What is the significance of the react-router-dom library
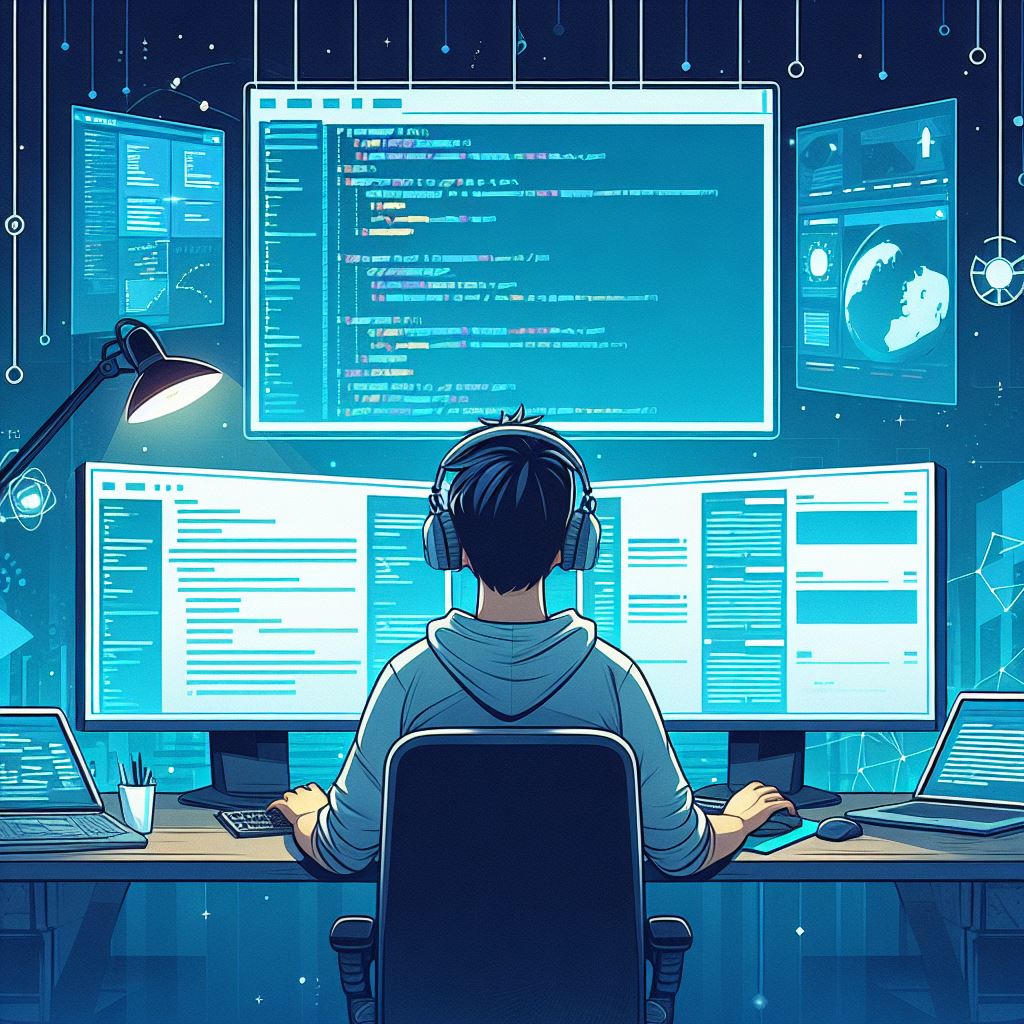
The advent of single-page applications (SPAs) has revolutionized web development, and at the forefront of seamless navigation in React applications stands the react-router-dom
library. This comprehensive guide aims to uncover the significance of react-router-dom
, exploring its core features, benefits, and practical applications that empower developers to create dynamic and engaging user interfaces.
Introduction to react-router-dom
- Navigating in React SPAs: Establish the need for a robust navigation solution in React applications, highlighting the challenges of managing complex navigation structures in SPAs.
- What is react-router-dom?:
Introduce
react-router-dom
as the go-to library for handling routing and navigation in React applications. Outline its role in creating a declarative and efficient way to manage UI state and URLs.
Core Features of react-router-dom
-
Declarative Routing with Route Component: Dive into the heart of
react-router-dom
with theRoute
component, showcasing how it enables developers to declaratively define UI components based on the URL.// Example of Declarative Routing with Route Component <Route path="/about" component={About} />
-
Nested Routes and Route Matching: Explore the versatility of
react-router-dom
in handling nested routes, allowing developers to structure their applications logically and manage complex UI hierarchies. -
Navigation with Link Component: Introduce the
Link
component, a fundamental tool for creating hyperlinks that seamlessly navigate between different routes while preserving the SPA experience.// Example of Navigation with Link Component <Link to="/about">Go to About Page</Link>
-
Dynamic Route Parameters: Showcase the power of dynamic route parameters in
react-router-dom
, enabling developers to create flexible routes that adapt to dynamic data.// Example of Dynamic Route Parameters <Route path="/user/:id" component={UserProfile} />
Benefits of Using react-router-dom
- Maintaining UI State:
Highlight how
react-router-dom
seamlessly manages UI state by synchronizing it with the URL, enabling the creation of bookmarkable and shareable URLs. - Enhancing User Experience: Discuss how the library contributes to a smoother user experience by preventing full page reloads during navigation, leading to faster transitions between views.
