How do you pass data between parent and child components in React
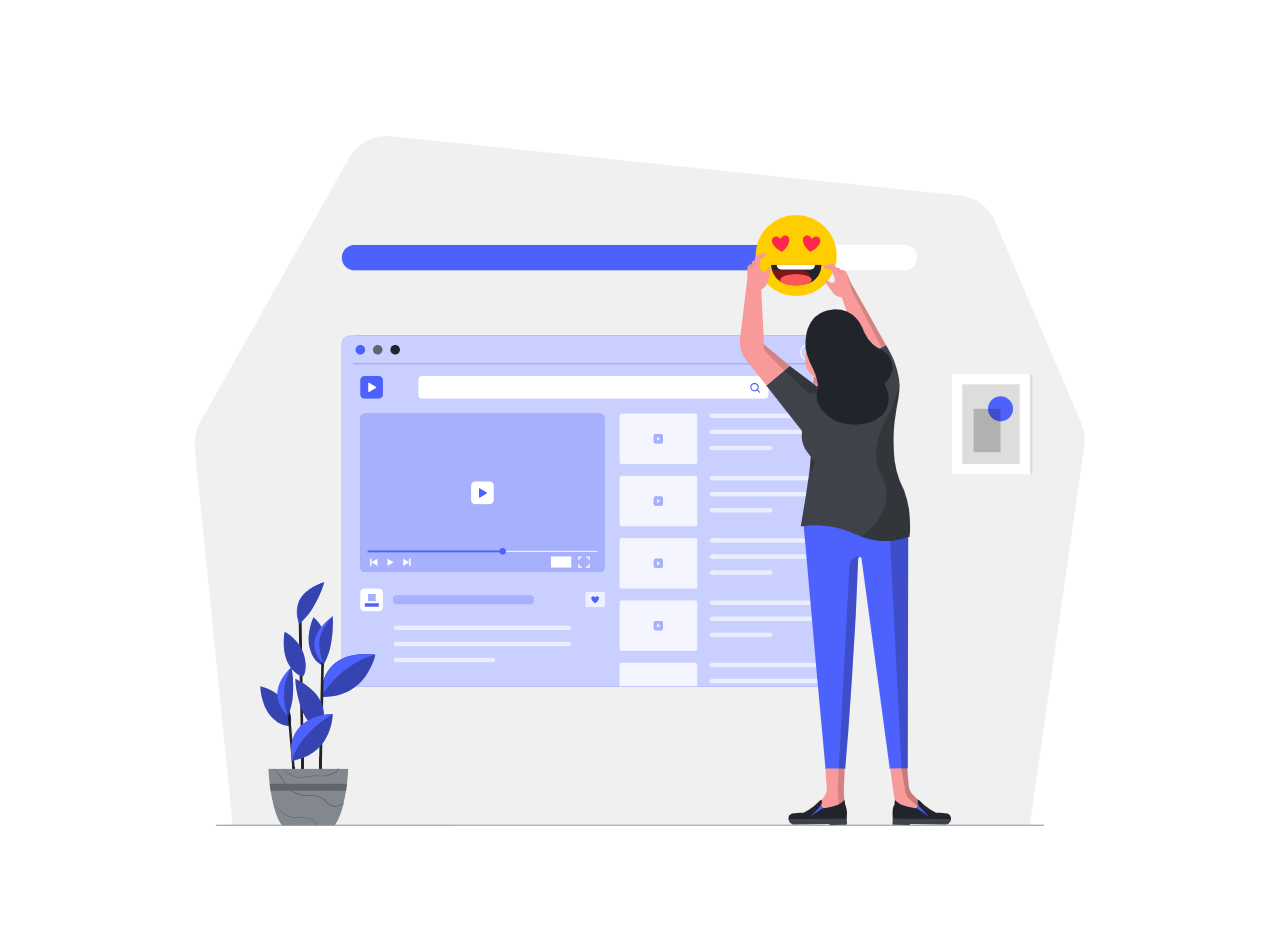
In the realm of React development, efficient data communication between parent and child components is fundamental for building robust and scalable applications. This guide explores various techniques and best practices for seamlessly passing data between React components, enabling developers to create modular and maintainable codebases.
-
Using Props for Parent-to-Child Communication
-
Introduction to Props: Props (short for properties) are a mechanism for passing data from a parent component to its child components. They provide a straightforward and fundamental way to establish communication within a React application.
// Parent Component const ParentComponent = () => { const dataToPass = "Hello from parent!"; return <ChildComponent message={dataToPass} />; }; // Child Component const ChildComponent = (props) => { return <p>{props.message}</p>; };
-
Passing Dynamic Data: Props can hold dynamic data, such as state variables, and are updated whenever the parent component re-renders. This ensures that the child components reflect the latest data from the parent.
