How to implement a sticky header with Bootstrap
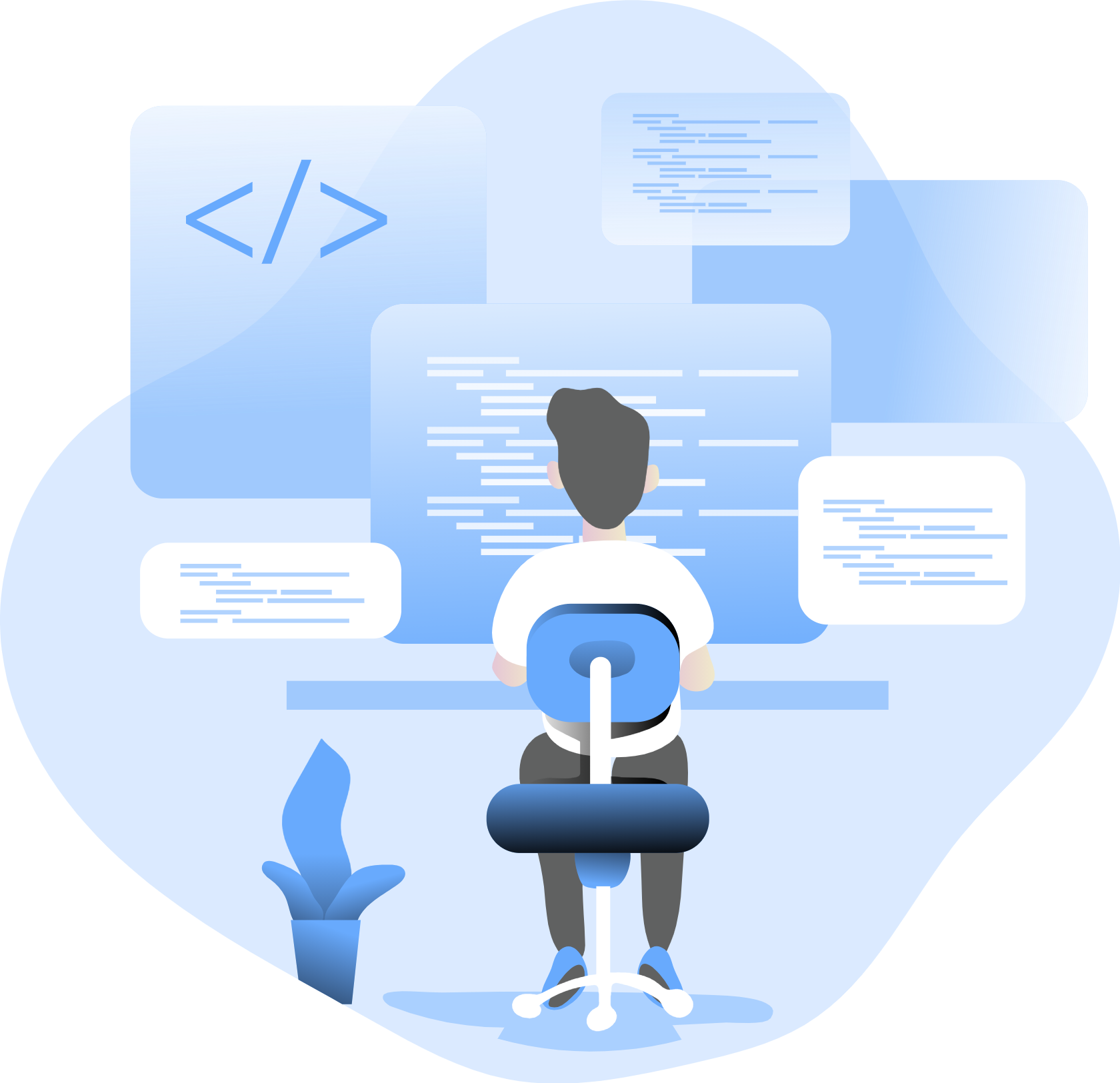
Sticky headers are a popular design element that enhances user navigation on websites, ensuring that the header remains visible as users scroll through the content. Leveraging the capabilities of Bootstrap, a leading front-end framework, we can seamlessly implement a sticky header to improve user experience and provide a polished design. In this comprehensive guide, we’ll explore the step-by-step process of implementing a sticky header with Bootstrap.
