
// Other global styles
`}</style>
);
export default GlobalStyles;
The global
modifier allows styles to apply globally, affecting all components in the application. This flexibility ensures that developers can strike a balance between component-specific styles and global styling requirements.
Server-Side Rendering (SSR) and Critical CSS
Next.js excels in server-side rendering, and styled-jsx seamlessly integrates with this feature. One of the notable advantages is the ability to generate critical CSS during server-side rendering, enhancing the initial page load performance.
When a Next.js application is rendered on the server, styled-jsx automatically extracts only the critical CSS needed for the initial render. This extracted CSS is then inlined into the HTML document, reducing the amount of CSS that needs to be loaded before the initial render. This results in faster page loads and improved user experience, particularly crucial for performance-sensitive applications.
Conclusion
The styled-jsx library in Next.js serves a crucial role in simplifying and enhancing the styling process for React components. By promoting encapsulation, dynamic styling, theming, and global styles, it provides a comprehensive solution to manage styles at the component level. The integration with server-side rendering further boosts performance by optimizing the delivery of critical CSS.
As web development continues to evolve, tools like styled-jsx empower developers to create maintainable, scalable, and performant applications. Whether you are building a small project or a large-scale application, understanding and leveraging the capabilities of styled-jsx in Next.js can significantly contribute to the success of your web development endeavors.
How does Next.js differ from traditional React applications
How does client-side rendering (CSR) work in Next.js
How does Next.js handle bundling and code splitting
What is incremental static regeneration in Next.js
Can a Next.js application use both server-side rendering and client-side rendering
How does Next.js handle SEO for dynamic pages
How to use Bootstrap’s table-responsive class