How can you implement authentication with third-party providers in Next.js
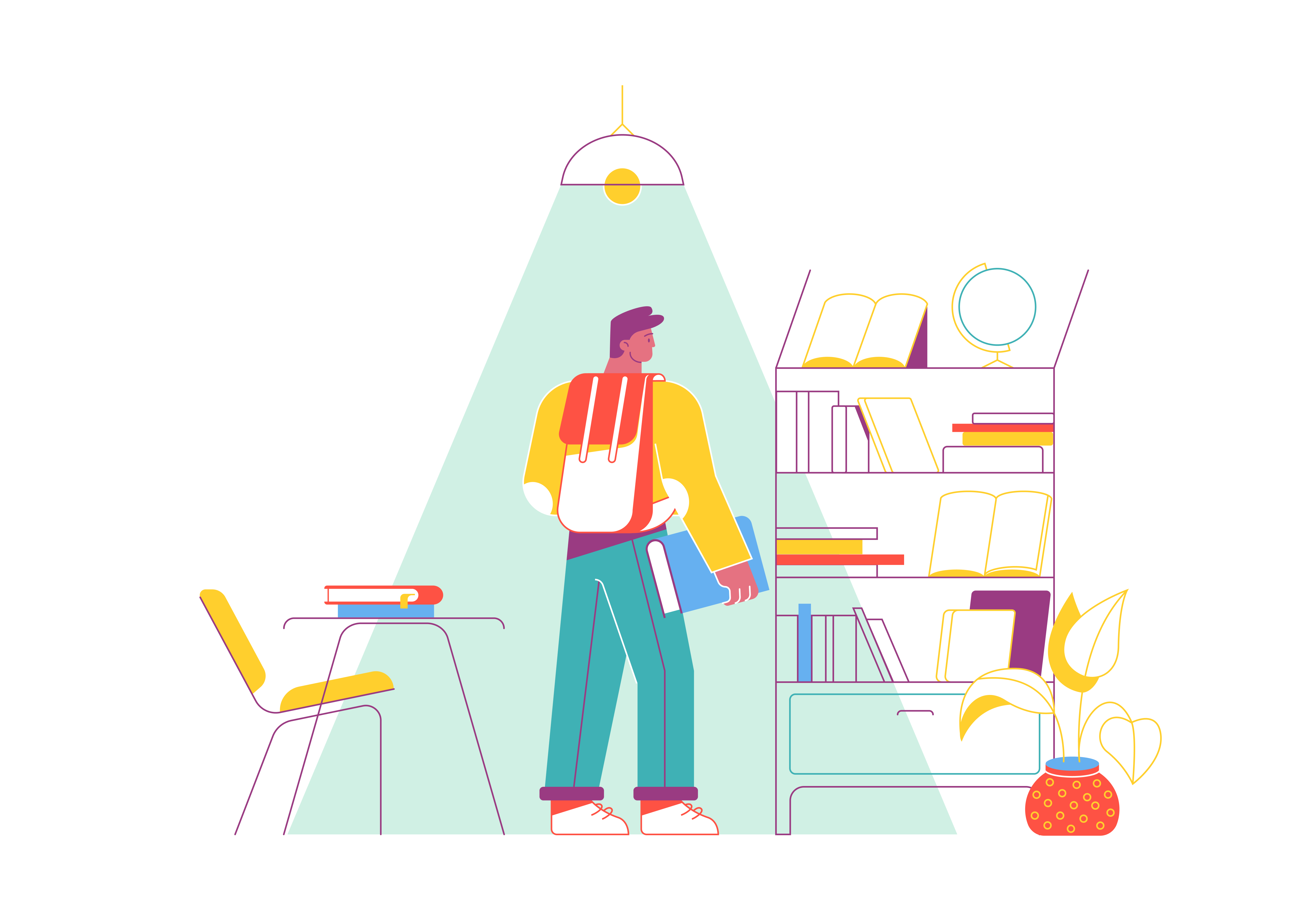
Authentication is a crucial aspect of modern web applications, ensuring that users can securely access and interact with protected resources. While building authentication systems from scratch can be complex and time-consuming, integrating third-party authentication providers offers a convenient and secure solution. In this blog post, we’ll explore how you can implement authentication with third-party providers in a Next.js application, leveraging popular services like Google, Facebook, and GitHub.
