Explain the concept of lazy loading in React
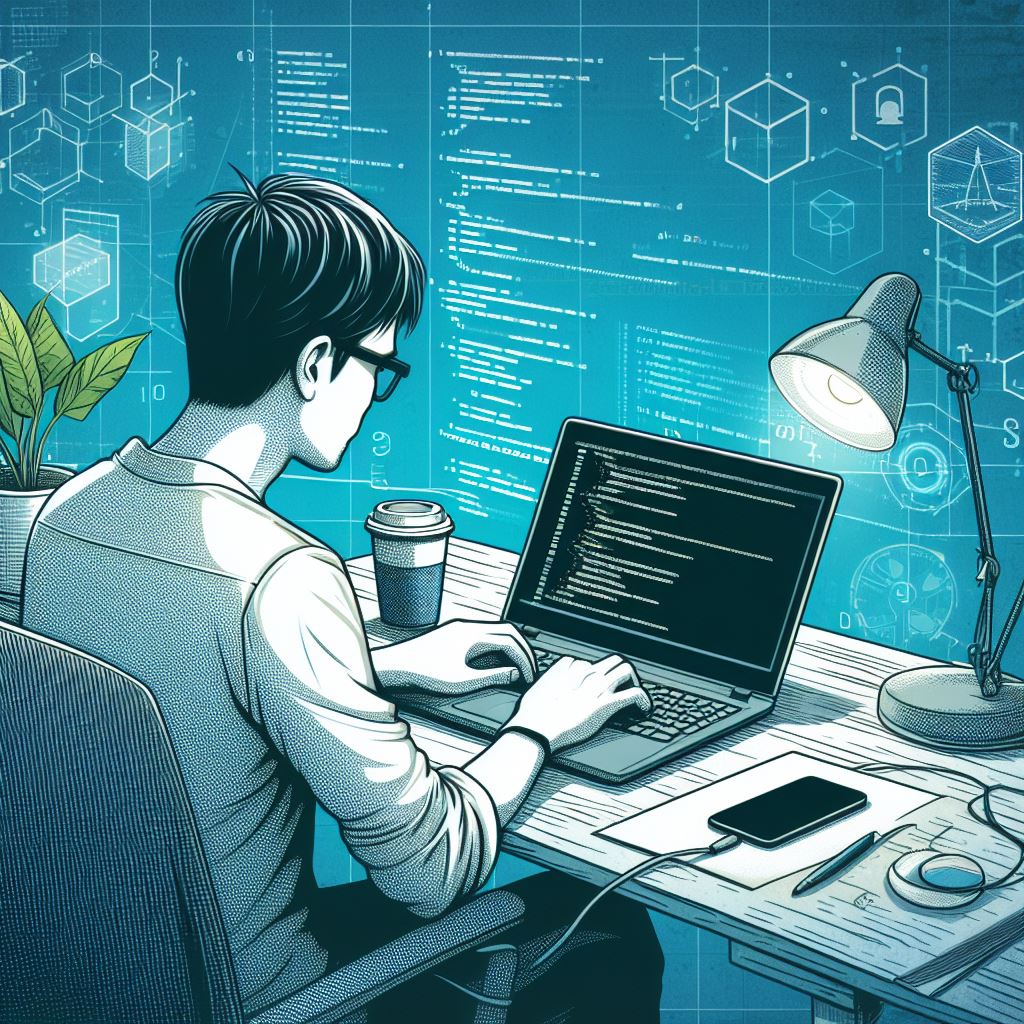
Lazy loading is a powerful optimization technique in React that allows you to defer the loading of certain components until they are actually needed. This not only helps reduce the initial load time of your application but also contributes to a more efficient use of resources. In this comprehensive guide, we’ll delve into the concept of lazy loading in React, exploring its benefits, implementation methods, and best practices for enhancing the performance of your React applications.
Understanding Lazy Loading
- Overview of Lazy Loading: Explore the fundamental concept of lazy loading, which involves delaying the loading of specific components or assets until they are required. This is particularly beneficial in scenarios where loading all resources upfront might lead to unnecessary delays and increased initial page load times.
- Benefits of Lazy Loading: Understand the advantages of lazy loading, including improved page load performance, reduced resource utilization, and a more responsive user experience. Lazy loading is especially valuable in large-scale applications with complex component hierarchies.
Lazy Loading in React
-
Dynamic Imports with
import()
: Learn how dynamic imports using theimport()
function enable lazy loading in React. This syntax allows you to load modules, components, or other assets asynchronously, ensuring they are fetched only when needed.// Dynamic Import Syntax for Lazy Loading const MyComponent = React.lazy(() => import('./MyComponent'));
-
React.lazy and Suspense: Explore the integration of
React.lazy
with theSuspense
component to handle the asynchronous loading of components. This combination simplifies the implementation of lazy loading and provides a mechanism for rendering fallback content while waiting for the dynamic import to resolve.// Using React.lazy with Suspense const MyComponentWrapper = () => ( <React.Suspense fallback={<LoadingSpinner />}> <MyComponent /> </React.Suspense> );
Implementation Strategies
-
Route-Based Lazy Loading: Implement lazy loading on a route-based level, ensuring that components are loaded only when the corresponding route is accessed. This approach minimizes the initial bundle size, optimizing the loading of resources.
