What is the purpose of the getStaticPaths function in dynamic routes
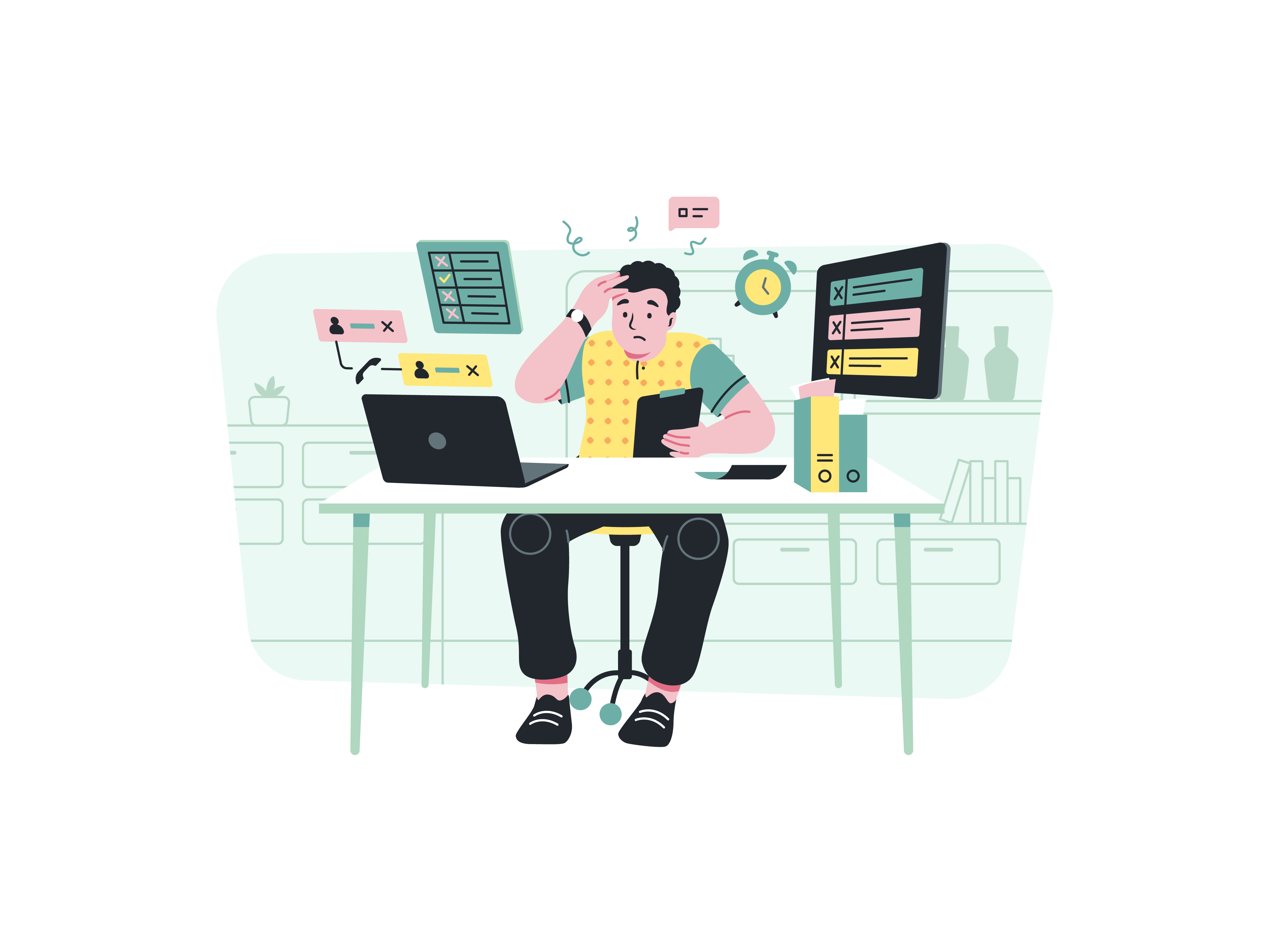
Dynamic routes have become a cornerstone in modern web development, enabling developers to create dynamic, data-driven pages with ease. One crucial aspect of dynamic routes in frameworks like Next.js is the getStaticPaths
function. This function plays a pivotal role in pre-rendering pages and optimizing performance. In this article, we will delve into the purpose of the getStaticPaths
function and explore how it contributes to the overall efficiency of dynamic routes.
