What is the purpose of the useCallback hook in React
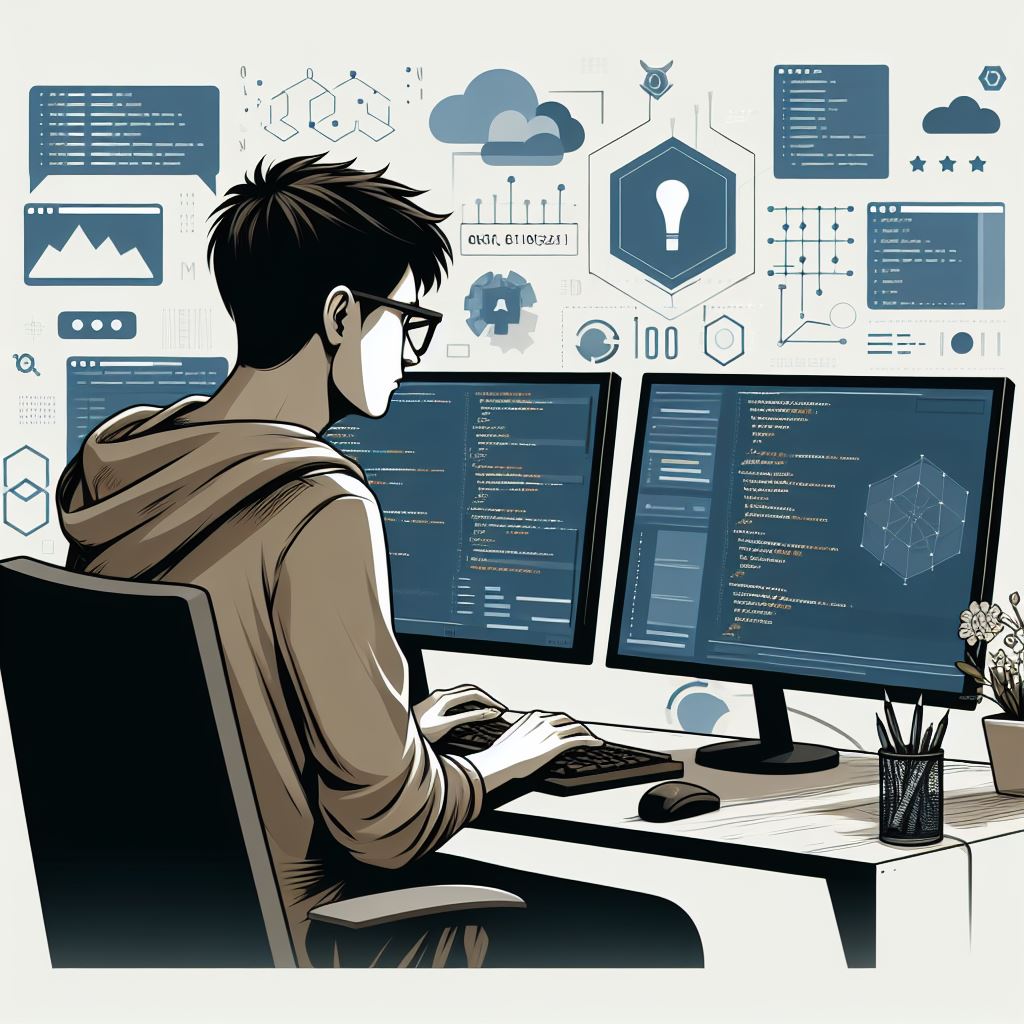
React, known for its powerful and efficient state management, introduces various hooks to optimize functional components. Among these, the useCallback
hook plays a crucial role in enhancing performance by memoizing callback functions. In this article, we’ll delve into the purpose of the useCallback
hook, its benefits, and practical use cases that demonstrate its significance in React development.
