How do you implement server-side rendering with React and Express
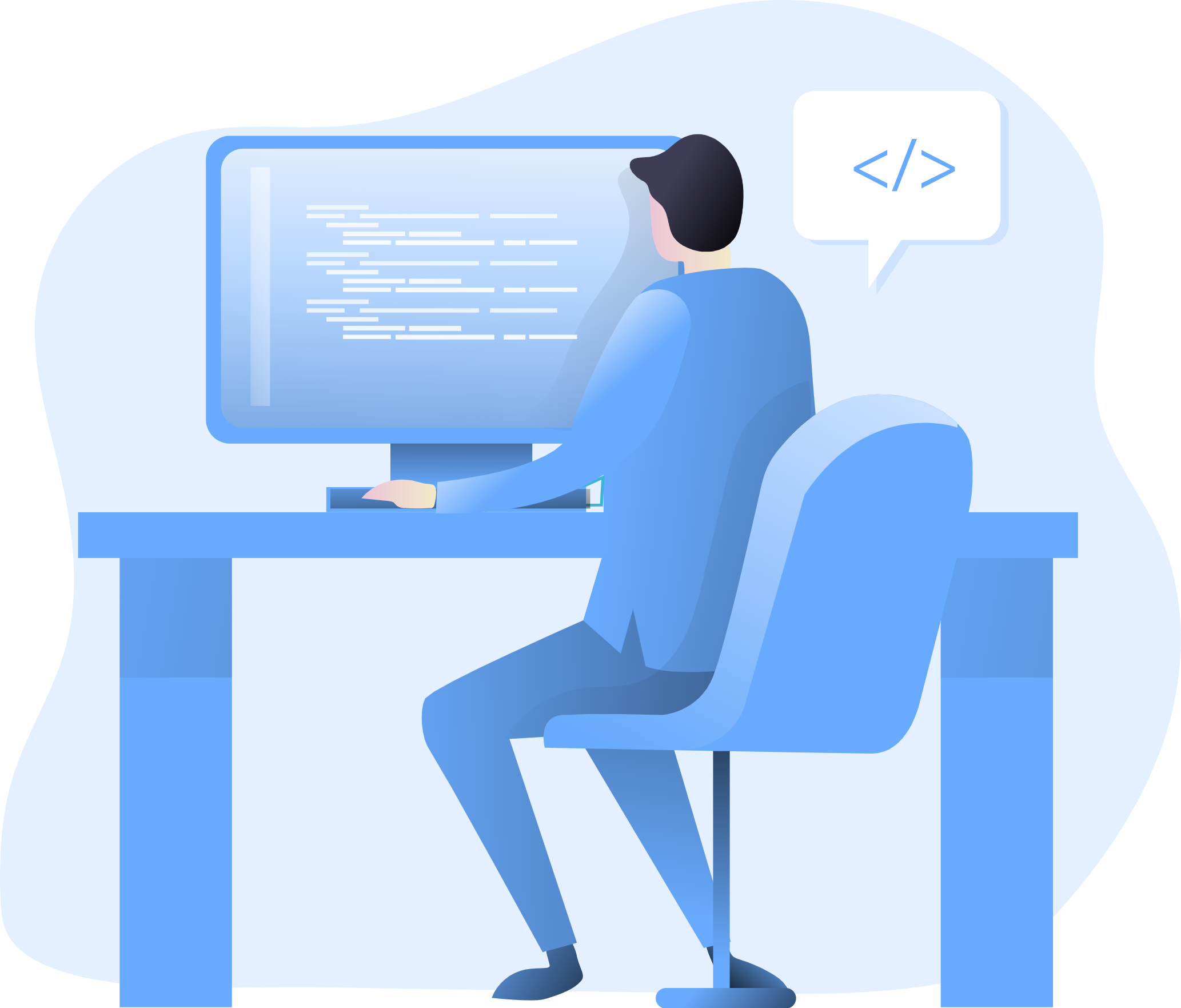
Server-Side Rendering (SSR) is a powerful technique that enhances the performance and SEO of React applications by rendering components on the server before sending them to the client. In this comprehensive guide, we will delve into the intricacies of implementing Server-Side Rendering with React and Express, exploring the benefits, challenges, and step-by-step procedures to empower developers in creating high-performance and SEO-friendly web applications.
Understanding Server-Side Rendering (SSR)
- Overview of SSR in React: Establish the foundational concepts of SSR, emphasizing its advantages in improving initial load times, enhancing SEO, and providing a better user experience.
- Client-Side Rendering vs. Server-Side Rendering: Compare the traditional client-side rendering approach with SSR, highlighting the differences in rendering processes and their impact on performance.
Benefits of Server-Side Rendering
- Improved Performance: Explore how SSR reduces the time to first render, resulting in faster page load times and a more responsive user interface.
