What is the difference between functional components and class components
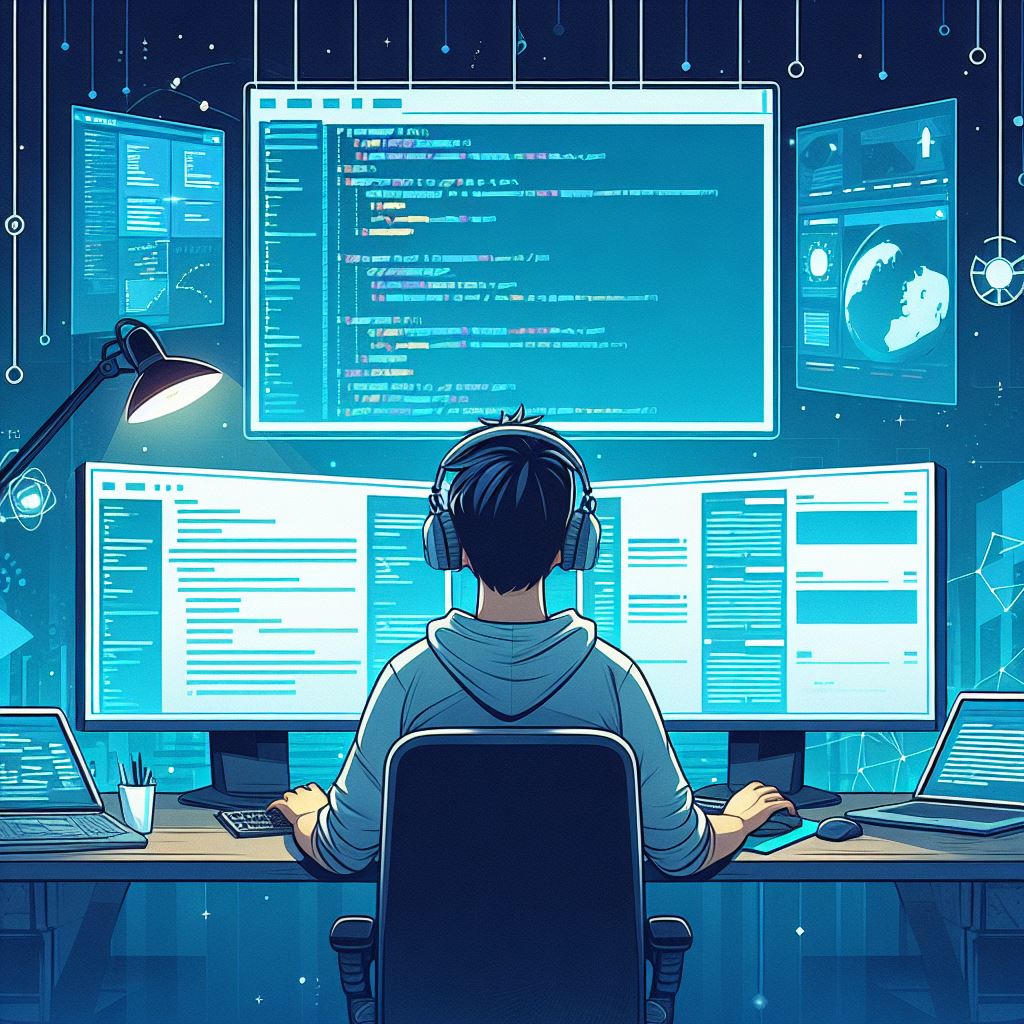
React, a popular JavaScript library for building user interfaces, offers two primary types of components: functional components and class components. While both serve the same fundamental purpose, they exhibit key differences in syntax, features, and use cases. In this comprehensive guide, we’ll explore the distinctions between functional and class components in React, providing insights into when to use each and how React has evolved over time.
-
Functional Components
-
Introduction to Functional Components: Functional components are a concise and modern way to define React components. They are written as JavaScript functions and primarily focus on rendering UI based on the props they receive.
// Example of a Functional Component const FunctionalComponent = (props) => { return <div>{props.message}</div>; };
-
Stateless Nature: Functional components are inherently stateless, meaning they don’t manage local state. They are ideal for simple, presentational components that receive data through props and render UI.
-
Introduction of Hooks: The introduction of Hooks in React 16.8 further enhanced the capabilities of functional components. Hooks, such as
useState
anduseEffect
, allow functional components to manage state and side effects effectively.
-
-
Class Components
-
Introduction to Class Components: Class components are the traditional way of defining React components. They are ES6 classes that extend from
React.Component
and can manage state, lifecycle methods, and more.// Example of a Class Component class ClassComponent extends React.Component { constructor(props) { super(props); this.state = { message: 'Hello from class component!' }; } render() { return <div>{this.state.message}</div>; } }
-
Local State Management: Class components can manage local state using the
setState
method. This allows them to handle dynamic data, respond to user interactions, and trigger re-renders based on state changes. -
Lifecycle Methods: Class components offer lifecycle methods like
componentDidMount
,componentDidUpdate
, andcomponentWillUnmount
. These methods provide control over the component’s lifecycle events.
-
-
Functional Components with Hooks
-
State Management with useState: Functional components can now manage state using the
useState
hook. This simplifies state management in functional components, making them more versatile and eliminating the need for class components in many cases.// Functional Component with Hooks import React, { useState } from 'react'; const FunctionalComponent = () => { const [message, setMessage] = useState('Hello from functional component!'); return <div>{message}</div>; };
-
Side Effects with useEffect: The
useEffect
hook allows functional components to handle side effects, such as data fetching, subscriptions, or manual DOM manipulations, in a clean and efficient way.
-
-
Performance Considerations
- Functional Components and Hooks Performance: With the introduction of Hooks, functional components have become more performant and offer a simpler syntax for managing state and side effects. Hooks allow for better code organization and reduce the cognitive load compared to class components.
- Class Components Performance: While class components are still widely used, especially in existing codebases, they may exhibit a slightly higher performance overhead due to the nature of class instantiation and the additional complexity of managing state and lifecycle methods.
-
Syntax and Readability
- Conciseness of Functional Components: Functional components, especially when using arrow function syntax and Hooks, are more concise and easier to read. They reduce boilerplate code and make the component’s purpose more apparent.
- Verbosity of Class Components: Class components are more verbose and involve additional syntax for defining constructors, binding methods, and managing the component’s lifecycle. This verbosity can lead to less readable code, especially for simple components.
-
Use Cases and Best Practices
- When to Use Functional Components: Functional components are the preferred choice for most scenarios. They are suitable for presentational components, components that don’t require state management, and cases where Hooks provide sufficient functionality.
- When to Use Class Components: Class components may still be necessary in certain situations, such as when working with older codebases, integrating with third-party libraries that rely on class components, or when dealing with complex state and lifecycle logic.
- Transitioning from Class to Functional Components: As functional components with Hooks offer a more modern and concise syntax, transitioning from class to functional components is often a good practice. This enhances code readability, maintainability, and takes advantage of the latest features in React.
-
Conclusion
React’s evolution has brought about significant changes in how components are defined and managed. While class components have been a staple in React development, the rise of functional components with Hooks has simplified and enhanced the way developers approach component composition. Understanding the differences between functional and class components empowers developers to make informed decisions based on their project’s requirements, code readability, and best practices in the ever-evolving landscape of React development.
How do you handle code splitting with React Router
How does React handle code splitting with React.lazy, Suspense, and webpack 5
How does React handle context switching
What is the purpose of middleware in Redux
How does React handle forms and form validation
What are the best practices for managing state in a React application