-
-
Callback Functions for Child-to-Parent Communication
-
Callback Functions: To enable child components to communicate with their parent, callback functions can be passed as props. This allows child components to trigger functions in the parent component.
// Parent Component const ParentComponent = () => { const handleChildClick = (message) => { console.log(`Message from child: ${message}`); }; return <ChildComponent onClick={handleChildClick} />; }; // Child Component const ChildComponent = (props) => { const handleClick = () => { props.onClick("Hello from child!"); }; return <button onClick={handleClick}>Click Me</button>; };
-
Passing Data from Child to Parent: Through callback functions, child components can pass data to their parent components, enabling a bidirectional flow of information.
-
-
Using React Context for Global State
-
Introduction to Context API: The React Context API provides a way to share state data globally within a React application. While primarily used for managing global state, it can also be leveraged for passing data between deeply nested components.
// Create a context const MyContext = React.createContext(); // Parent Component const ParentComponent = () => { const dataToPass = "Hello from parent!"; return ( <MyContext.Provider value={dataToPass}> <ChildComponent /> </MyContext.Provider> ); }; // Child Component const ChildComponent = () => { const receivedData = useContext(MyContext); return <p>{receivedData}</p>; };
-
Global State Management: Context provides a central repository for state, making it accessible to any component that subscribes to it. This eliminates the need to pass data through multiple levels of nested components.
-
-
State Lifting for Complex State Management
-
State Lifting: In scenarios where multiple components need access to the same state, state lifting involves lifting the state up to a common ancestor component. This shared state is then passed down to child components as props.
// Common Ancestor Component const CommonAncestor = () => { const [sharedState, setSharedState] = useState("Shared state"); return ( <ParentComponent sharedState={sharedState} setSharedState={setSharedState} /> ); }; // Parent Component const ParentComponent = (props) => { return <ChildComponent sharedState={props.sharedState} />; }; // Child Component const ChildComponent = (props) => { return <p>{props.sharedState}</p>; };
-
Benefits of State Lifting: State lifting centralizes the management of shared state, simplifying the application’s structure and making it easier to reason about and maintain.
-
-
Redux for Global State Management
-
Introduction to Redux: Redux is a state management library commonly used with React to manage global state. It provides a centralized store where data can be stored and accessed by any component within the application.
// Redux Actions and Reducer const SET_DATA = 'SET_DATA'; const setData = (data) => { return { type: SET_DATA, payload: data }; }; const dataReducer = (state = null, action) => { switch (action.type) { case SET_DATA: return action.payload; default: return state; } }; // Redux Store const store = createStore(dataReducer); // Parent Component const ParentComponent = () => { const dataToPass = "Hello from parent!"; store.dispatch(setData(dataToPass)); return <ChildComponent />; }; // Child Component const ChildComponent = () => { const receivedData = useSelector(state => state); return <p>{receivedData}</p>; };
-
Managing Complex State: Redux is particularly useful when dealing with large-scale applications with complex state logic. It provides a predictable state container that facilitates the management of intricate application states.
-
-
Best Practices and Considerations
- Keep Components Stateless When Possible: Whenever feasible, aim to keep components stateless and rely on props for passing data. This promotes a cleaner architecture and facilitates better code maintenance.
- Consider the Scope of Data: Choose the data passing method based on the scope and relationship between components. For local communication, props might be sufficient, while global state management solutions like Redux or Context API are suitable for broader data sharing needs.
- Avoid Excessive Nesting: Minimize excessive nesting of components to reduce the complexity of data passing. If components are deeply nested, consider using state lifting or global state management for more straightforward communication.
- Opt for the Right Solution: The choice between props, context, state lifting, or state management libraries depends on the specific needs of your application. Evaluate the requirements and choose the most appropriate method for efficient data flow.
-
Conclusion
Efficient data flow is the backbone of React applications, and mastering the techniques for passing data between parent and child components is crucial for building scalable and maintainable projects. By understanding the various methods such as props, callback functions, context, state lifting, and state management libraries like Redux, developers can choose the right tool for the job and create applications that are not only functional but also well-structured and easy to maintain. As React continues to evolve, the principles of data flow remain foundational for successful component-based web development.
How to implement a parallax scrolling effect with Bootstrap
How to use Bootstrap’s list group as a menu
How to create a responsive pricing table with Bootstrap
How to implement a split-screen layout with Bootstrap
How to implement a card flip animation with Bootstrap
How to use Bootstrap’s table-responsive class
How to create a responsive login form with Bootstrap
How to use Bootstrap’s media objects