- Enhanced SEO: Discuss how SSR contributes to better search engine optimization by providing search engines with pre-rendered HTML content.
- Optimal Initial Load: Illustrate how SSR optimizes the initial load by delivering a fully-rendered page to the client, minimizing the need for subsequent requests.
Setting Up a React Application with Express
- Initializing a React App: Guide developers through creating a basic React application using tools like Create React App or a custom setup.
- Installing and Configuring Express: Demonstrate the installation of Express and the configuration of a basic server to serve the React application.
Integrating Server-Side Rendering with React
-
Setting Up Babel for Server-Side Rendering: Configure Babel to enable the server to transpile JSX and modern JavaScript syntax for server-side rendering.
-
Creating an Express Route for SSR: Define an Express route dedicated to server-side rendering, handling React component rendering and data fetching.
// Express Route for SSR app.get('/', (req, res) => { // SSR logic res.send(html); });
-
Fetching Initial Data on the Server: Illustrate how to fetch initial data on the server before rendering the React components, ensuring a consistent data flow between client and server.
Implementing Server-Side Rendering in React Components
-
Using ReactDOMServer.renderToString: Introduce
ReactDOMServer.renderToString
to render React components to a string on the server, converting them into HTML.// Server-Side Rendering with renderToString const html = ReactDOMServer.renderToString(<App />);
-
Hydrating on the Client Side: Address the need for hydration on the client side to enable interactivity and dynamic behavior after the initial render.
// Client-Side Hydration const rootElement = document.getElementById('root'); ReactDOM.hydrate(<App />, rootElement);
Managing Routes and Navigation
-
Integrating react-router for SSR: Incorporate
react-router
to handle client-side routing and ensure proper navigation in both server-side rendered and client-rendered scenarios.// Server-Side Rendering with react-router const context = {}; const html = ReactDOMServer.renderToString( <StaticRouter location={req.url} context={context}> <App /> </StaticRouter> );
-
Handling Redirects and 404s: Discuss strategies for managing redirects and handling 404 errors in the context of server-side rendering.
Optimizing Performance and Caching
- Implementing Server-Side Caching: Explore techniques for implementing server-side caching to further enhance performance and reduce the load on the server.
- Utilizing CDNs for Static Assets: Discuss the use of Content Delivery Networks (CDNs) to efficiently serve static assets and improve global performance.
Testing Server-Side Rendering
-
Unit Testing SSR Components: Provide insights into unit testing server-side rendered components using testing libraries like Jest and React Testing Library.
// Jest Test for SSR Component test('renders App component on the server', () => { const html = ReactDOMServer.renderToString(<App />); expect(html).toContain('Hello, World!'); });
-
Integration Testing with Puppeteer: Demonstrate how to conduct integration tests using Puppeteer to validate the entire SSR process, including client-side hydration.
// Puppeteer Integration Test test('renders and hydrates App component on the client', async () => { const browser = await puppeteer.launch(); const page = await browser.newPage(); const response = await page.goto('http://localhost:3000/'); const html = await page.content(); // Validate HTML content and client-side hydration expect(html).toContain('Hello, World!'); await browser.close(); });
Troubleshooting Common Issues
- Debugging SSR Errors: Offer guidance on debugging common SSR issues, including mismatched server and client states, and resolving common error scenarios.
- Handling Asynchronous Operations: Discuss strategies for handling asynchronous operations during SSR, such as data fetching, to ensure a smooth rendering process.
Deployment Considerations
- Configuring Server for Production: Guide developers through configuring the Express server for production, including setting up environment variables, security measures, and performance optimizations.
- Choosing Hosting Services: Discuss considerations for choosing hosting services that support Node.js and provide scalability for server-side rendered React applications.
SEO Considerations with Server-Side Rendering
- Ensuring SEO-Friendly Routes: Address the importance of creating SEO-friendly routes with server-side rendering, emphasizing the role of meaningful URLs and metadata for search engine optimization.
- Optimizing for Search Engine Crawlers: Discuss techniques for optimizing server-side rendering to ensure search engine crawlers can efficiently index and understand the content.
Conclusion
Server-Side Rendering with React and Express is a transformative technique that elevates the performance and search engine visibility of web applications. By mastering the intricacies of SSR, developers can create blazing-fast, SEO-friendly applications that provide users with a seamless and engaging experience. This comprehensive guide serves as a roadmap for implementing SSR with React and Express, empowering developers to harness the full potential of server-side rendering in their projects.
How to implement a split-screen layout with Bootstrap
How to implement a card flip animation with Bootstrap
How to use Bootstrap’s table-responsive class
How to create a responsive login form with Bootstrap
How to use Bootstrap’s media objects
How to integrate Bootstrap with JavaScript libraries like jQuery
How to implement a modal/popup with Bootstrap