How to resolve CORS errors in a web application
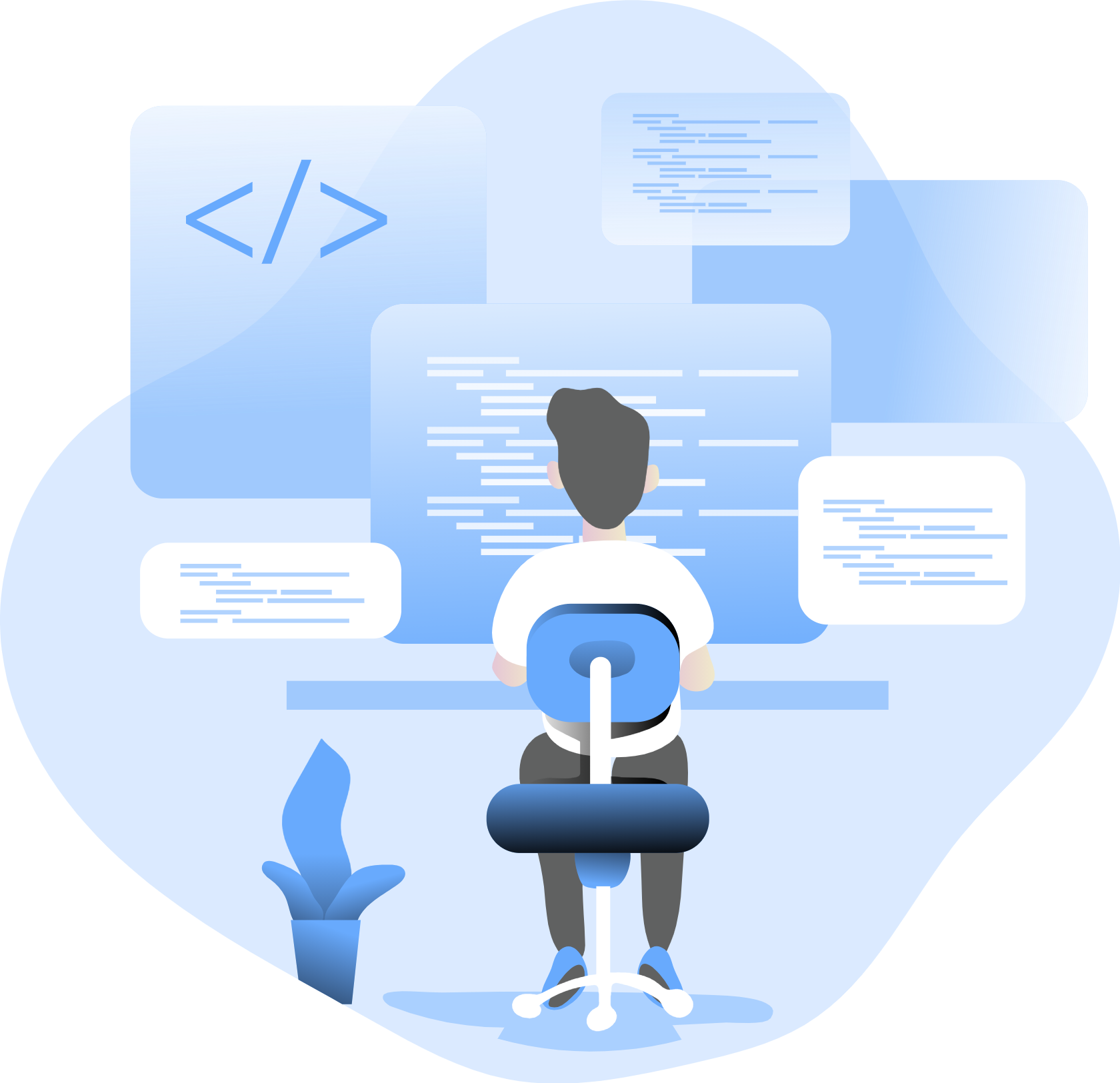
Cross-Origin Resource Sharing (CORS) is an essential aspect of web security and a common issue developers encounter when building web applications. CORS errors can seem complex if you’re new to the topic, but with a proper understanding, they can be resolved efficiently. This article will walk you through the fundamental concepts of CORS, explain why these errors occur, and provide detailed methods to resolve them.