-
Understanding the Basics of React Hooks
React Hooks were introduced to allow functional components to manage state and side effects, previously exclusive to class components. These hooks enable developers to encapsulate logic and maintain component state without converting to class components. The
useCallback
hook, in particular, addresses the issue of unnecessary re-rendering caused by the creation of new callback functions on each render cycle. -
The Essence of useCallback
-
When a functional component renders, its internal functions, including callbacks, are recreated. While this behavior is generally not problematic, it can lead to inefficiencies, especially when passing callbacks to child components. The
useCallback
hook aims to optimize this process by memoizing the callback function, ensuring it remains consistent across renders unless its dependencies change. -
Syntax and Usage
The
useCallback
hook takes two arguments: the callback function and an array of dependencies. It returns a memoized version of the callback function that only changes if the dependencies change. This ensures that the same callback instance is provided across renders unless the specified dependencies have changed.const memoizedCallback = useCallback( () => { // Callback logic }, [dependency1, dependency2] );
-
Preventing Unnecessary Renderings
Consider a scenario where a parent component renders a child component and passes a callback function as a prop. Without
useCallback
, the callback is recreated on each render of the parent component, triggering unnecessary re-renders of the child component. By usinguseCallback
, the callback remains constant unless its dependencies change, preventing unnecessary renderings of the child component.// Without useCallback const ParentComponent = () => { const handleClick = () => { // Callback logic }; return <ChildComponent onClick={handleClick} />; };
// With useCallback const ParentComponent = () => { const handleClick = useCallback(() => { // Callback logic }, []); return <ChildComponent onClick={handleClick} />; };
-
Optimizing Performance in Dependency-Driven Scenarios
In scenarios where the callback’s behavior depends on specific state or props, specifying those dependencies in the
useCallback
dependency array ensures that the callback is updated only when the relevant data changes. This optimization is particularly beneficial in scenarios involving heavy computations or expensive calculations.const ParentComponent = ({ data }) => { const handleClick = useCallback(() => { // Callback logic using data }, [data]); return <ChildComponent onClick={handleClick} />; };
-
Use Cases and Practical Examples
- Memoizing Event Handlers:
When passing event handlers to child components, memoizing them with
useCallback
prevents unnecessary child component renders. - Optimizing useEffect Dependencies:
In scenarios where an effect depends on specific props or state, using
useCallback
helps optimize the effect’s dependency array. - Preventing Unnecessary Renders in List Iterations:
When mapping over an array to render a list, using
useCallback
for callback functions ensures that the same function instance is used for each item. - Enhancing Performance in Context Providers:
When using context providers,
useCallback
can be employed to memoize functions provided through the context value.
- Memoizing Event Handlers:
When passing event handlers to child components, memoizing them with
-
Caveats and Considerations
- Balancing Optimization and Complexity:
While
useCallback
offers performance benefits, it’s essential to balance optimization with code simplicity. OverusinguseCallback
can lead to unnecessary complexity. - Avoiding Callbacks in Dependencies Array: Care should be taken not to include callback functions in the dependency array if they are defined within the component, as it may lead to unintended behavior.
- Understanding Use Cases:
Consider using
useCallback
primarily in scenarios where callback functions are passed as props or used in dependencies to prevent unnecessary re-renders.
- Balancing Optimization and Complexity:
While
-
Future Developments and Best Practices
As React evolves, best practices around hooks may change, and additional optimizations may be introduced. Keeping an eye on the official React documentation and staying informed about the latest developments ensures that developers adhere to best practices.
Conclusion
In the realm of React development, the useCallback
hook proves to be a valuable tool for optimizing performance by memoizing callback functions. By preventing unnecessary re-renders and ensuring consistency in callback instances, React developers can create more efficient and responsive applications. Understanding the scenarios where useCallback
shines and incorporating it judiciously into the development workflow empowers developers to strike a balance between performance optimization and code simplicity in their React applications.
How do you manage state in a Redux store
How does React handle memoization
How do you handle forms in React with controlled and uncontrolled components
What is the purpose of the useMemo hook in React
What are the key features of React
How does React handle component-based architecture