Why Use Third-Party Authentication Providers
Before diving into the implementation details, let’s understand the benefits of using third-party authentication providers:
- Simplified Authentication: Third-party providers handle the complexity of user authentication, including user registration, password management, and account recovery, freeing developers from implementing these features themselves.
- Improved Security: Authentication providers employ industry-standard security practices, such as OAuth 2.0 and OpenID Connect, to authenticate users securely and protect sensitive information.
- Social Login: Integrating third-party providers allows users to sign in using their existing accounts from popular platforms like Google, Facebook, and GitHub, eliminating the need to create new credentials.
Implementing Authentication with Third-Party Providers
Next.js provides a flexible and straightforward approach to integrating authentication with third-party providers. Let’s explore how you can implement authentication using OAuth 2.0, a widely adopted protocol for authentication and authorization.
1. Set Up OAuth 2.0 Credentials
Start by creating OAuth 2.0 credentials for the desired third-party providers (e.g., Google, Facebook, GitHub) in their respective developer consoles. Obtain the client ID and client secret, which you’ll need to authenticate users in your Next.js application.
2. Install OAuth Libraries
Next, install OAuth libraries for the desired providers using npm or yarn. For example, you can install next-auth
for Next.js applications:
npm install next-auth
3. Configure Authentication Providers
Configure the authentication providers in your Next.js application by specifying the client ID, client secret, and callback URL. This information varies depending on the provider but typically involves adding configuration options in your Next.js application’s configuration file.
// next.config.js
module.exports = {
env: {
GOOGLE_CLIENT_ID: 'YOUR_GOOGLE_CLIENT_ID',
GOOGLE_CLIENT_SECRET: 'YOUR_GOOGLE_CLIENT_SECRET',
FACEBOOK_CLIENT_ID: 'YOUR_FACEBOOK_CLIENT_ID',
FACEBOOK_CLIENT_SECRET: 'YOUR_FACEBOOK_CLIENT_SECRET',
// Add configuration for other providers
},
};
4. Implement Authentication Routes
Create authentication routes in your Next.js application to handle user authentication. Use the OAuth libraries to authenticate users with the configured providers and handle the authentication process.
// pages/api/auth/[...nextauth].js
import NextAuth from 'next-auth';
import Providers from 'next-auth/providers';
export default NextAuth({
providers: [
Providers.Google({
clientId: process.env.GOOGLE_CLIENT_ID,
clientSecret: process.env.GOOGLE_CLIENT_SECRET,
}),
Providers.Facebook({
clientId: process.env.FACEBOOK_CLIENT_ID,
clientSecret: process.env.FACEBOOK_CLIENT_SECRET,
}),
// Add providers for other third-party services
],
});
5. Integrate Authentication Components
Integrate authentication components into your Next.js application, such as login buttons for each provider and a logout button. Use the useSession
hook provided by next-auth
to manage user sessions and display the appropriate UI based on the user’s authentication status.
// components/AuthButtons.js
import { signIn, signOut, useSession } from 'next-auth/react';
const AuthButtons = () => {
const { data: session } = useSession();
return (
<div>
{!session ? (
<>
<button onClick={() => signIn('google')}>Sign in with Google</button>
<button onClick={() => signIn('facebook')}>Sign in with Facebook</button>
{/* Add buttons for other providers */}
</>
) : (
<button onClick={() => signOut()}>Sign out</button>
)}
</div>
);
};
export default AuthButtons;
Conclusion
Implementing authentication with third-party providers in a Next.js application offers numerous benefits, including simplified authentication, improved security, and support for social login. By following the steps outlined in this guide, you can seamlessly integrate authentication with popular providers like Google, Facebook, and GitHub into your Next.js application, providing users with a secure and convenient authentication experience. Elevate your Next.js application with third-party authentication and streamline the user login process today.
How do you pass data between parent and child components in React
What is the difference between functional components and class components
How do you handle authentication in a React-Redux application
How does React handle dynamic imports
How do you handle code splitting with React Router
Explain the concept of lazy loading in React
What is the significance of the forwardRef function in React
What is the significance of the react-router-dom library
How do you implement server-side rendering with React and Node.js
How do you implement server-side rendering with React and Express