// Route-Based Lazy Loading const Home = React.lazy(() => import('./Home')); const About = React.lazy(() => import('./About')); <BrowserRouter> <Switch> <Route path="/" exact component={Home} /> <Route path="/about" component={About} /> </Switch> </BrowserRouter>
-
Component-Level Lazy Loading: Explore component-level lazy loading for more granular control over when specific components are loaded. This strategy is useful when certain components are conditionally rendered or accessed based on user interactions.
// Component-Level Lazy Loading const MyComponentWrapper = React.lazy(() => import('./MyComponent')); const App = () => ( <div> <OtherComponent /> <MyComponentWrapper /> </div> );
Optimizing Lazy Loading Performance
- Bundle Analysis and Optimization: Utilize tools like Webpack Bundle Analyzer to analyze your application’s bundle size and composition. Optimize lazy loading by identifying opportunities to further split code and reduce unnecessary dependencies.
- Code-Splitting Libraries: Explore third-party libraries and tools, such as React Loadable or React Query, that provide additional features and customization options for lazy loading in React. These libraries can enhance the performance and maintainability of lazy-loaded components.
Error Handling and Fallback Mechanisms
-
Error Boundaries for Lazy-Loaded Components: Implement error boundaries around lazy-loaded components to gracefully handle any errors that may occur during the loading process. This prevents the entire application from breaking due to a failure in loading a specific component.
// Error Boundary for Lazy-Loaded Component class ErrorBoundary extends React.Component { componentDidCatch(error, errorInfo) { logErrorToService(error, errorInfo); } render() { return this.props.children; } }
-
Fallback UI Components: Create fallback UI components to display while lazy-loaded components are still in the process of loading. This ensures a smooth user experience by providing visual feedback during asynchronous loading.
// Fallback UI Component for Lazy Loading const LoadingFallback = () => <div>Loading...</div>; const MyComponentWrapper = React.lazy(() => import('./MyComponent')); const App = () => ( <React.Suspense fallback={<LoadingFallback />}> <MyComponentWrapper /> </React.Suspense> );
Lazy Loading Images and Other Assets
-
Lazy Loading Images: Extend the concept of lazy loading to images and other assets within your React application. Utilize the
loading="lazy"
attribute for images to defer their loading until they are within the user’s viewport.// Lazy Loading Images in React <img src="image.jpg" alt="Lazy Loaded Image" loading="lazy" />
-
Intersection Observer API: Implement the Intersection Observer API to track when elements come into the user’s viewport. This enables a more fine-grained approach to lazy loading, ensuring that assets are loaded precisely when they are needed.
// Intersection Observer for Lazy Loading const observer = new IntersectionObserver((entries) => { entries.forEach((entry) => { if (entry.isIntersecting) { // Load the asset entry.target.src = entry.target.dataset.src; observer.unobserve(entry.target); } }); }); const LazyImage = ({ src, alt }) => { const ref = useRef(); useEffect(() => { observer.observe(ref.current); return () => observer.disconnect(); }, []); return <img ref={ref} data-src={src} alt={alt} />; };
Testing Lazy Loaded Components
-
Unit Testing Dynamically Imported Components: Learn effective strategies for unit testing components that are dynamically imported. Utilize testing libraries such as Jest and React Testing Library to ensure the proper rendering and behavior of lazily loaded components.
// Jest Test for Dynamically Imported Component test('renders MyComponent', async () => { const { findByText } = render( <React.Suspense fallback="Loading..."> <MyComponent /> </React.Suspense> ); expect(await findByText('Hello World!')).toBeInTheDocument(); });
-
Integration Testing with Jest and Puppeteer: Conduct integration tests using tools like Jest and Puppeteer to simulate user interactions and verify that lazy-loaded components work seamlessly in real-world scenarios.
// Jest and Puppeteer Integration Test test('renders lazy-loaded component on user interaction', async () => { const browser = await puppeteer.launch(); const page = await browser.newPage(); await page.goto('http://localhost:3000/'); // Simulate user interaction triggering lazy loading await page.click('button'); const lazyLoadedComponent = await page
.waitForSelector('.lazy-loaded-component'); expect(lazyLoadedComponent).toBeTruthy();
await browser.close();
});
```
SEO Considerations with Lazy Loading
- Ensuring SEO-Friendly Practices: Address SEO considerations when implementing lazy loading in React. Ensure that search engines can crawl and index dynamically loaded content by utilizing server-side rendering or other SEO-friendly techniques.
- Metadata and Server-Side Rendering: Leverage server-side rendering to generate metadata and essential content for lazy-loaded components. This ensures that search engine crawlers receive complete information about each component, contributing to improved SEO.
Conclusion
Lazy loading in React is a key optimization technique that can significantly enhance the performance and user experience of your applications. By understanding the principles of lazy loading, implementing best practices, and leveraging React’s built-in features, you can strike a balance between loading efficiency and user interactivity. Embrace lazy loading to create faster, more responsive React applications that delight users with seamless navigation and improved performance.
What is Redux, and how does it integrate with React
Explain the concept of higher-order components (HOCs) in React
What is the purpose of middleware in Redux
How does React handle forms and form validation
What are the best practices for managing state in a React application
How does React handle server-side rendering (SSR)
How do I create a responsive layout with Tailwind CSS
How do I vertically center an element in Tailwind CSS
How do I customize the spacing like padding and margin between elements in Tailwind CSS