How can you implement lazy loading in a Next.js application
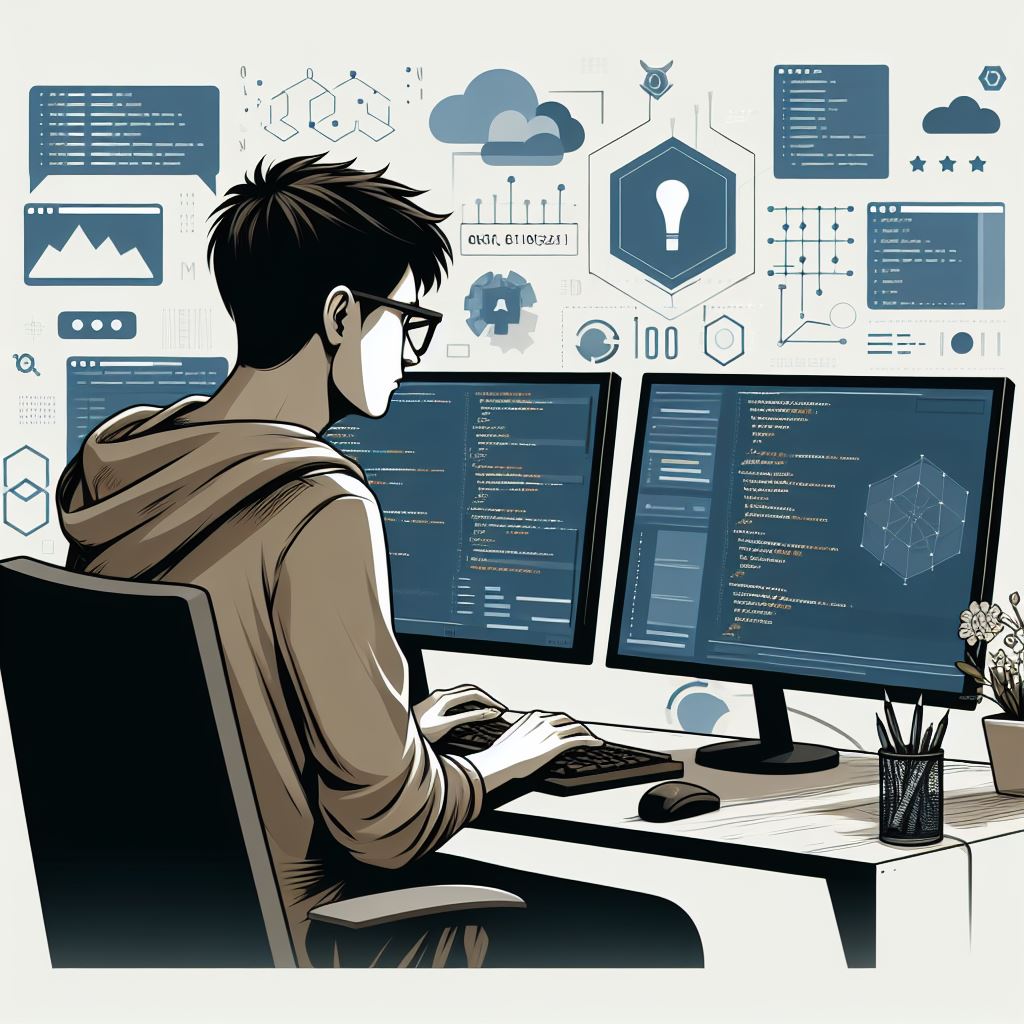
Lazy loading is a crucial optimization technique that allows web developers to defer the loading of certain assets until they are needed. In a Next.js application, where performance is paramount, implementing lazy loading can significantly improve user experience by reducing initial page load times. In this article, we will explore the concept of lazy loading and delve into practical methods to implement it effectively in a Next.js application.
Understanding Lazy Loading
Lazy loading involves delaying the loading of non-essential resources, such as images or JavaScript, until the user actually needs them. This technique is particularly beneficial for web pages with extensive content or resources that might not be immediately visible. By deferring the loading of these assets, we can optimize the initial page load time, resulting in faster and more efficient web applications.