1. Introduction to CORS
What is CORS?
CORS stands for Cross-Origin Resource Sharing, a security feature implemented by browsers to control how web pages can request resources from a different domain than the one they originated from.
For example, suppose your application is hosted at https://example.com
and it tries to make a request to an API at https://api.otherdomain.com
. Due to security reasons, the browser will block the request unless the server at https://api.otherdomain.com
explicitly allows it.
Why does CORS exist?
CORS exists to protect users from malicious websites that might try to request data from other sites without the user’s consent. It’s a safeguard to prevent what’s known as Cross-Site Request Forgery (CSRF) attacks.
Without CORS, a website could make requests to other domains, potentially accessing sensitive data like user information or login sessions, without the user knowing.
2. Understanding How CORS Works
To understand how to resolve CORS errors, it’s essential to understand the request and response cycle in CORS.
Same-Origin Policy
By default, web browsers follow the Same-Origin Policy (SOP), which restricts web pages from making requests to a different origin than their own. An origin consists of:
- Scheme/Protocol: (e.g.,
http
orhttps
) - Domain: (e.g.,
example.com
) - Port: (e.g.,
8080
)
If any of these components differ, the request is considered a cross-origin request, triggering the CORS mechanism.
Simple Requests vs Preflighted Requests
There are two types of CORS requests:
1. Simple Requests:
A simple request is one that meets specific criteria, such as using one of the following HTTP methods:
GET
POST
HEAD
Additionally, the request headers must be limited to specific values like:
Content-Type: application/x-www-form-urlencoded
Content-Type: multipart/form-data
Content-Type: text/plain
If the request is simple, the browser sends it directly to the server.
2. Preflighted Requests:
If a request uses HTTP methods like PUT
, DELETE
, or includes custom headers, the browser sends a preflight request using the OPTIONS
method to check if the actual request is allowed. If the server responds with the appropriate headers, the browser proceeds with the actual request.
The CORS Headers
To allow a cross-origin request, the server needs to include specific CORS headers in its response:
- Access-Control-Allow-Origin: Specifies which domains are allowed to access the resource. It can be a specific domain (e.g.,
https://example.com
) or a wildcard (*
) to allow any domain. - Access-Control-Allow-Methods: Lists the HTTP methods allowed (e.g.,
GET
,POST
,PUT
). - Access-Control-Allow-Headers: Specifies which request headers can be sent by the client (e.g.,
Authorization
,Content-Type
). - Access-Control-Allow-Credentials: Allows cookies or other credentials to be sent in the request.
- Access-Control-Max-Age: Caches the preflight request result for a specified time (in seconds).
3. What is a CORS Error?
A CORS error occurs when the browser detects that the request violates the security policy of the target server.
Example of a CORS Error
When a CORS error happens, the browser typically blocks the request and outputs an error in the developer console. The error message may look like this:
Access to XMLHttpRequest at 'https://api.otherdomain.com' from origin 'https://example.com' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource.
This means that the server at https://api.otherdomain.com
has not allowed cross-origin requests from https://example.com
.
Why Do CORS Errors Occur?
CORS errors occur when:
- The server doesn’t explicitly allow the requesting origin.
- The request includes methods or headers that are not allowed.
- The preflight request fails because the server doesn’t respond with the correct CORS headers.
4. How to Resolve CORS Errors
Solution 1: Enable CORS on the Server
The most effective way to resolve CORS issues is by configuring the server to handle cross-origin requests properly.