- Code Organization and Separation of Concerns:
Emphasize how
react-router-dom
encourages a clean separation of concerns by centralizing routing logic, resulting in well-organized and maintainable codebases.
Practical Applications and Use Cases
-
Authentication and Route Guarding: Explore how
react-router-dom
facilitates authentication and route guarding, allowing developers to control access to specific routes based on the user’s authentication status. -
Conditional Rendering with Switch: Showcase the usage of
Switch
to conditionally render the firstRoute
that matches, providing a powerful tool for creating exclusive routes.// Example of Conditional Rendering with Switch <Switch> <Route path="/home" component={Home} /> <Route path="/about" component={About} /> </Switch>
-
Animating Route Transitions: Demonstrate how developers can leverage
react-router-dom
in conjunction with CSS transitions or third-party animation libraries to create smooth route transition animations.
Integration with React Context and Hooks
- Combining with React Context:
Explore how
react-router-dom
seamlessly integrates with React Context, allowing developers to share routing information across components without the need for prop drilling. - Hooks for Navigation:
Introduce navigation hooks like
useHistory
anduseParams
, empowering developers to access navigation history and retrieve dynamic route parameters programmatically.
Server-Side Rendering (SSR) and react-router-dom
- Enabling Server-Side Rendering:
Discuss how
react-router-dom
supports Server-Side Rendering (SSR), enabling developers to implement efficient server rendering for improved SEO and initial page load performance. - Hydrating on the Client Side: Explain the process of hydrating client-side navigation after server-side rendering, ensuring a seamless transition between server-rendered and client-rendered content.
Optimizing Performance with react-router-dom
-
Lazy Loading and Code Splitting: Showcase how
react-router-dom
seamlessly integrates with code splitting, allowing developers to lazy load components and optimize performance by loading only the necessary code for a specific route.// Example of Lazy Loading with react-router-dom <Route path="/lazy" component={lazy(() => import('./LazyLoadedComponent'))} />
-
Preloading Routes: Discuss techniques for preloading routes with
react-router-dom
, ensuring that critical resources for upcoming routes are loaded in the background to enhance user experience.// Example of Preloading Routes with react-router-dom <Link to="/preloaded" onMouseOver={() => preload('/preloaded')}> Preload this route </Link>
Testing and Debugging react-router-dom Applications
-
Unit Testing Routes: Provide insights into unit testing routes and navigation logic using testing libraries like Jest and React Testing Library.
// Jest Test for react-router-dom test('renders Home component for / route', () => { render( <MemoryRouter initialEntries={['/']}> <App /> </MemoryRouter> ); expect(screen.getByText(/Home/i)).toBeInTheDocument(); });
-
Debugging Navigation Issues: Guide developers on debugging common navigation issues using browser developer tools and React DevTools, ensuring a smooth and error-free navigation experience.
SEO Considerations and react-router-dom
- Creating SEO-Friendly Routes:
Address the importance of creating SEO-friendly routes with
react-router-dom
, emphasizing the role of meaningful URLs and metadata for search engine optimization. - Server-Side Rendering for SEO:
Revisit the significance of server-side rendering with
react-router-dom
in the context of SEO, ensuring that search engines can efficiently crawl and index content.
Updates and Versioning in react-router-dom
- Handling Updates and Breaking Changes:
Provide guidance on handling updates and potential breaking changes in
react-router-dom
, emphasizing the importance of staying informed about library updates and versioning.
Community and Ecosystem
- Rich Ecosystem and Plugins:
Explore the rich ecosystem surrounding
react-router-dom
, including various plugins and extensions that enhance its functionality for specific use cases. - Community Support and Documentation:
Highlight the strong community support and well-documented nature of
react-router-dom
, making it an accessible and well-maintained library for React developers.
Conclusion
react-router-dom
emerges as an indispensable tool for React developers, providing a comprehensive and flexible solution for handling navigation and routing in single-page applications. By understanding its core features, practical applications, and optimization strategies, developers can harness the full potential of react-router-dom
to create dynamic, engaging, and performant user interfaces. Dive into the world of seamless navigation with react-router-dom
and elevate your React applications to new heights of user experience and code maintainability.
How many states are there in React
Where is state stored in React
How does React differ from other JavaScript frameworks
What is JSX, and how does it differ from regular JavaScript syntax
Uncover React’s Virtual DOM: Exploring Its Crucial Role and Advantage
How does React handle animations