In the context of a Next.js application, lazy loading can be applied to various elements, including images, components, and even entire pages.
Lazy Loading Images
One of the most common use cases for lazy loading in a web application is with images. In a Next.js application, you can implement lazy loading for images using the next/image
component.
// Example of lazy loading images in Next.js
import Image from 'next/image';
const LazyImage = () => (
<div>
{/* Placeholder image that is loaded immediately */}
<Image
src="/placeholder.jpg"
alt="Placeholder Image"
width={300}
height={200}
/>
{/* Actual image loaded lazily when it comes into view */}
<Image
src="/real-image.jpg"
alt="Real Image"
width={300}
height={200}
loading="lazy"
/>
</div>
);
export default LazyImage;
In this example, the loading="lazy"
attribute is added to the Image
component, indicating that the browser should load the image lazily. The placeholder image is loaded immediately, providing a seamless user experience while the actual image is loaded in the background.
Lazy Loading Components
Lazy loading is not limited to images; you can also apply it to React components to optimize the loading of certain parts of your application. React provides a built-in method for lazy loading components using the React.lazy
function and the Suspense
component.
// Example of lazy loading components in Next.js
import { lazy, Suspense } from 'react';
const LazyComponent = lazy(() => import('./LazyComponent'));
const LazyLoadingExample = () => (
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
);
export default LazyLoadingExample;
In this example, the React.lazy
function is used to load the LazyComponent
dynamically. The Suspense
component is employed to show a fallback UI while the component is being loaded.
Lazy Loading Pages
Next.js allows for the lazy loading of entire pages, ensuring that resources associated with specific routes are only loaded when the user navigates to those routes. This is achieved using the next/dynamic
function.
// Example of lazy loading pages in Next.js
import dynamic from 'next/dynamic';
const LazyPage = dynamic(() => import('../components/LazyPage'));
const Index = () => (
<div>
{/* Content of the initial page */}
<p>Welcome to the home page!</p>
{/* Lazy-loaded page */}
<LazyPage />
</div>
);
export default Index;
In this example, the next/dynamic
function is used to load the LazyPage
component dynamically. This ensures that the resources associated with LazyPage
are only fetched when the user navigates to the page.
Combining Strategies for Optimal Lazy Loading
In a real-world Next.js application, you may find yourself needing a combination of lazy loading strategies to achieve optimal performance. For instance, you might employ lazy loading for images within lazy-loaded components on specific pages. This layered approach allows you to fine-tune the lazy loading behavior based on your application’s structure and requirements.
// Example of combining lazy loading strategies in Next.js
import dynamic from 'next/dynamic';
import Image from 'next/image';
const LazyComponentWithImages = dynamic(() => import('./LazyComponentWithImages'));
const LazyLoadingExample = () => (
<div>
{/* Lazy-loaded component */}
<LazyComponentWithImages />
</div>
);
export default LazyLoadingExample;
In this example, the LazyComponentWithImages
component is lazy-loaded, and within that component, individual images are also loaded lazily using the next/image
component. This layered approach allows for a granular and efficient implementation of lazy loading.
Conclusion
Lazy loading is a potent optimization technique that can significantly enhance the performance of your Next.js applications. By deferring the loading of non-essential resources until they are needed, you can achieve faster initial page load times and create a more responsive user experience. Whether you are dealing with images, components, or entire pages, Next.js provides the tools and flexibility to implement lazy loading seamlessly. As you navigate the landscape of web development, consider incorporating lazy loading into your toolkit to unlock the full potential of your Next.js applications.
How to use Bootstrap’s responsive embed classes for videos
How to create a responsive contact form with Bootstrap
How to use Bootstrap’s utilities for hiding and showing elements
How to implement a sticky footer with a content area that scrolls
How to use Bootstrap’s form control sizing classes
How to create a responsive image carousel with captions
How to use Bootstrap’s responsive utilities for text alignment
How to implement a full-screen background image with Bootstrap
How to use Bootstrap’s list group with badges
How to use Bootstrap’s custom form controls for file inputs
How to implement a fixed sidebar with a scrollable content area