Understanding the Concept
A sticky header, also known as a fixed header, is a navigation bar that remains fixed at the top of the viewport as users scroll down a webpage. This feature ensures easy access to essential navigation links and enhances user engagement. Bootstrap simplifies the implementation of a sticky header by providing classes that facilitate the creation of a clean and responsive design.
Setting Up Your Project
Before diving into the implementation, make sure you have Bootstrap integrated into your project. You can either download the Bootstrap files and include them locally or use a Content Delivery Network (CDN). Here’s a basic HTML template with Bootstrap included:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link href="path/to/bootstrap.min.css" rel="stylesheet">
<title>Sticky Header with Bootstrap</title>
</head>
<body>
<!-- Your content goes here -->
<script src="path/to/bootstrap.bundle.min.js"></script>
</body>
</html>
Replace “path/to/bootstrap.min.css” and “path/to/bootstrap.bundle.min.js” with the correct file paths or URLs.
Creating the Sticky Header
To implement a sticky header with Bootstrap, you can use the fixed-top
class along with other utility classes. Here’s an example:
<nav class="navbar navbar-expand-lg navbar-light bg-light fixed-top">
<div class="container">
<a class="navbar-brand" href="#">Your Logo</a>
<button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNav">
<ul class="navbar-nav ml-auto">
<li class="nav-item active">
<a class="nav-link" href="#">Home</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">About</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Services</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Contact</a>
</li>
</ul>
</div>
</div>
</nav>
In this example, the fixed-top
class is added to the nav
element, making the header sticky. The container
class is used to ensure that the content within the header is appropriately aligned.
Customizing the Sticky Header
Bootstrap allows for extensive customization of the sticky header to match your design preferences. You can modify the background color, typography, and other styling elements using Bootstrap’s utility classes or by adding your custom CSS.
<style>
/* Custom styles for the sticky header */
.navbar {
background-color: #3498db; /* Change to your preferred color */
}
.navbar-brand {
color: #ffffff !important; /* Change to your preferred text color */
}
.navbar-nav .nav-link {
color: #ffffff !important; /* Change to your preferred text color */
}
</style>
In this CSS snippet, we’ve customized the background color of the navbar to a shade of blue and adjusted the text color for better visibility.
Handling Content Below the Sticky Header
When you have a sticky header, content below it may get partially hidden. To prevent this, you can add padding to the top of the body
element to accommodate the fixed header’s height.
<style>
/* Add padding to the top of the body to prevent content from being hidden */
body {
padding-top: 56px; /* Adjust according to your header's height */
}
</style>
Adjust the padding-top
value based on your specific header height to ensure that the content starts below the sticky header.
Responsive Design Considerations
Bootstrap’s responsive design principles seamlessly apply to sticky headers. The fixed-top
class ensures that the header adapts to different screen sizes, providing an optimal viewing experience on devices ranging from large desktop monitors to small mobile screens.
To further enhance responsiveness, include the Bootstrap viewport meta tag in the <head>
section of your HTML:
<meta name="viewport" content="width=device-width, initial-scale=1.0">
This meta tag ensures proper scaling on various devices.
Conclusion
Implementing a sticky header with Bootstrap elevates the visual appeal and functionality of your website. By following this step-by-step guide, you can create a polished and responsive design that enhances user navigation and engagement. Customize the styling to align with your brand aesthetics and experiment with additional Bootstrap components to create a cohesive and user-friendly web experience. With the power of Bootstrap’s utility classes, crafting a sticky header becomes a seamless process, allowing you to focus on delivering a compelling and dynamic user interface.
How to use Bootstrap’s text truncation classes for long content
How to create a responsive contact form with validation in Bootstrap
How to use Bootstrap’s container and container-fluid classes
How to implement a full-screen background image with Bootstrap
How to use Bootstrap’s list group with badges
How to use Bootstrap’s custom form controls for file inputs
How to implement a fixed sidebar with a scrollable content area