Dynamic Routes in Next.js
Before we dive into the getStaticPaths
function, let’s understand the basics of dynamic routes in Next.js. In a traditional static site, each page corresponds to a specific file, making it easy to generate and serve. However, in dynamic routes, the content of a page depends on dynamic parameters.
For instance, consider a blog website where each blog post has a unique identifier. Instead of creating a separate file for each post, you can use a dynamic route to handle this scenario. The URL might look like /posts/[id]
, where [id]
is a placeholder for the dynamic parameter, such as the post identifier.
The Need for getStaticPaths
While dynamic routes offer flexibility, they present challenges when it comes to pre-rendering. Pre-rendering involves generating static HTML files for each page at build time, providing faster load times and improved search engine optimization. However, with dynamic routes, it’s impossible to know all the possible values of the dynamic parameters during the build process.
This is where the getStaticPaths
function comes into play. It allows developers to specify which paths Next.js should pre-render, providing a solution to the challenge posed by dynamic routes.
Defining getStaticPaths
In Next.js, the getStaticPaths
function is part of the page component. This function is responsible for returning an object with two properties: paths
and fallback
.
The paths
Property
The paths
property is an array of objects, where each object represents a set of dynamic parameters for a page. These parameters will be used to generate the static HTML files during the build process. For example:
export async function getStaticPaths() {
return {
paths: [
{ params: { id: '1' } },
{ params: { id: '2' } },
// more params as needed
],
fallback: false,
};
}
In this example, getStaticPaths
specifies an array of paths, each with a different value for the dynamic parameter id
. These paths represent the pages that Next.js will pre-render during the build process.
The fallback
Property
The fallback
property in the object returned by getStaticPaths
determines what happens when a user requests a page that hasn’t been pre-rendered. It can take three values: false
, true
, or 'blocking'
.
false
: Returns a 404 page if the requested page is not pre-rendered.true
: Generates the requested page on-the-fly at the first request. Subsequent requests use the pre-rendered page.'blocking'
: Similar totrue
, but the server waits until the page is generated before responding to the request.
Use Cases for getStaticPaths
Understanding when and how to use getStaticPaths
is crucial for optimizing your Next.js application. Here are some common use cases:
1. Blogging Platforms
In a blogging platform, each blog post might be a dynamic page. The getStaticPaths
function can be used to pre-render the paths for all blog posts during the build process. This ensures fast loading times and better SEO for individual blog posts.
export async function getStaticPaths() {
// Fetch blog post IDs from a database or API
const posts = await fetchPosts();
// Generate paths for each blog post
const paths = posts.map(post => ({ params: { id: post.id.toString() } }));
return { paths, fallback: false };
}
2. E-commerce Websites
For e-commerce websites with product pages, dynamic routes can be employed to handle different products. Using getStaticPaths
, you can pre-render the pages for specific products, ensuring a seamless and performant shopping experience.
export async function getStaticPaths() {
// Fetch product IDs from a database or API
const products = await fetchProducts();
// Generate paths for each product
const paths = products.map(product => ({ params: { id: product.id.toString() } }));
return { paths, fallback: false };
}
3. Multi-language Websites
For websites with multiple languages, dynamic routes can be utilized to handle language-specific content. By using getStaticPaths
, you can pre-render pages for each language, providing a faster and more tailored experience for users.
export async function getStaticPaths() {
const languages = ['en', 'fr', 'es'];
// Generate paths for each language
const paths = languages.map(lang => ({ params: { lang } }));
return { paths, fallback: false };
}
Conclusion
In the realm of dynamic routes, the getStaticPaths
function in Next.js serves a vital purpose. It enables developers to specify which paths should be pre-rendered during the build process, addressing the challenges posed by dynamic parameters. Whether you’re building a blog, an e-commerce site, or a multi-language platform, understanding and effectively using getStaticPaths
is key to optimizing performance, improving user experience, and enhancing search engine visibility in your Next.js applications.
How does Next.js differ from traditional React applications
How does client-side rendering (CSR) work in Next.js
How does Next.js handle bundling and code splitting
What is incremental static regeneration in Next.js
Can a Next.js application use both server-side rendering and client-side rendering
How does Next.js handle SEO for dynamic pages
How do I create a multi-column layout with Tailwind CSS