What is the significance of the forwardRef function in React
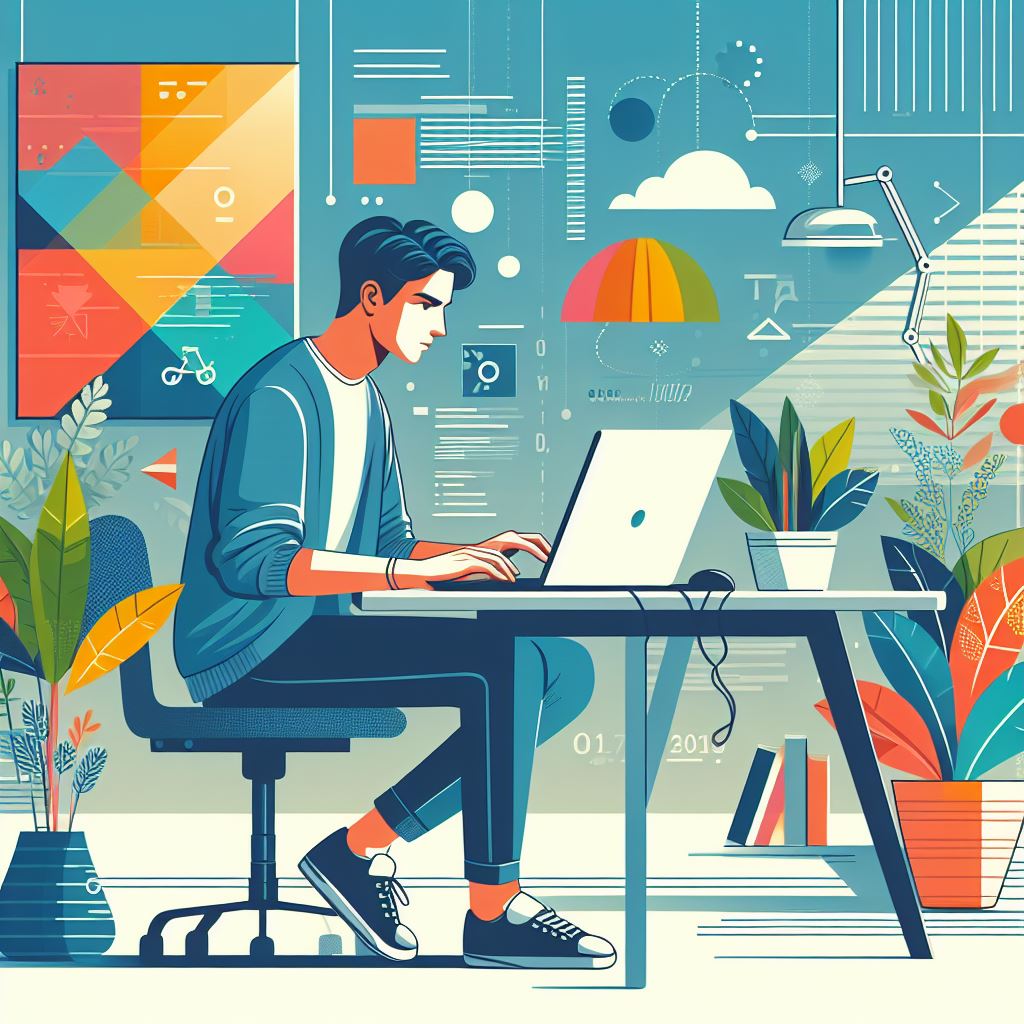
In the dynamic world of React, the forwardRef function stands out as a powerful tool, offering developers enhanced control and flexibility when dealing with component references. This comprehensive guide aims to unravel the significance of forwardRef in React, delving into its use cases, benefits, and practical examples to empower developers in harnessing its potential for building more modular and maintainable applications.
Understanding Refs in React
- Overview of Refs: Begin by establishing a foundational understanding of refs in React. Refs provide a way to access and interact with the DOM or React elements directly. They play a crucial role in scenarios where direct manipulation or inspection of a component is necessary.
- Use Cases for Refs: Explore common use cases for refs, including managing focus, triggering imperative animations, integrating with third-party libraries, and accessing underlying DOM elements.