How to Do It:
-
Node.js (Express): In a Node.js/Express application, you can use the
cors
middleware to easily configure CORS:const express = require('express'); const cors = require('cors'); const app = express(); // Allow all domains to access the server app.use(cors()); // Or allow only specific domains app.use(cors({ origin: 'https://example.com' })); app.listen(3000, () => { console.log('Server running on port 3000'); });
-
Django: In a Django application, install the
django-cors-headers
package:pip install django-cors-headers
Then, add it to your
INSTALLED_APPS
and configure allowed origins:INSTALLED_APPS = [ ... 'corsheaders', ] MIDDLEWARE = [ ... 'corsheaders.middleware.CorsMiddleware', 'django.middleware.common.CommonMiddleware', ] CORS_ALLOWED_ORIGINS = [ 'https://example.com', ]
-
ASP.NET: In an ASP.NET application, you can enable CORS by adding the
Microsoft.AspNetCore.Cors
package and configuring it inStartup.cs
:public void ConfigureServices(IServiceCollection services) { services.AddCors(options => { options.AddPolicy("AllowSpecificOrigin", builder => builder.WithOrigins("https://example.com") .AllowAnyHeader() .AllowAnyMethod()); }); } public void Configure(IApplicationBuilder app, IHostingEnvironment env) { app.UseCors("AllowSpecificOrigin"); }
Solution 2: Use a Proxy Server
Sometimes, you don’t have control over the server, and you need to work around the CORS issue. In this case, a proxy server can be used.
How to Do It:
-
Set up a Proxy: You can set up a proxy server that your front-end application communicates with. The proxy server forwards the requests to the actual target server, bypassing the CORS restrictions.
-
Configure Webpack Dev Server (For React or Vue): If you’re using a front-end framework with Webpack, you can configure the Webpack dev server to proxy requests to your API:
module.exports = { devServer: { proxy: { '/api': { target: 'https://api.otherdomain.com', changeOrigin: true, }, }, }, };
Solution 3: Use JSONP (Only for GET Requests)
JSONP (JSON with Padding) is an old technique that can be used to bypass CORS, but it’s only suitable for GET
requests. With JSONP, you inject a <script>
tag into your HTML to request data from another domain.
However, JSONP has several limitations and security risks, so it’s not recommended for modern applications.
Solution 4: Modify the Client-Side Code (Temporary Fix)
You can temporarily resolve CORS issues by modifying your client-side code to handle errors gracefully. However, this is not a permanent solution and should only be used for testing.
For example, in a JavaScript application, you can handle the error using try...catch
:
try {
const response = await fetch('https://api.otherdomain.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('CORS error:', error);
}
5. Best Practices for Handling CORS
1. Restrict Allowed Origins
Instead of using a wildcard (*
) to allow all domains, specify trusted domains in your CORS configuration. This prevents unauthorized domains from accessing your resources.
app.use(cors({
origin: ['https://example.com', 'https://anotherdomain.com']
}));
2. Limit Allowed Methods and Headers
Only allow the necessary HTTP methods and headers to minimize the risk of malicious requests:
app.use(cors({
origin: 'https://example.com',
methods: ['GET', 'POST'],
allowedHeaders: ['Content-Type', 'Authorization']
}));
3. Use Credentials Securely
If your application requires sending credentials (cookies or HTTP authentication), ensure the server is properly configured to handle it:
app.use(cors({
origin: 'https://example.com',
credentials: true
}));
And in the client code:
fetch('https://api.example.com/data', {
credentials: 'include'
});
4. Cache Preflight Requests
To reduce the number of preflight requests, you can cache the results by using the Access-Control-Max-Age
header. This can improve performance by reusing the preflight check for future requests within the specified time:
Access-Control-Max-Age: 3600
6. Conclusion
CORS errors are a common hurdle in web development, but they exist for good reasons related to security. Understanding how CORS works and configuring your server to allow cross-origin requests is key to resolving these errors. Whether you have control over the server or need to implement a proxy solution, there are several ways to tackle CORS issues and ensure smooth communication between different origins.
How to implement a card flip animation with Bootstrap
How to use Bootstrap’s table-responsive class
How to create a responsive login form with Bootstrap
How to use Bootstrap’s media objects
How to integrate Bootstrap with JavaScript libraries like jQuery
How to implement a modal/popup with Bootstrap