Introduction to forwardRef
- What is forwardRef?: Introduce forwardRef as a higher-order function in React, designed to pass refs through a component to its child. Unlike traditional refs, forwardRef enables the encapsulation of ref logic within a component, making it more reusable and versatile.
- Benefits of Using forwardRef: Highlight the advantages of utilizing forwardRef, such as improved component abstraction, enhanced reusability, and the ability to separate concerns by keeping ref-related logic within the concerned component.
Basic Syntax and Usage
-
Syntax of forwardRef: Break down the syntax of forwardRef, showcasing how it’s applied to functional components and demonstrating its simplicity in usage.
const MyComponent = React.forwardRef((props, ref) => { // Component logic return <div ref={ref}>{/* Component JSX */}</div>; });
-
Creating Ref-Forwarding Components: Walk through the process of creating components that leverage forwardRef, emphasizing the seamless passing of refs from parent to child components.
Use Cases and Practical Examples
- Ref Forwarding for DOM Elements: Illustrate how forwardRef can be employed for forwarding refs to underlying DOM elements, facilitating direct manipulation or inspection of those elements.
- Creating Reusable Higher-Order Components: Showcase the creation of reusable higher-order components that utilize forwardRef, enabling developers to encapsulate complex ref-related logic within these components.
- Integrating with Third-Party Libraries: Explore scenarios where forwardRef is beneficial for integrating React components with third-party libraries, enhancing interoperability and ensuring a seamless developer experience.
Forwarding Refs in Higher-Order Components (HOCs)
- Overview of HOCs: Provide a brief overview of Higher-Order Components and their role in enhancing component composition and reusability.
- Using forwardRef with HOCs: Demonstrate how forwardRef can be seamlessly integrated into HOCs, allowing for the creation of higher-order components that efficiently forward refs to their wrapped components.
Optimizing Performance with forwardRef
- Avoiding Unnecessary Re-renders: Discuss how forwardRef can aid in avoiding unnecessary re-renders by allowing components to selectively re-render based on the changes in props or state, while ignoring changes in refs.
- Memoization and Pure Components: Explore memoization techniques and the use of React’s PureComponent to further optimize components that leverage forwardRef.
Best Practices and Considerations
- Documenting Ref-Forwarding Components: Emphasize the importance of documenting ref-forwarding components to improve code maintainability and provide clear guidelines for component usage.
- Avoiding Overuse of Refs: Discuss best practices for using refs in React, highlighting the importance of avoiding overuse and opting for declarative solutions when possible.
Forwarding Refs in Class Components
-
Compatibility with Class Components: Discuss the compatibility of forwardRef with class components, showcasing how the same ref-forwarding benefits can be achieved in both functional and class-based components.
class MyComponent extends React.Component { // Ref can be forwarded in class components as well }
Testing Ref-Forwarding Components
-
Unit Testing Strategies: Provide guidance on unit testing ref-forwarding components, utilizing testing libraries such as Jest and React Testing Library to ensure proper functionality and behavior.
// Jest Test for Ref-Forwarding Component test('renders MyComponent with forwarded ref', () => { const ref = React.createRef(); render(<MyComponent ref={ref} />); expect(ref.current).toBeInTheDocument(); });
Common Pitfalls and Troubleshooting
- Ref Forwarding Limitations: Address common pitfalls and limitations associated with ref forwarding, offering troubleshooting tips and solutions to overcome potential challenges.
Implementing forwardRef with Context
- Combining forwardRef and Context API: Showcase advanced use cases where forwardRef can be combined with the Context API, providing a powerful combination for managing state and refs in a React application.
Conclusion
In conclusion, forwardRef emerges as a valuable tool in the React developer’s arsenal, providing a clean and efficient way to handle references in components. By understanding its significance, mastering its usage, and incorporating it into your React projects, you can elevate your component design, improve code maintainability, and foster a more modular and scalable codebase. Explore the possibilities of forwardRef to unlock a new level of control and flexibility in your React applications.
How to create a responsive form layout with Bootstrap
How to implement a parallax scrolling effect with Bootstrap
How to use Bootstrap’s list group as a menu
How to create a responsive pricing table with Bootstrap
How to implement a split-screen layout with Bootstrap
How to implement a card flip animation with Bootstrap
How to use Bootstrap’s table-responsive class
How do you handle authentication in a React-Redux application
How does React handle dynamic imports
How do you implement server-side rendering with React and Node.js